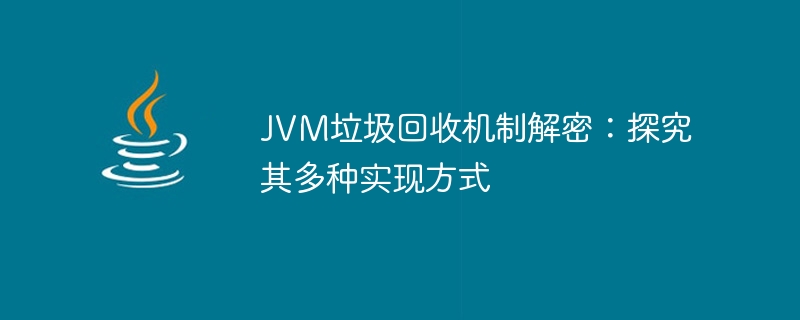
Decryption of JVM garbage collection mechanism: To explore its multiple implementation methods, specific code examples are required
Abstract:
Garbage collection is a key component of the Java Virtual Machine (JVM) One of the important functions is that it can automatically manage memory and reduce the burden on programmers. This article will delve into the various implementation methods of JVM garbage collection and provide specific code examples to help readers better understand its working principle and usage.
- Introduction
With the development of computer science, memory management has become an extremely important issue. Especially in object-oriented programming languages, using a dynamic memory allocation mechanism requires an automatic memory recycling mechanism. The JVM's garbage collection mechanism is designed to solve this problem.
- Basic principles of garbage collection
Before introducing the implementation of JVM garbage collection, let us first understand the basic principles of garbage collection. The garbage collection mechanism performs memory recycling through marking and clearing.
Marking phase: The JVM will traverse all objects in the memory starting from the root object and mark all referenced objects.
Clear phase: JVM will clear other objects except the marked objects so that the memory space occupied by these objects can be reused.
- Implementation methods of JVM garbage collection
JVM garbage collection mechanism has many implementation methods, common ones include:
- Reference Counting algorithm (Reference Counting): This algorithm passes Add a reference counter to each object. When an object is referenced, the counter is incremented by 1. When the reference is invalid, the counter is decremented by 1. When the reference counter reaches 0, the object can be recycled. However, the reference counting algorithm cannot solve the problem of circular references, so it is not common in practical use.
Sample code:
class Object {
private int count;
public Object() {
count = 0;
}
public void addReference() {
count++;
}
public void removeReference() {
count--;
if (count == 0) {
// 回收对象
}
}
}
- Mark-Sweep algorithm (Mark-Sweep): This algorithm marks all reachable objects through mark traversal, and then clears the unreachable objects. The marked object. This algorithm can solve the problem of circular references, but it will produce memory fragmentation.
Sample code:
void markAndSweep() {
mark(root); // 从根对象开始标记
sweep(); // 清除未被标记的对象
}
void mark(Object object) {
if (!object.marked) {
object.marked = true; // 标记对象
for (Object reference : object.references) {
mark(reference); // 递归标记引用对象
}
}
}
void sweep() {
for (Object object : objects) {
if (!object.marked) {
// 回收对象
} else {
object.marked = false; // 清除标记
}
}
}
- Copying algorithm (Copying): This algorithm divides the memory into two areas, and only uses one area at a time. When one area is full, all surviving objects are copied to another area, and then the entire area is emptied. This algorithm can solve the problem of memory fragmentation, but requires additional memory space to store the copied objects.
Sample code:
void copy() {
for (Object object : objects) {
if (object.marked) {
// 将对象复制到另一块区域
}
}
}
- Summary
This article delves into the various implementation methods of JVM garbage collection and provides specific code examples. Different implementation methods have their own advantages and disadvantages, and you can choose the appropriate method according to different application scenarios. I hope this article can help readers better understand the working principle and usage of JVM garbage collection, and be able to correctly use the garbage collection mechanism in actual development.
The above is the detailed content of Demystifying the JVM garbage collection mechanism: in-depth discussion of different implementation methods. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn