The implementation skills of the greatest common divisor algorithm in C language require specific code examples
The Greatest Common Divisor (GCD) refers to two or more The largest divisor shared by all integers. In computer programming, finding the greatest common denominator is a common problem, especially in programming tasks in fields such as numerical analysis and cryptography. The following will introduce several of the most commonly used algorithms for finding the greatest common divisor in C language, as well as implementation techniques and specific code examples.
- Euclidean division method (Euclidean algorithm)
Euclidean division method is a common method for finding the greatest common divisor, also known as Euclidean algorithm. The basic idea is to divide a larger number by a smaller number, then use the remainder as the new divisor, then use this remainder as the dividend, and the original divisor as the divisor. This cycle continues until the remainder is 0, and the divisor at this time is the greatest common denominator. number.
The following is an example of C language code that uses euclidean division to find the greatest common divisor:
#include <stdio.h> // 使用辗转相除法求最大公约数 int gcd(int a, int b) { while (b != 0) { int temp = a; a = b; b = temp % b; } return a; } int main() { int a, b; printf("请输入两个整数:"); scanf("%d%d", &a, &b); int result = gcd(a, b); printf("最大公约数为:%d ", result); return 0; }
Through the above code, you can input two integers, and the program will output their greatest common divisor. number.
- Additional Subtraction Method
Additional Subtraction Method is another method to find the greatest common divisor. It approaches the greatest common divisor by continuously subtracting the difference between two numbers. The specific steps are: if a and b are two numbers, if a > b, then a = a - b; if a
The following is an example of C language code that uses the subtraction method to find the greatest common divisor:
#include <stdio.h> // 使用更相减损法求最大公约数 int gcd(int a, int b) { while (a != b) { if (a > b) { a = a - b; } else { b = b - a; } } return a; } int main() { int a, b; printf("请输入两个整数:"); scanf("%d%d", &a, &b); int result = gcd(a, b); printf("最大公约数为:%d ", result); return 0; }
Compared with the euclidean division method, the operation process of the subtraction method may be more expensive. time, so it is rarely used in practical applications.
- Other methods
In addition to the euclidean division method and the phase subtraction method, there are other methods that can also be used to solve the greatest common divisor, such as the prime factorization method, the continuous integer detection method, etc. . According to different application scenarios and requirements, choosing the appropriate method can improve computing efficiency.
In actual programming, there are some skills that need to be paid attention to:
- When the input number is very large, in order to improve calculation efficiency, you can use long integer ( long) to store data.
- Check the validity of the input to ensure that the input is a positive integer to avoid invalid calculations or numerical overflow problems.
- Using functions for code modular design can improve the readability and maintainability of the code.
Summary:
Solving the greatest common divisor is a common programming task. In C language, the euclidean and subtraction methods are the most commonly used solving methods. By flexibly using these algorithms, combined with reasonable code implementation techniques, the efficiency and stability of the program can be improved, making it better adaptable to various computing needs.
The above is the detailed content of Tip: Implementing the Greatest Common Divisor Algorithm in C. For more information, please follow other related articles on the PHP Chinese website!
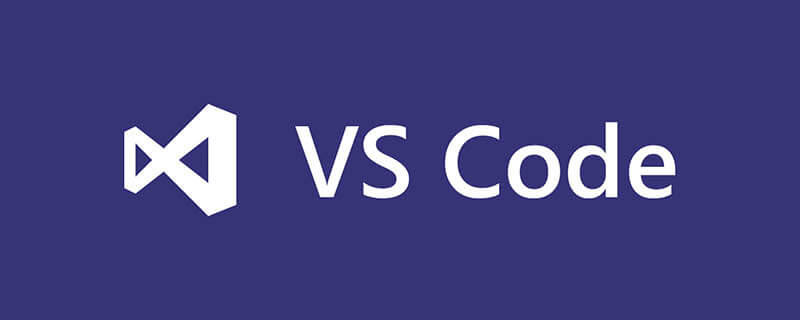
VScode中怎么配置C语言环境?下面本篇文章给大家介绍一下VScode配置C语言环境的方法(超详细),希望对大家有所帮助!
