


php editor Strawberry brings you a wonderful article: "Practical Analysis of Java Iterator and Iterable: Cleverly Handling Various Data Structures". This article will delve into the use of Iterator and Iterable interfaces in Java to help readers better handle various data structures and improve code efficiency and readability. Let’s learn these practical skills together and improve your Java programming skills!
Array is one of the simplest data structures. It can store a series of elements, and the types of the elements must be the same. To iterate over an array, you can use the following code:
int[] numbers = {1, 2, 3, 4, 5}; for (int number : numbers) { System.out.println(number); }
This code will output all elements in the array.
2. List
List is another commonly used data structure, which is similar to an array but more flexible. Lists can store different types of data and can be resized dynamically. To iterate over a list you can use the following code:
List<String> names = new ArrayList<>(); names.add("John"); names.add("Mary"); names.add("Bob"); for (String name : names) { System.out.println(name); }
This code will output all elements in the list.
3. Collection
Set is another important data structure that can store a set of unique elements. To iterate over a collection, you can use the following code:
Set<Integer> numbers = new HashSet<>(); numbers.add(1); numbers.add(2); numbers.add(3); for (int number : numbers) { System.out.println(number); }
This code will output all elements in the collection.
4. Mapping
A map is a data structure that maps keys to values. To iterate over the map, you can use the following code:
Map<String, Integer> ages = new HashMap<>(); ages.put("John", 25); ages.put("Mary", 30); ages.put("Bob", 35); for (Map.Entry<String, Integer> entry : ages.entrySet()) { System.out.println(entry.geTKEy() + " = " + entry.getValue()); }
This code will output all key-value pairs in the map.
5. Custom data structure
You can also use Iterator and Iterable to iterate over custom data structures. For example, you can create your own LinkedList class and implement the Iterator interface. This way you can use the above code to iterate over all elements in the linked list.
in conclusion
Java Iterator and Iterable are two powerful tools that can help you iterate over various data structures easily. By understanding how to use them, you can process data more easily and write more robust code.
The above is the detailed content of Java Iterator and Iterable practical analysis: cleverly handle various data structures. For more information, please follow other related articles on the PHP Chinese website!
