In-depth exploration of efficient methods of data processing in Golang
Golang (also known as Go language), as an emerging concurrent programming language, is loved by programmers for its simplicity, efficiency and ease of use. In daily development, data processing is one of the indispensable and important links. This article will delve into efficient methods of data processing in Golang, and use specific code examples to show how to use Golang's features to process data.
1. Use map for data processing
In Golang, map is a very flexible and efficient data structure, especially suitable for fast data query and processing. Here is a simple example that shows how to use map to count the number of occurrences of each word in a piece of text:
package main import ( "fmt" "strings" ) func main() { text := "Go is a concurrent and efficient programming language Go Go" words := strings.Fields(text) wordCount := make(map[string]int) for _, word := range words { wordCount[word]++ } for word, count := range wordCount { fmt.Printf("%s: %d ", word, count) } }
In the above example, we first use the strings.Fields
function to The text is split into words, and a map is constructed with the word as the key, then the word slices are traversed, the number of occurrences of each word is counted, and each word and the number of occurrences are printed out.
2. Use goroutine for concurrent processing
Golang's goroutine is a lightweight thread that can handle concurrent tasks more efficiently. The following is an example that shows how to use goroutine to process multiple tasks concurrently:
package main import ( "fmt" "time" ) func processTask(task string) { // 模拟任务处理过程 time.Sleep(1 * time.Second) fmt.Println("Processed task:", task) } func main() { tasks := []string{"task1", "task2", "task3", "task4", "task5"} for _, task := range tasks { go processTask(task) } // 等待所有任务完成 time.Sleep(2 * time.Second) }
In the above example, we define a processTask
function to simulate the task processing process, and then process it concurrently through goroutine Multiple tasks. By using goroutine, we can handle concurrent tasks more efficiently and improve program performance.
3. Use channel for data communication
In concurrent programming, data sharing and communication is an important issue. Golang provides channels as a mechanism for communication between multiple goroutines. The following is an example showing how to use channels to implement data communication between goroutines:
package main import "fmt" func produce(ch chan int) { for i := 1; i <= 5; i++ { ch <- i // 将数据发送到channel } close(ch) // 关闭channel } func consume(ch chan int) { for num := range ch { fmt.Println("Consumed:", num) } } func main() { ch := make(chan int) go produce(ch) go consume(ch) // 等待goroutine执行完成 var input string fmt.Scanln(&input) }
In the above example, we define a produce
function to send data to the channel, define A consume
function is used to receive data from the channel. By using channels, we can realize data communication between goroutines and ensure the safe transmission and sharing of data.
Summary, this article deeply explores efficient methods of Golang data processing through specific code examples, covering the use of map for data processing, the use of goroutine for concurrent processing, and the use of channels for data communication. By making full use of Golang's features, the efficiency and performance of data processing can be improved, providing developers with a better experience.
The above is the detailed content of In-depth exploration of efficient methods of data processing in Golang. For more information, please follow other related articles on the PHP Chinese website!
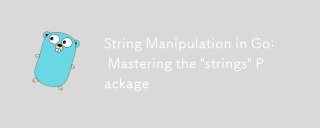
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
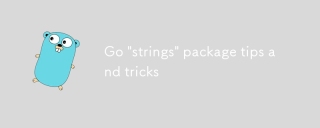
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
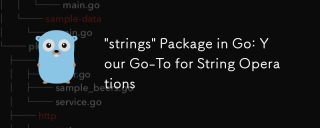
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
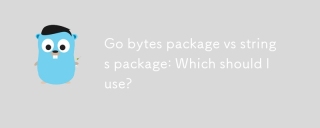
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
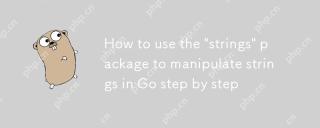
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
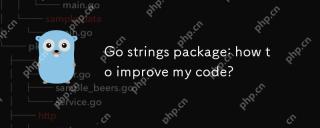
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
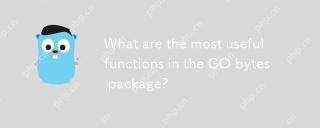
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
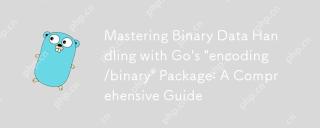
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
