A Practical Guide to Java Reflection: From Beginner to Mastery
1. JavaReflectionGetting Started
The "Java Reflection Practical Guide: From Beginner to Master" recommended by php editor Xinyi is a simple and easy-to-understand Java programming guide, which comprehensively introduces the basic knowledge and practical application skills of reflection. This book aims to help readers systematically learn the Java reflection mechanism, from entry to proficiency, master the application of reflection in actual projects, and improve programming skills.
1. Get class information
Obtaining class information is one of the most basic functions of reflection. We can obtain class information through various methods of the Class
class, such as:
// 获取类的名称 String className = Class.getName(); // 获取类的父类名称 String superClassName = Class.getSuperclass().getName(); // 获取类的所有字段 Field[] fields = Class.getFields(); // 获取类的所有方法 Method[] methods = Class.getMethods();
2. Create an instance of the class
Reflection can also be used to create instances of classes. We can use the newInstance()
method to create an instance of a class, such as:
// 创建一个类的实例 Object instance = Class.newInstance();
3. Call the constructor, methods and fields of the class
Reflection can also be used to call the constructor, methods and fields of a class. We can use the getConstructor()
, getMethod()
and getField()
methods to get these members, and then use the invoke()
method to call them, such as:
// 获取类的构造方法 Constructor<?> constructor = Class.getConstructor(int.class); // 创建一个类的实例 Object instance = constructor.newInstance(10); // 获取类的字段 Field field = Class.getField("name"); // 设置字段的值 field.set(instance, "John"); // 获取类的某个方法 Method method = Class.getMethod("getName"); // 调用该方法 String name = (String) method.invoke(instance);
2. Java reflection practice
In actual development, reflection can be used to implement many functions, such as:
1. Dynamically loading classes
Reflection can be used to dynamically load classes. This is very useful when developing plugins or extensions because we can dynamically load and run the plugin or extension at runtime without the need to recompile and deploy the entire application.
// 动态加载一个类 Class<?> clazz = Class.forName("com.example.MyClass"); // 创建一个类的实例 Object instance = clazz.newInstance(); // 调用类的某个方法 Method method = clazz.getMethod("getName"); // 调用该方法 String name = (String) method.invoke(instance);
2. Check whether the class implements an interface
Reflection can be used to check whether a class implements an interface. This is very useful when writing frameworks or libraries because we can check the types of classes to determine if they are compatible with the framework or library.
// 检查类是否实现某个接口 boolean isInterface = Class.isInterface();
3. Check whether the class is derived from a certain class
Reflection can be used to check whether a class is derived from a certain class. This is also very useful when writing a framework or library as we can check the types of classes to determine if they are compatible with the framework or library.
// 检查类是否派生自某个类 boolean isAssignableFrom = Class.isAssignableFrom(Class);
3. Notes on Java Reflection
When using reflection, you need to pay attention to the following points:
1. Performance overhead
Reflection is a dynamic operation and therefore slower than calling methods or fields directly. Therefore, reflection should be used with caution in scenarios with high performance requirements.
2. SafetySex
Reflection can be used to access private members. This can be a safety hazard, so you should be careful when using reflection.
3. Compatibility
Reflection may differ in different versions of Java. Therefore, you should pay attention to compatibility issues when writing code that uses reflection.
Conclusion
Reflection is a powerful technology in the Java language that can be used to obtain class information and perform dynamic operations. In actual development, reflection can be used to implement many functions, such as dynamically loading classes, checking whether a class implements a certain interface, checking whether a class is derived from a certain class, etc. When using reflection, you need to pay attention to performance overhead and security
The above is the detailed content of A Practical Guide to Java Reflection: From Beginner to Mastery. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
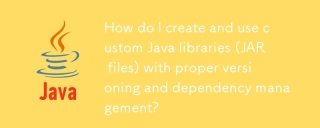
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
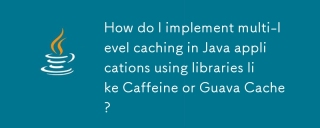
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
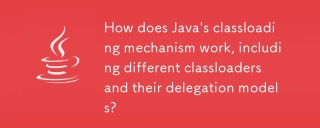
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
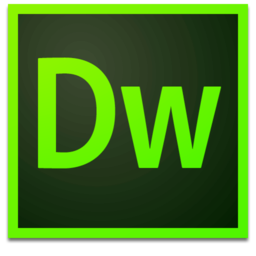
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.