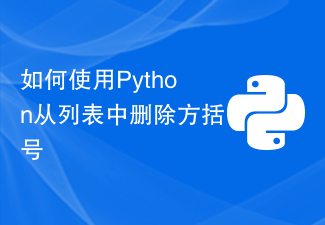
Python是一款非常有用的软件,可以根据需要用于许多不同的目的。Python可以用于Web开发、数据科学、机器学习等许多其他需要自动化处理的领域。它具有许多不同的功能,可以帮助我们执行这些任务。Python列表是Python的一个非常有用的功能之一。顾名思义,列表包含您希望存储的所有数据。它基本上是一组不同类型的信息。删除方括号的不同方法许多时候,用户会遇到列表项显示在方括号中的情况。在本文中,我们将详细介绍如何去掉这些括号,以便更好地查看您的列表。字符串和替换函数删除括号的最简单方法之一是在
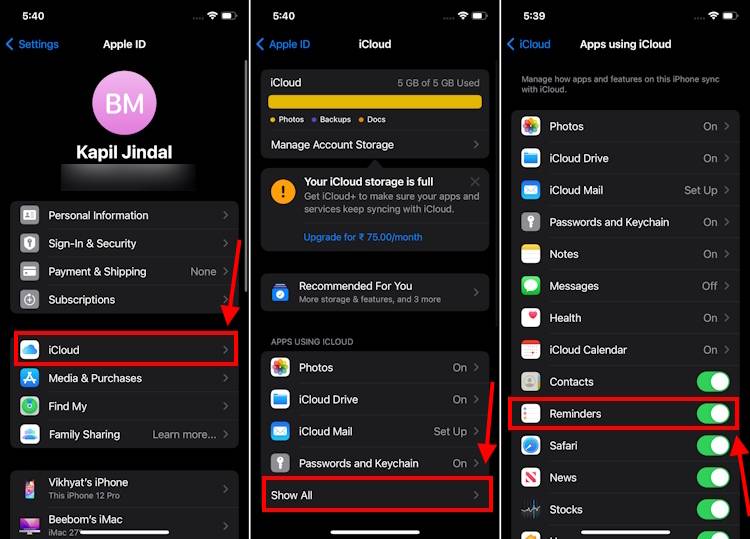
如何在iOS17中的iPhone上制作GroceryList在“提醒事项”应用中创建GroceryList非常简单。你只需添加一个列表,然后用你的项目填充它。该应用程序会自动将您的商品分类,您甚至可以与您的伴侣或扁平伙伴合作,列出您需要从商店购买的东西。以下是执行此操作的完整步骤:步骤1:打开iCloud提醒事项听起来很奇怪,苹果表示您需要启用来自iCloud的提醒才能在iOS17上创建GroceryList。以下是它的步骤:前往iPhone上的“设置”应用,然后点击[您的姓名]。接下来,选择i
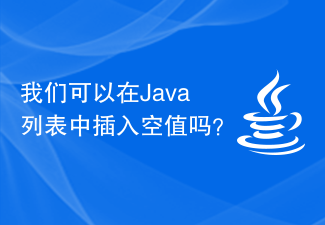
SolutionYes,Wecaninsertnullvaluestoalisteasilyusingitsadd()method.IncaseofListimplementationdoesnotsupportnullthenitwillthrowNullPointerException.Syntaxbooleanadd(Ee)将指定的元素追加到此列表的末尾。类型参数E −元素的运行时类型。参数e −要追加到此列表的元
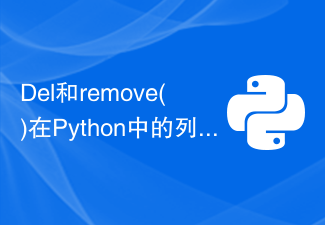
在讨论差异之前,让我们先了解一下Python列表中的Del和Remove()是什么。Python列表中的Del关键字Python中的del关键字用于从List中删除一个或多个元素。我们还可以删除所有元素,即删除整个列表。示例使用del关键字从Python列表中删除元素#CreateaListmyList=["Toyota","Benz","Audi","Bentley"]print("List="
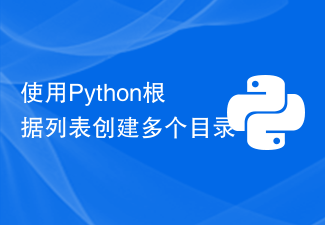
Python凭借其简单性和多功能性,已成为各种应用程序中最流行的编程语言之一。无论您是经验丰富的开发人员还是刚刚开始编码之旅,Python都提供了广泛的功能和库,使复杂的任务变得易于管理。在本文中,我们将探讨一个实际场景,Python可以通过自动执行基于列表创建多个目录的过程来帮助我们。通过利用Python内置模块和技术的强大功能,我们可以有效地处理此任务,而无需手动干预。在本教程中,我们将深入研究创建多个目录的问题,并为您提供使用Python解决该问题的不同方法。在本文结束时,我们的目标是为您
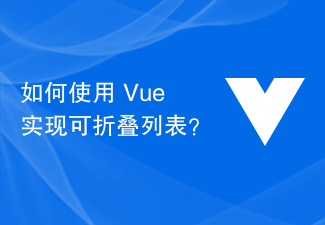
Vue是一款流行的JavaScript库,广泛应用于Web开发领域。在Vue中,我们可以很方便地实现各种组件和交互效果。其中,可折叠列表是一个比较实用的组件,它可以将列表数据分组,提高数据展示的可读性,同时又能够在需要展开具体内容时进行展开,方便用户查看详细信息。本文就将介绍如何使用Vue实现可折叠列表。准备工作在使用Vue实现可折叠列
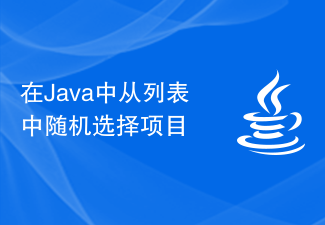
List是JavaCollection接口的子接口。它是一种线性结构,按照顺序存储和访问每个元素。为了使用list的特性,我们使用实现了list接口的ArrayList和LinkedList类。在本文中,我们将创建一个ArrayList,并尝试随机选择该列表中的项目。在Java中随机选择列表中的项目的程序随机类别我们创建此类的对象来生成伪随机数。我们将自定义该对象并应用我们自己的逻辑从列表中选择任何随机项目。语法RandomnameOfObject=newRandom();Example1的翻译
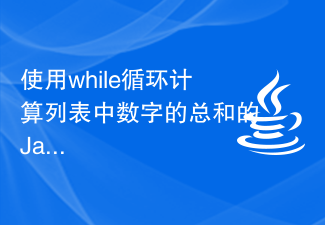
介绍使用while循环计算列表中数字总和的Java程序是一个简单的程序,它获取整数列表并使用while循环结构计算它们的总和。在此程序中,创建了一个整数ArrayList,并将一些数字添加到该列表中。然后,程序使用while循环迭代列表中的每个元素,将每个元素添加到变量“sum”中,该变量跟踪数字的运行总和。循环完成后,“sum”的最终值将打印到控制台,它是列表中所有数字的总和。该程序演示了在编程中处理数据集合的常用技术,即使用循环迭代集合中的每个元素并对每个元素执行一些计算或转换。该计划还重点


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
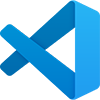
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
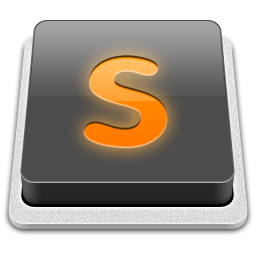
SublimeText3 Mac version
God-level code editing software (SublimeText3)
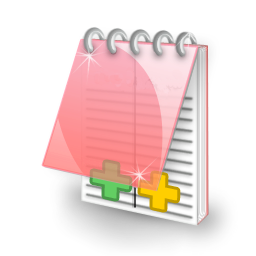
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
