Java Iterator vs. Iterable: Unlocking the Secret to Effective Programming. Iterator and Iterable in Java are key interfaces commonly used in programming. They can help us achieve efficient data traversal and operations. Flexible use of Iterator and Iterable in Java programming can make our code more concise and efficient, improving development efficiency and code quality. This article will delve into the usage tips and precautions of Iterator and Iterable to help readers better understand and apply these two interfaces, thereby improving programming efficiency and quality.
Iterator is an interface in the Java collection framework used to traverse collection elements. It provides two basic methods, hasNext() and next(), which are used to check whether there are more elements in the collection and to get the next element respectively. The Iterable interface is the parent interface of Iterator. It only declares the iterator() method, which is used to return a new Iterator instance.
Iterator and Iterable are very simple to use, just use Java’s foreach statement. The foreach statement automatically creates an Iterator instance and iterates through all elements in the collection without having to manually call the hasNext() and next() methods. For example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); for (int number : numbers) { System.out.println(number); }
Output:
List<String> names = Arrays.asList("John", "Mary", "Bob"); Iterator<String> iterator = names.iterator(); while (iterator.hasNext()) { String name = iterator.next(); System.out.println(name); }
- Use Iterable to create your own iterable object:
class MyIterable implements Iterable<Integer> { private List<Integer> numbers; public MyIterable(List<Integer> numbers) { this.numbers = numbers; } @Override public Iterator<Integer> iterator() { return new MyIterator(numbers); } } class MyIterator implements Iterator<Integer> { private List<Integer> numbers; private int index = 0; public MyIterator(List<Integer> numbers) { this.numbers = numbers; } @Override public boolean hasNext() { return index < numbers.size(); } @Override public Integer next() { return numbers.get(index++); } } public class Main { public static void main(String[] args) { MyIterable iterable = new MyIterable(Arrays.asList(1, 2, 3, 4, 5)); for (int number : iterable) { System.out.println(number); } } }
Output:
