PHP SPL data structure best practices: ensuring code robustness
php editor Banana introduces you to the best practices of PHP SPL data structure. These practices can ensure that your code is more robust. By using various data structures provided by the PHP standard library, such as SplStack, SplQueue, and SplHeap, you can improve the performance and maintainability of your code, making your application more stable and efficient. When writing PHP code, it is very important to properly apply SPL data structures. Let us learn how to use these tools to improve code quality and efficiency!
ADT defines a set of operations and attributes that are used to describe data types abstractly. SPL provides a large number of ADT implementations, including arrays, collections, queues, and stacks. Choosing the right ADT is critical because it affects the behavior and overhead of your code.
- Array (ArrayObject): Ordered collection, used to store key-value pairs.
- SetObject (SetObject): Unordered collection, used to store unique elements.
- Queue (QueueObject): First in first out (FIFO) Data structure, used to process messages and events.
- Stack (StackObject): Last-in-first-out (LIFO) data structure used for recursive processing and function calls.
2. Use iterator to traverse
Iterators provide a unified way to traverse elements in a data structure. SPL provides an extensive iterator interface that allows data to be traversed in a variety of ways. This makes the code more flexible and reduces the need for hard-coded loops.
Sample code:
$array = new ArrayObject(["a", "b", "c"]); foreach ($array as $key => $value) { echo "Key: $key, Value: $value "; }
3. Use stack expansion (SplStack)
Stacks provide an easy way to manage nested blocks. By using the SplStack
class, you can easily push and pop objects to keep track of the current execution context. This is useful for recursive algorithms and state management.
Sample code:
$stack = new SplStack(); $stack->push(1); $stack->push(2); while (!$stack->isEmpty()) { $value = $stack->pop(); echo "Value: $value "; }
4. Ensure object type consistency
The SPL data structure is designed to allow storage of different types of data. However, to keep your code maintainable, it is recommended to enforce object type consistency. This can be achieved using the instanceof
operator or type hints.
Sample code:
$array = new ArrayObject(); $array->setIteratORMode(ArrayObject::STD_PROP_LIST); // 仅允许存储字符串 foreach ($array as $key => &$value) { if (!is_string($value)) { throw new InvalidArgumentException("Value must be a string"); } }
5. Take advantage of advanced features
The SPL data structure provides many advanced features that can further enhance the robustness and efficiency of your code. These features include:
- Filter and Sort (FilterIterator, SortIterator)
- UnionIterator, IntersectIterator)
- ReverseIterator, Countable)
- Serialization and Clone (Serializable, Cloneable)
Sample code:
$array = new ArrayObject(["a", "b", "c"]); // 过滤奇数元素 $filteredArray = new FilterIterator($array, function ($value) { return $value % 2; }); // 输出奇数元素 foreach ($filteredArray as $value) { echo $value . " "; }
in conclusion
Using PHP SPL data structure can significantly improve the efficiency and robustness of complex data processing. By following these best practices, developers can take full advantage of these data structures and write maintainable and efficient code. From selecting the right ADT to leveraging advanced features, SPL data structures provide a wide range of tools to meet a variety of data processing needs.
The above is the detailed content of PHP SPL data structure best practices: ensuring code robustness. For more information, please follow other related articles on the PHP Chinese website!
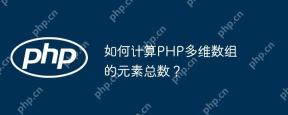
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
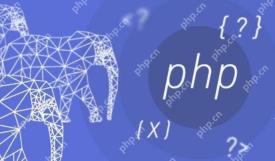
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
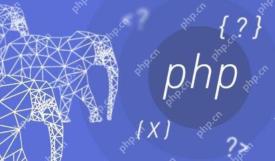
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.
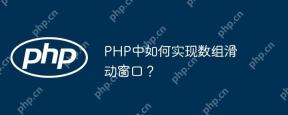
Implementing an array sliding window in PHP can be done by functions slideWindow and slideWindowAverage. 1. Use the slideWindow function to split an array into a fixed-size subarray. 2. Use the slideWindowAverage function to calculate the average value in each window. 3. For real-time data streams, asynchronous processing and outlier detection can be used using ReactPHP.
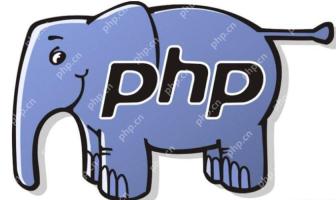
The __clone method in PHP is used to perform custom operations when object cloning. When cloning an object using the clone keyword, if the object has a __clone method, the method will be automatically called, allowing customized processing during the cloning process, such as resetting the reference type attribute to ensure the independence of the cloned object.
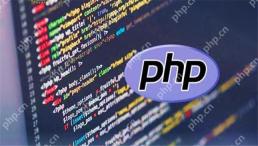
In PHP, goto statements are used to unconditionally jump to specific tags in the program. 1) It can simplify the processing of complex nested loops or conditional statements, but 2) Using goto may make the code difficult to understand and maintain, and 3) It is recommended to give priority to the use of structured control statements. Overall, goto should be used with caution and best practices are followed to ensure the readability and maintainability of the code.
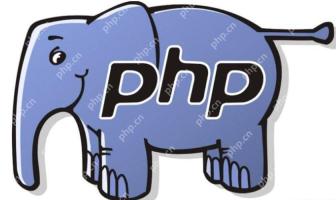
In PHP, data statistics can be achieved by using built-in functions, custom functions, and third-party libraries. 1) Use built-in functions such as array_sum() and count() to perform basic statistics. 2) Write custom functions to calculate complex statistics such as medians. 3) Use the PHP-ML library to perform advanced statistical analysis. Through these methods, data statistics can be performed efficiently.
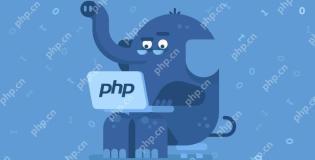
Yes, anonymous functions in PHP refer to functions without names. They can be passed as parameters to other functions and as return values of functions, making the code more flexible and efficient. When using anonymous functions, you need to pay attention to scope and performance issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
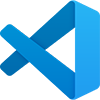
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
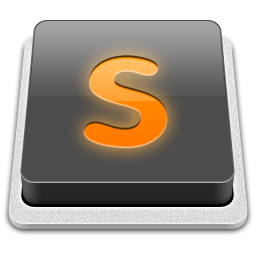
SublimeText3 Mac version
God-level code editing software (SublimeText3)
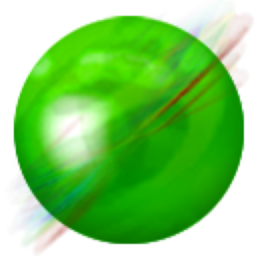
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
