


Java Iterator and Iterable: An in-depth analysis of Java collection traversal mechanism
Iterator interface
Iterator and Iterable in Java are key interfaces in the Java collection framework, which provide convenience for traversing collections. In this article, PHP editor Xinyi will deeply analyze the working principles of Iterator and Iterable in Java to help readers better understand the traversal mechanism of Java collections.
-
hasNext()
: Check if there are more elements in the collection. -
next()
: Returns the next element in the collection.
Iterator interface also defines some optional methods, such as the remove()
method, which is used to remove the current element from the collection.
Use Iterator interface
You can use the following steps to traverse a collection using the Iterator interface:
- Get the Iterator object of the collection.
- Use the
hasNext()
method to check if there are more elements in the collection. - If there are more elements, use the
next()
method to get the next element. - Repeat steps 2 and 3 until there are no more elements in the set.
The following is an example of using the Iterator interface to traverse an ArrayList:
import java.util.ArrayList; import java.util.Iterator; public class IteratorExample { public static void main(String[] args) { // 创建一个 ArrayList ArrayList<String> names = new ArrayList<>(); // 向 ArrayList 中添加一些元素 names.add("John"); names.add("Mary"); names.add("Bob"); // 获取 ArrayList 的 Iterator 对象 Iterator<String> iterator = names.iterator(); // 使用 Iterator 对象遍历 ArrayList while (iterator.hasNext()) { String name = iterator.next(); System.out.println(name); } } }
Iterable interface
The Iterable interface is a high-level interface in the Java collection framework for traversing collections. It defines an iterator()
method that returns an Iterator object.
Use Iterable interface
You can use the following steps to traverse a collection using the Iterable interface:
- Get the Iterable object of the collection.
- Use the
iterator()
method to obtain an Iterator object. - Use an Iterator object to traverse the collection.
The following is an example of using the Iterable interface to traverse an ArrayList:
import java.util.ArrayList; import java.util.Iterable; public class IterableExample { public static void main(String[] args) { // 创建一个 ArrayList ArrayList<String> names = new ArrayList<>(); // 向 ArrayList 中添加一些元素 names.add("John"); names.add("Mary"); names.add("Bob"); // 获取 ArrayList 的 Iterable 对象 Iterable<String> iterable = names; // 使用 Iterable 对象获取一个 Iterator 对象 Iterator<String> iterator = iterable.iterator(); // 使用 Iterator 对象遍历 ArrayList while (iterator.hasNext()) { String name = iterator.next(); System.out.println(name); } } }
The difference between Iterator and Iterable interfaces
Iterator and Iterable interfaces are both interfaces for traversing collections, but there are some differences between them.
- Iterator is a low-level interface that directly operates on the elements in the collection.
- Iterable is a high-level interface that operates elements in the collection through the Iterator interface. The
- Iterator interface defines two main methods:
hasNext()
andnext()
. - The Iterable interface defines only one method:
iterator()
.
When to use the Iterator interface and when to use the Iterable interface
Generally speaking, if you need to directly operate the elements in the collection, you can use the Iterator interface. If you only need to iterate over the elements in a collection, you can use the Iterable interface.
For example, if you need to remove elements from a collection, you can use the remove()
method of the Iterator interface. If you only need to traverse the elements in the collection, you can use the iterator()
method of the Iterable interface to obtain an Iterator object, and then use the Iterator object to traverse the collection.
The above is the detailed content of Java Iterator and Iterable: An in-depth analysis of Java collection traversal mechanism. For more information, please follow other related articles on the PHP Chinese website!
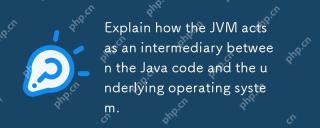
JVM works by converting Java code into machine code and managing resources. 1) Class loading: Load the .class file into memory. 2) Runtime data area: manage memory area. 3) Execution engine: interpret or compile execution bytecode. 4) Local method interface: interact with the operating system through JNI.

JVM enables Java to run across platforms. 1) JVM loads, validates and executes bytecode. 2) JVM's work includes class loading, bytecode verification, interpretation execution and memory management. 3) JVM supports advanced features such as dynamic class loading and reflection.

Java applications can run on different operating systems through the following steps: 1) Use File or Paths class to process file paths; 2) Set and obtain environment variables through System.getenv(); 3) Use Maven or Gradle to manage dependencies and test. Java's cross-platform capabilities rely on the JVM's abstraction layer, but still require manual handling of certain operating system-specific features.
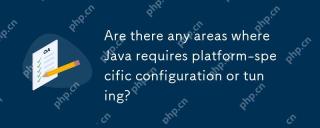
Java requires specific configuration and tuning on different platforms. 1) Adjust JVM parameters, such as -Xms and -Xmx to set the heap size. 2) Choose the appropriate garbage collection strategy, such as ParallelGC or G1GC. 3) Configure the Native library to adapt to different platforms. These measures can enable Java applications to perform best in various environments.
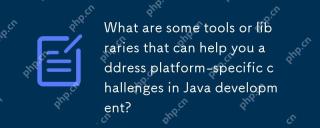
OSGi,ApacheCommonsLang,JNA,andJVMoptionsareeffectiveforhandlingplatform-specificchallengesinJava.1)OSGimanagesdependenciesandisolatescomponents.2)ApacheCommonsLangprovidesutilityfunctions.3)JNAallowscallingnativecode.4)JVMoptionstweakapplicationbehav
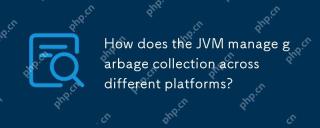
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
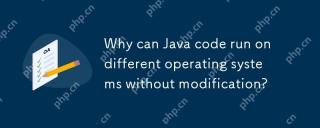
Java code can run on different operating systems without modification, because Java's "write once, run everywhere" philosophy is implemented by Java virtual machine (JVM). As the intermediary between the compiled Java bytecode and the operating system, the JVM translates the bytecode into specific machine instructions to ensure that the program can run independently on any platform with JVM installed.
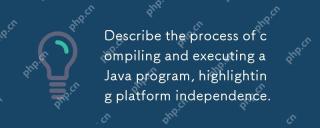
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
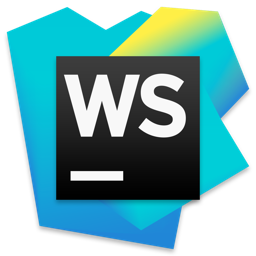
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor
