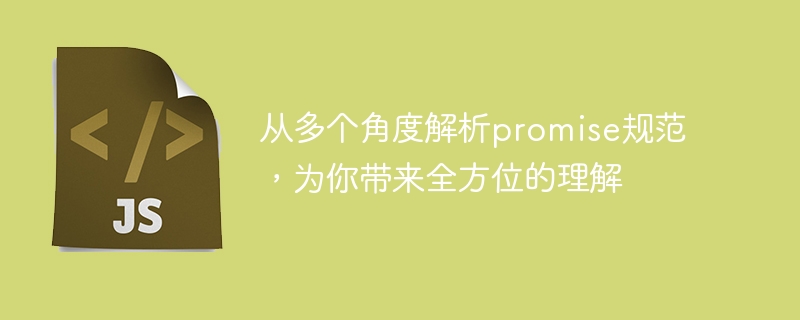
Analyze Promise specifications from multiple angles to bring you a comprehensive understanding
1. Overview
Promise is a type of JavaScript Programming pattern for handling asynchronous operations. Promises provide an elegant way to organize and handle asynchronous code, making the code more readable and maintainable. The Promise specification defines the behavior and methods of Promise, ensuring interoperability between different implementations.
2. The basic concept of Promise
- Promise object: Promise is an object that represents the result of an asynchronous operation. It can have three states: Pending (in progress), Fulfilled (successful) and Rejected (failed).
- resolve and reject: The Promise object has two internal methods, resolve and reject, which are used to change the state of the Promise.
- then method: The then method of the Promise object is used to add a callback function when the state changes. It accepts two parameters, one is the callback function for the Fulfilled state, and the other is the callback function for the Rejected state.
3. Advantages of Promise
- High readability: Through chain calls, Promise makes the logic of asynchronous code easier to read and understand.
- Avoid callback hell: Promise can call the then method in a chain and return a new Promise object inside each callback function, thereby avoiding the problem of callback hell.
- Convenient error capture: Promise objects can capture errors through the catch method and handle them uniformly.
- Support concurrent execution: The Promise.all method can execute multiple Promise objects at the same time and wait for them to complete.
4. Usage examples of Promise
- Basic usage:
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Hello, Promise!');
}, 1000);
});
promise.then(value => {
console.log(value); // 输出:Hello, Promise!
});
- Promise chain:
fetch(url)
.then(response => response.json())
.then(data => {
// 处理数据
})
.catch(error => {
// 错误处理
});
- Concurrent execution of Promise:
const promise1 = fetch(url1);
const promise2 = fetch(url2);
const promise3 = fetch(url3);
Promise.all([promise1, promise2, promise3])
.then(values => {
// 处理返回的数据
})
.catch(error => {
// 错误处理
});
5. Implementation principle of Promise
The implementation principle of Promise can be simply summarized in the following steps:
- Create a Promise object and set the initial state to Pending.
- Inside the Promise object, two functions, resolve and reject, are defined to change the state of the Promise.
- Call the executor function (the function passed to the Promise constructor), passing resolve and reject as parameters.
- Perform asynchronous operations in the executor function and call resolve or reject at the appropriate time.
- The Promise object registers a callback function when the state changes through the then method.
- After the asynchronous operation is completed, call resolve or reject to change the state of the Promise.
- According to the state of Promise, execute the corresponding callback function.
6. Extended application of Promise
- The Generator function cooperates with Promise: the asynchronous task is packaged into a Promise object through the yield keyword to achieve synchronous writing.
- async/await: The await keyword can be used inside an asynchronous function defined using the async keyword to wait for the Promise object to be resolved or rejected.
7. Conclusion
The Promise specification provides a more elegant and readable asynchronous programming method for JavaScript. In actual development, reasonable use of Promise can improve the maintainability and scalability of the code. Through analysis from multiple angles, we can understand the Promise specification more comprehensively and apply it to actual projects.
The above is the detailed content of An in-depth discussion of promise specifications to help you fully understand. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn