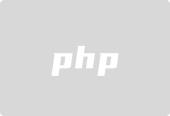
python Current status and trends of concurrent programming
In recent years, PythonConcurrentprogramming has made significant progress, and the rise of asyncio libraries is one of the major trends. asyncio is an asynchronous I/O library that allows developers to write concurrent, non-blocking code. This is very efficient for handling large numbers of concurrent connections and events.
In addition,
Multi-threading
and traditional concurrency technologies such as multi-process are still widely used. MultiThreading allows multiple tasks to be executed simultaneously within one process, while multi-process creates multiple tasks in different processes.
It is worth noting that the futures library provides a unified interface for asynchronous and synchronous code. It allows developers to easily use different concurrency methods and switch between them as needed.
Opportunities of concurrent programming
Concurrent programming in Python provides the following key opportunities:
Efficient utilization of multi-core processors: - Concurrent programming allows applications to perform tasks on multiple cores simultaneously, significantly improving performance.
Improve application response speed: - Asynchronous I/O and non-blocking code can reduce the application's dependence on blocking operations, thus improving response speed.
Scalability: - Concurrent programming allows applications to handle more connections and events, thereby improving scalability.
Challenges of Concurrent Programming
Despite these opportunities, Python concurrent programming also faces some key challenges:
Debugging concurrent code: - Debugging concurrent code is more challenging than sequential code because multiple tasks interact at the same time.
Managing concurrent shared state: - Managing shared state in a concurrent environment can be complex and can lead to race conditions and dead locks.
Performance optimization: -
Performance optimization in concurrent programming is a complex task, which involves the selection of parallelism, the use of thread pool and avoiding GIL s expenses.
Strategies for Overcoming Challenges
To overcome these challenges, developers can employ the following strategies:
Use asyncio and futures: - They simplify asynchronous and concurrent programming and provide flexible interfaces.
Use thread pool: - Thread pool can manage threads, thereby reducing the cost of creating and destroying threads.
Using lock and synchronization primitives: - These primitives can help developers control concurrent access to shared state, thereby preventing race conditions.
Perform performance analysis: - Using performance analysis tools can help identify performance bottlenecks and optimize code.
Code Example
The following is a code example demonstrating asyncio:
import asyncio
async def hello_world():
print("Hello, world!")
async def main():
await asyncio.gather(hello_world(), hello_world())
asyncio.run(main())
This example creates two concurrent tasks that will run in two separate coroutines.
in conclusion
The future of concurrent programming in Python is bright. It provides a range of opportunities to improve application performance, responsiveness and scalability. By overcoming the challenges of debugging, shared state management, and performance optimization, developers can take advantage of Python's concurrency features to create efficient and reliable applications. As asyncio and other technologies continue to evolve, Python will continue to play a vital role in the world of concurrent programming.
The above is the detailed content of The future of concurrent programming in Python: Exploring evolving opportunities and challenges. For more information, please follow other related articles on the PHP Chinese website!