The Magic Recipe of Python Syntax: Master the Basics of Code
Variables and data types
Variables are used to store data, and their names must follow the naming rules of python. Python supports multiple data types, including numbers, strings, lists, and tuples. When declaring a variable, associate it with a value using the assignment operator (=).
# 声明一个整数变量 age = 25 # 声明一个字符串变量 name = "John Smith"
Process Control
Python uses conditional statements (if-else) and loop statements (for, while) to control program flow. Conditional statements execute different blocks of code based on a condition, while loop statements repeatedly execute a specific block of code until an exit condition is met.
# if-else 语句 if age >= 18: print("成年人") else: print("未成年人") # for 循环 for i in range(5): print(i)
Functions and modules
Function encapsulates a set of reusable code that can be called using the function name. Modules organize related functions and data together and can be imported and reused.
# 定义一个函数 def add_numbers(a, b): return a + b # 导入一个模块 import math
data structure
Python provides powerful data structures to organize and manipulate data, including lists, tuples, dictionaries and sets.
- List: An ordered, mutable collection of data whose elements can be accessed and modified using indexes .
- Tuple: An ordered immutable collection of data that cannot be modified once created.
- Dictionary: Unordered variable data collection, using key-value pairs to store data.
- Set: Unordered collection of unique elements, used to find and delete duplicate elements.
Objects and Classes
Python is an object-orientedprogramming language, where objects encapsulate data and methods. A class is a blueprint for creating new objects, and it defines the object's behavior and properties.
# 定义一个类 class Person: def __init__(self, name, age): self.name = name self.age = age def greet(): print(f"Hello, my name is {self.name} and I am {self.age} years old.")
Error handling
try-except statements are used to handle errors in code. When an error occurs, the program executes the code in the except block and continues running.
try: # 可能引发错误的代码 except Exception as e: # 错误处理代码
Summarize
Mastering the basics of Python syntax is a solid first step in the programming journey. From variables and data types to flow control, data structures, objects, and classes, Python provides a rich set of tools for developing powerful and flexible applications. Through continuous practice and exploration, you will become an expert in Python programming.
The above is the detailed content of The Magic Recipe of Python Syntax: Master the Basics of Code. For more information, please follow other related articles on the PHP Chinese website!
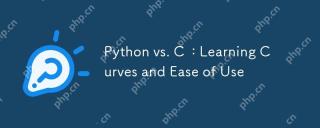
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
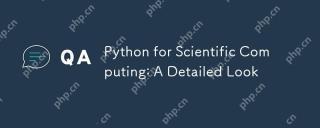
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
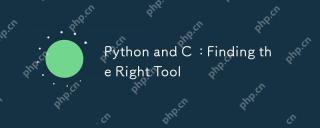
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
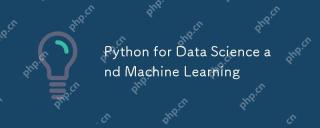
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
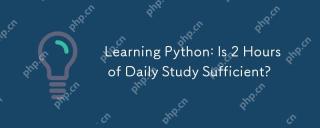
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
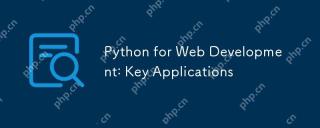
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
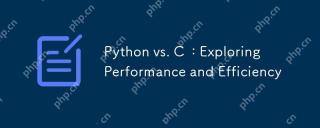
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
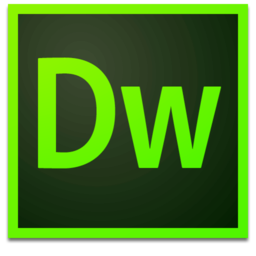
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
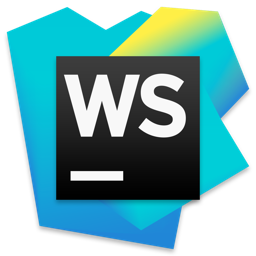
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.