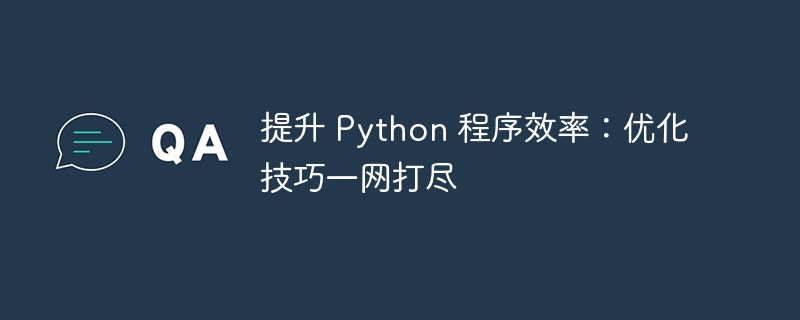
1. Code structure optimization
-
Modular development: Decompose large programs into smaller modules to achieve code reusability and maintainability and avoid excessive nesting.
-
Use object-oriented programming: Encapsulate data and behavior to improve the scalability and readability of the code.
-
Avoid global variables: Use local variables instead of global variables to reduce naming conflicts and improve program maintainability.
# 模块化开发示例
def calculate_average(nums):
return sum(nums) / len(nums)
def print_average(nums):
print(calculate_average(nums))
# 调用模块
nums = [1, 2, 3, 4, 5]
print_average(nums)
2. Algorithm optimization
-
Choose the appropriate algorithm: Choose an efficient algorithm based on the amount of data and computing requirements, such as binary search and hash table.
-
Optimize algorithm parameters: Adjust the parameters of the algorithm to obtain the best performance, such as the parameters of the hash function.
-
Divide and conquer: Decompose the problem into smaller sub-problems and solve them step by step to improve efficiency.
# 二分查找示例
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
3. Data structure optimization
-
Select the appropriate container: Select the appropriate data structure based on the data access mode and storage requirements, such as list, dictionary, set.
-
Avoid unnecessary copying: Use reference passing instead of value passing to reduce memory overhead.
-
Pre-allocated memory: Allocate the required memory in advance to avoid frequent memory allocation and recycling.
# 预分配内存示例
import array
# 为存储 1000 个整数预分配内存
arr = array.array("i", [0] * 1000)
4. Other optimization techniques
-
Use performance analysis tools: such as cProfile or timeit to analyze code performance and identify performance bottlenecks.
-
Avoid unnecessary function calls: Try to inline function calls to reduce function call overhead.
-
Pay attention to memory usage: Optimize memory usage to avoid memory leaks and performance degradation.
-
Take full advantage of multi-core processors: Use Multi-threading or multiple processes to take full advantage of multi-core processors.
# 多线程示例
import threading
def task(a, b):
return a * b
# 创建并启动线程
thread1 = threading.Thread(target=task, args=(1, 2))
thread2 = threading.Thread(target=task, args=(3, 4))
thread1.start()
thread2.start()
# 等待线程结束并获取结果
result1 = thread1.join()
result2 = thread2.join()
in conclusion
By adopting these optimization techniques, the efficiency of the python program can be significantly improved. By optimizing code structure, choosing appropriate algorithms, rational use of data structures, and applying other optimization techniques, you can maximize the power of Python and create high-performance and efficient programs.
The above is the detailed content of Improve Python program efficiency: optimization tips all in one place. For more information, please follow other related articles on the PHP Chinese website!