


Loops and iterations: Core concepts in programming
Loops and iterations are essential concepts in programming that allow a program to repeatedly execute a set of instructions. Loops are used to explicitly specify the number of repetitions, while iterations are used to traverse the elements in a collection or data structure.
Loop type
There are three main types of loops:
1. for loop
The for loop is used to execute a block of code when you know the number of repetitions. Its syntax is as follows:
for (初始化; 条件; 递增/递减) { // 要重复执行的代码块 }
For example, the following for loop prints the numbers 1 to 10:
for (int i = 1; i <= 10; i++) { System.out.println(i); }
2. while loop
The while loop is used to execute a block of code when a condition is true. Its syntax is as follows:
while (条件) { // 要重复执行的代码块 }
For example, the following while loop will execute until the user enters "exit":
Scanner input = new Scanner(System.in); String userInput; while (!userInput.equals("退出")) { System.out.println("输入退出以终止循环:"); userInput = input.nextLine(); }
3. do-while loop
The do-while loop is similar to the while loop, but it executes the block of code at least once, even if the condition is false. Its syntax is as follows:
do { // 要重复执行的代码块 } while (条件);
For example, the following do-while loop will execute until the user enters "exit":
Scanner input = new Scanner(System.in); String userInput; do { System.out.println("输入退出以终止循环:"); userInput = input.nextLine(); } while (!userInput.equals("退出"));
Iteration
Iteration refers to traversing elements in a collection or data structure. The most common form of iteration is the foreach loop, which allows iterating over each element in a collection using a simplified syntax. The syntax of the foreach loop is as follows:
for (元素类型 元素名 : 集合名称) { // 要重复执行的代码块 }
For example, the following foreach loop iterates through each element in the list:
List<String> colors = new ArrayList<>(); colors.add("红色"); colors.add("绿色"); colors.add("蓝色"); for (String color : colors) { System.out.println(color); }
in conclusion
Understanding loops and iteration is the key to mastering programming. By using these concepts, you can write concise and efficient code that solves complex problems and simplifies complexity. Master the adventures of loops and iterations and embark on a magical programming journey!