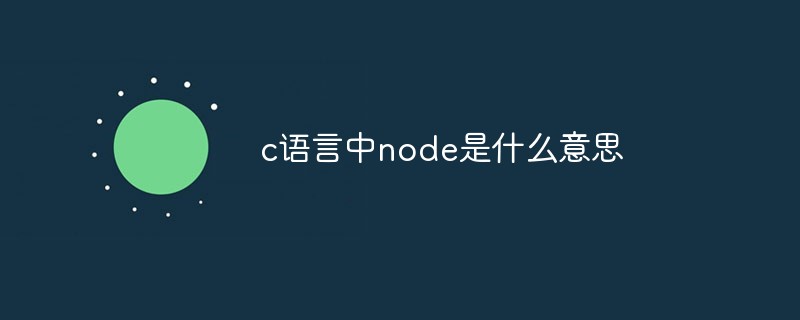
在C语言中,node是用于定义链表结点的名称,通常在数据结构中用作结点的类型名,语法为“struct Node{...};”;结构和类在定义出名称以后,直接用该名称就可以定义对象,C语言中还存在“Node * a”和“Node* &a”。

c语言将数字转换成字符串的方法:1、ascii码操作,在原数字的基础上加“0x30”,语法“数字+0x30”,会存储数字对应的字符ascii码;2、使用itoa(),可以把整型数转换成字符串,语法“itoa(number1,string,数字);”;3、使用sprintf(),可以能够根据指定的需求,格式化内容,存储至指针指向的字符串。
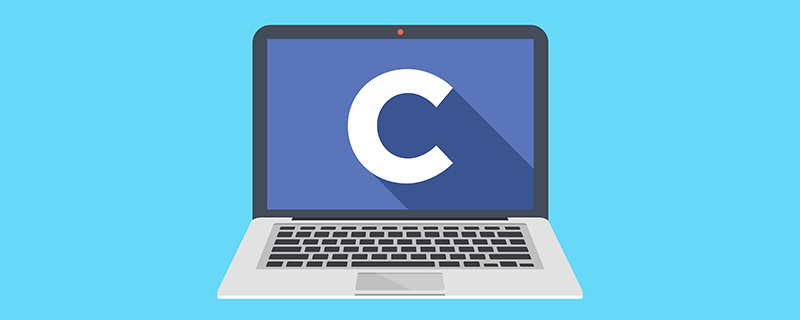
在c语言中,没有开根号运算符,开根号使用的是内置函数“sqrt()”,使用语法“sqrt(数值x)”;例如“sqrt(4)”,就是对4进行平方根运算,结果为2。sqrt()是c语言内置的开根号运算函数,其运算结果是函数变量的算术平方根;该函数既不能运算负数值,也不能输出虚数结果。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
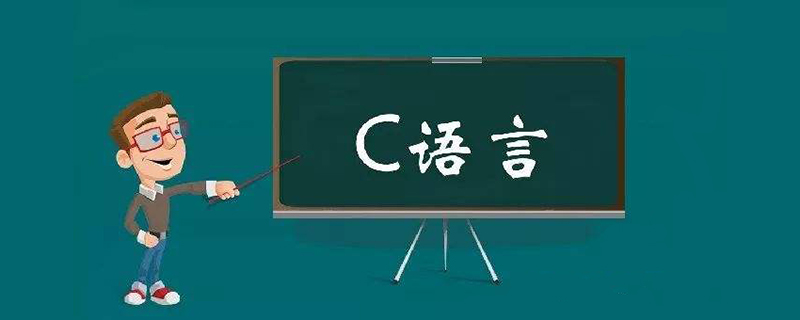
c语言合法标识符的要求是:1、标识符只能由字母(A~Z, a~z)、数字(0~9)和下划线(_)组成;2、第一个字符必须是字母或下划线,不能是数字;3、标识符中的大小写字母是有区别的,代表不同含义;4、标识符不能是关键字。
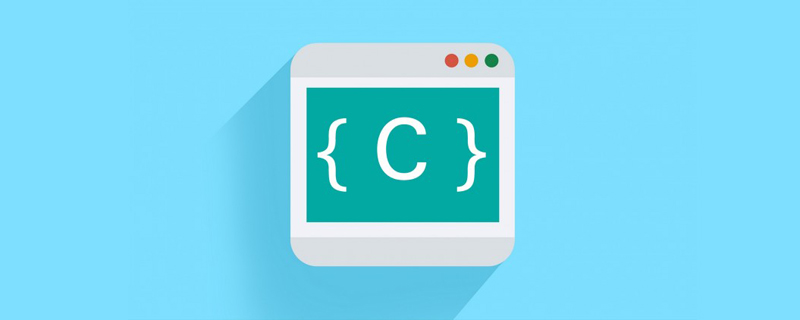
c语言编译后生成“.OBJ”的二进制文件(目标文件)。在C语言中,源程序(.c文件)经过编译程序编译之后,会生成一个后缀为“.OBJ”的二进制文件(称为目标文件);最后还要由称为“连接程序”(Link)的软件,把此“.OBJ”文件与c语言提供的各种库函数连接在一起,生成一个后缀“.EXE”的可执行文件。
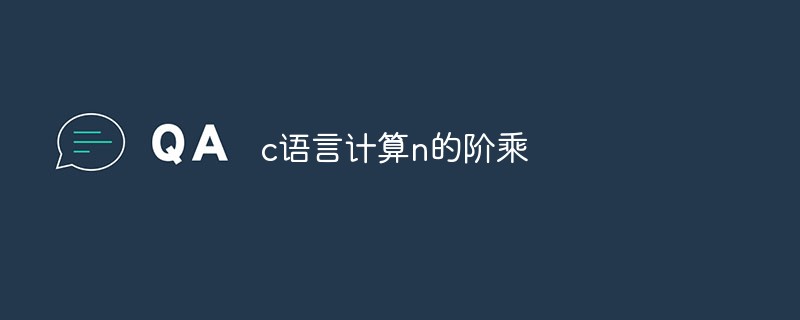
c语言计算n的阶乘的方法:1、通过for循环计算阶乘,代码如“for (i = 1; i <= n; i++){fact *= i;}”;2、通过while循环计算阶乘,代码如“while (i <= n){fact *= i;i++;}”;3、通过递归方式计算阶乘,代码如“ int Fact(int n){int res = n;if (n > 1)res...”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
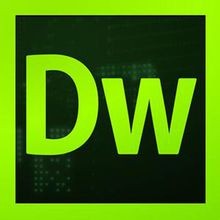
Dreamweaver CS6
Visual web development tools
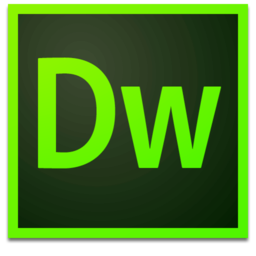
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