1 2 3 4 5
Iterator and Iterable are two very important interfaces in the Java collection framework. They provide efficient access and traversal of collection elements. By understanding the concepts and usage of Iterator and Iterable, you can write efficient and elegant Java code.
The above is the detailed content of Java Iterator vs. Iterable: Unlocking the Secret to Efficient Programming. For more information, please follow other related articles on the PHP Chinese website!
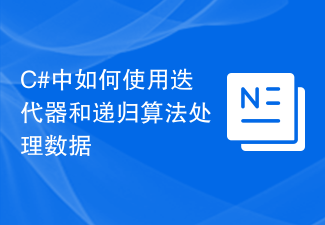
C#中如何使用迭代器和递归算法处理数据,需要具体代码示例在C#中,迭代器和递归算法是两种常用的数据处理方法。迭代器可以帮助我们遍历集合中的元素,而递归算法则能够有效地处理复杂的问题。本文将详细介绍如何使用迭代器和递归算法来处理数据,并提供具体的代码示例。使用迭代器处理数据在C#中,我们可以使用迭代器来遍历集合中的元素,而无需事先知道集合的大小。通过迭代器,我
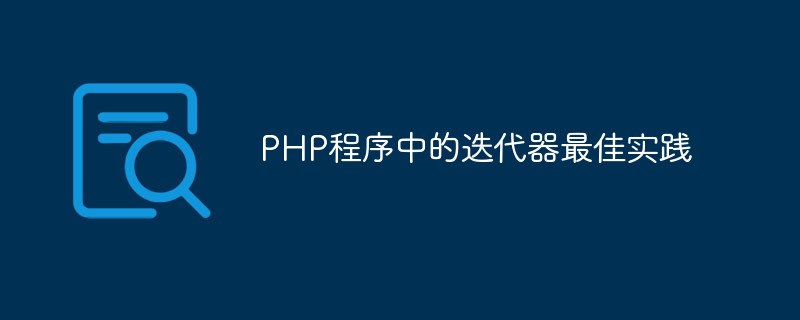
PHP程序中的迭代器最佳实践迭代器在PHP编程中是一种非常常用的设计模式。通过实现迭代器接口,我们可以遍历一个集合对象中的元素,而且还可以轻松的实现自己的迭代器对象。在PHP中,迭代器模式可以帮助我们更有效地操作数组、列表等集合对象。在本文中,我们将介绍PHP程序中迭代器的最佳实践,希望能帮助同样在迭代器应用方面工作的PHP开发人员。一、使用标准迭代器接口P
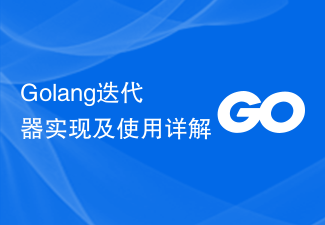
Golang是一个快速、高效的静态编译型语言,其简洁的语法和强大的性能让它在软件开发领域备受青睐。在Golang中,迭代器(Iterator)是一种常用的设计模式,用于遍历集合中的元素而无需暴露集合的内部结构。本文将详细介绍如何在Golang中实现和使用迭代器,通过具体的代码示例帮助读者更好地理解。1.迭代器的定义在Golang中,迭代器通常由一个接口和实
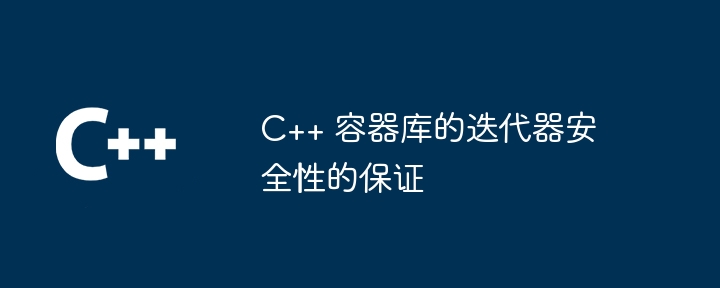
C++容器库提供以下机制确保迭代器的安全性:1.容器不变性保证;2.复制迭代器;3.范围for循环;4.Const迭代器;5.异常安全。
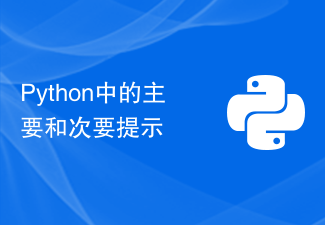
简介主要和次要提示,要求用户输入命令并与解释器进行通信,使得这种交互模式成为可能。主要提示通常由>>>表示,表示Python已准备好接收输入并执行相应的代码。了解这些提示的作用和功能对于发挥Python的交互式编程能力至关重要。在本文中,我们将讨论Python中的主要和次要提示符,强调它们的重要性以及它们如何增强交互式编程体验。我们将研究它们的功能、格式选择以及在快速代码创建、实验和测试方面的优势。开发人员可以通过理解主要和次要提示符来使用Python的交互模式,从而改善他们的
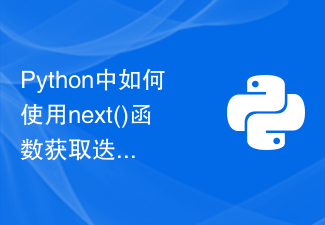
Python中如何使用next()函数获取迭代器的下一个元素迭代器是Python中很常用的一个概念,它允许我们按照特定的顺序遍历一个数据集合。在迭代过程中,我们经常需要获取迭代器的下一个元素,这时就可以使用next()函数来实现。在Python中,我们可以使用iter()函数将一个可迭代对象转换成一个迭代器。例如,如果我们有一个列表,可以将其转换成迭代器后进
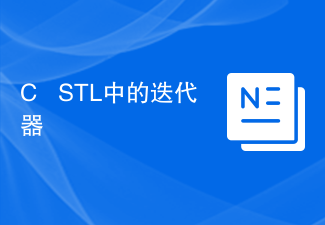
C++STL(StandardTemplateLibrary)是C++程序语言的标准库之一,它包含了一系列的标准数据结构和算法。在STL中,迭代器(iterator)是一种非常重要的工具,用于在STL的容器中进行遍历和访问。迭代器是一个类似于指针的对象,它可以指向容器(例如vector、list、set、map等)中的某个元素,并可以在容器中进行移动、

概念差异:Iterator:Iterator是一个接口,代表一个从集合中获取值的迭代器。它提供了MoveNext()、Current()和Reset()等方法,允许你遍历集合中的元素,并对当前元素进行操作。Iterable:Iterable也是一个接口,代表一个可迭代的对象。它提供了Iterator()方法,用于返回一个Iterator对象,以便于遍历集合中的元素。使用方式:Iterator:要使用Iterator,需要先获得一个Iterator对象,然后调用MoveNext()方法来移动到下一


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
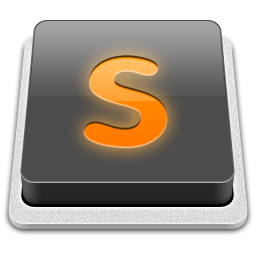
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