The above is the detailed content of Adventures in Loops and Iteration: An Adventure in Python Code. For more information, please follow other related articles on the PHP Chinese website!
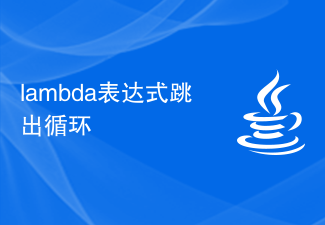
lambda表达式跳出循环,需要具体代码示例在编程中,循环结构是经常使用的一种重要语法。然而,在特定的情况下,我们可能希望在循环体内满足某个条件时,跳出整个循环,而不是仅仅终止当前的循环迭代。在这个时候,lambda表达式的特性可以帮助我们实现跳出循环的目标。lambda表达式是一种匿名函数的声明方式,它可以在内部定义简单的函数逻辑。它与普通的函数声明不同,
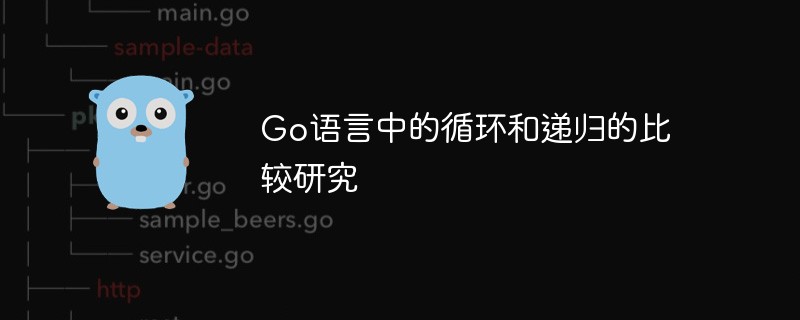
注:本文以Go语言的角度来比较研究循环和递归。在编写程序时,经常会遇到需要对一系列数据或操作进行重复处理的情况。为了实现这一点,我们需要使用循环或递归。循环和递归都是常用的处理方式,但在实际应用中,它们各有优缺点,因此在选择使用哪种方法时需要考虑实际情况。本文将对Go语言中的循环和递归进行比较研究。一、循环循环是一种重复执行某段代码的机制。Go语言中主要有三
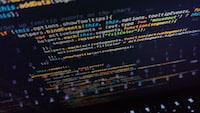
Iterator接口Iterator接口是一个用于遍历集合的接口。它提供了几个方法,包括hasNext()、next()和remove()。hasNext()方法返回一个布尔值,指示集合中是否还有下一个元素。next()方法返回集合中的下一个元素,并将其从集合中删除。remove()方法从集合中删除当前元素。以下代码示例演示了如何使用Iterator接口来遍历集合:Listnames=Arrays.asList("John","Mary","Bob");Iterator
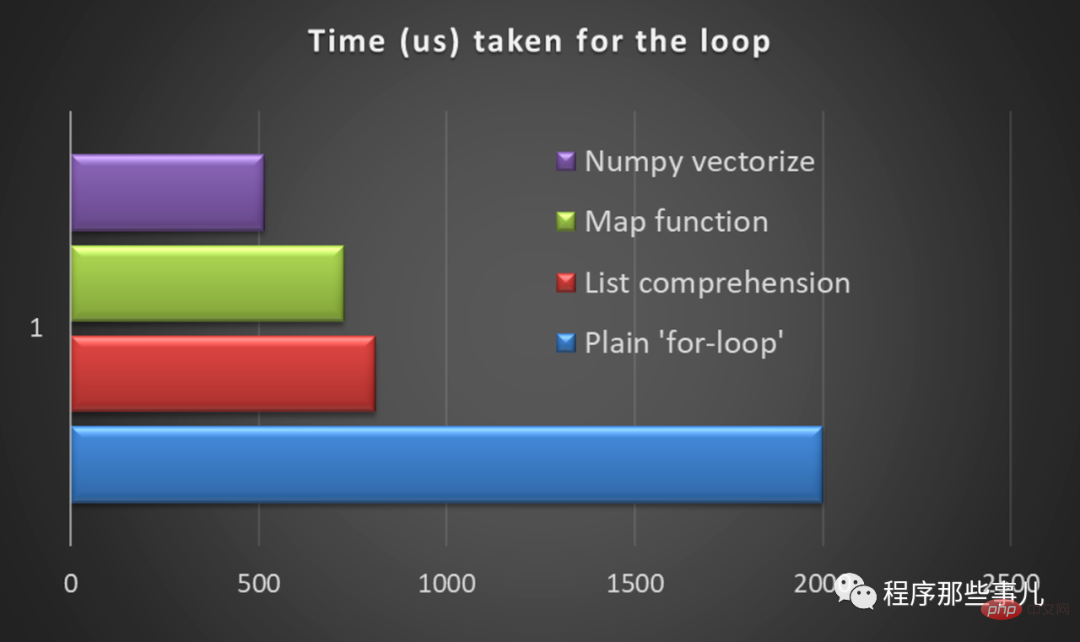
所有编程语言都离不开循环。因此,默认情况下,只要有重复操作,我们就会开始执行循环。但是当我们处理大量迭代(数百万/十亿行)时,使用循环是一种犯罪。您可能会被困几个小时,后来才意识到它行不通。这就是在python中实现矢量化变得非常关键的地方。什么是矢量化?矢量化是在数据集上实现(NumPy)数组操作的技术。在后台,它将操作一次性应用于数组或系列的所有元素(不同于一次操作一行的“for”循环)。接下来我们使用一些用例来演示什么是矢量化。求数字之和##使用循环importtimestart
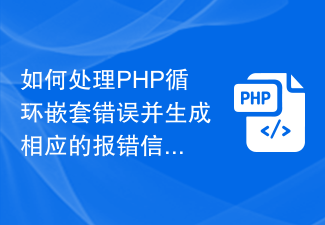
如何处理PHP循环嵌套错误并生成相应的报错信息在开发中,我们经常会用到循环语句来处理重复的任务,比如遍历数组、处理数据库查询结果等。然而,在使用循环嵌套的过程中,有时候会遇到错误,如无限循环或者嵌套层数过多,这种问题会导致服务器性能下降甚至崩溃。为了更好地处理这类错误,并生成相应的报错信息,本文将介绍一些常见的处理方式,并给出相应的代码示例。一、使用计数器来
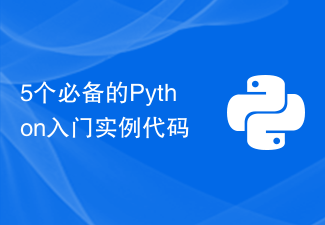
Python入门代码:学习必备的5个实例Python是一种简单易学的高级编程语言,广泛用于数据分析、机器学习、网络爬虫等领域。对于初学者来说,掌握一些基本的Python代码是很重要的。本文将介绍5个简单的实例代码,帮助初学者快速入门Python编程。打印Hello,World!print("Hello,World!")这是Python
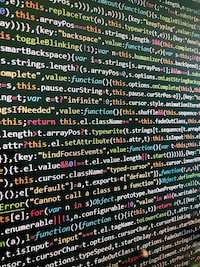
循环与迭代:编程中的核心概念循环和迭代是编程中必不可少的概念,它们允许程序重复执行一组指令。循环用于明确指定重复的次数,而迭代则用于遍历集合或数据结构中的元素。循环类型有三种主要类型的循环:1.for循环for循环用于当你知道重复次数时执行代码块。它的语法如下:for(初始化;条件;递增/递减){//要重复执行的代码块}例如,以下for循环打印数字1到10:for(inti=1;i
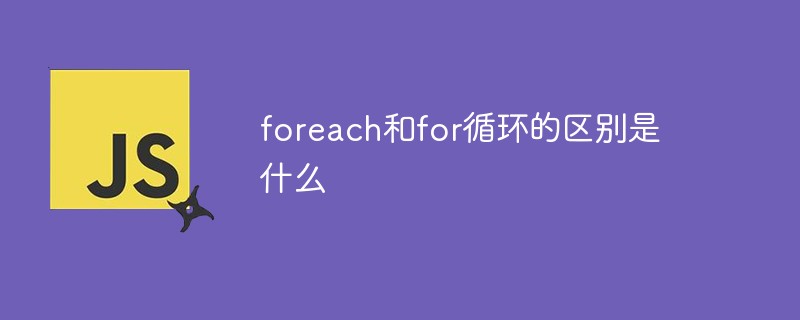
区别:1、for通过索引来循环遍历每一个数据元素,而forEach通过JS底层程序来循环遍历数组的数据元素;2、for可以通过break关键词来终止循环的执行,而forEach不可以;3、for可以通过控制循环变量的数值来控制循环的执行,而forEach不行;4、for在循环外可以调用循环变量,而forEach在循环外不能调用循环变量;5、for的执行效率要高于forEach。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
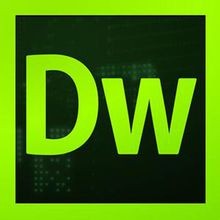
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
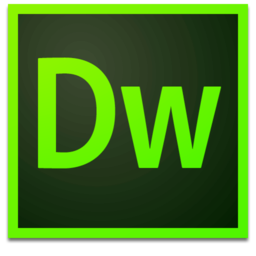
Dreamweaver Mac version
Visual web development tools