How does the new operator work in js?
What is the working principle of the new operator in js, specific code examples are needed
The new operator in js is the keyword used to create objects. Its function is to create a new instance object based on the specified constructor and return a reference to the object. When using the new operator, the following steps are actually performed:
- Create a new empty object;
- Point the prototype of the empty object to the prototype object of the constructor ;
- Assign the scope of the constructor to the new object (so this points to the new object);
- Execute the code in the constructor and add properties and methods to the new object;
- If the constructor returns an object, return that object; otherwise, return a new object.
The following is a simple example to illustrate how the new operator works:
// 定义一个构造函数 function Person(name, age) { this.name = name; this.age = age; } // 使用new操作符创建一个实例对象 var person1 = new Person('Tom', 18); // 输出实例对象的属性值 console.log(person1.name); // 输出 'Tom' console.log(person1.age); // 输出 18
In the above code, we define a constructor named Person. The constructor accepts two parameters, name and age, and uses them as attributes of the instance object person1.
When using the new operator to create a person1 instance, an empty object is first created, and then the prototype of the empty object is pointed to the prototype object of the constructor Person. Next, the scope of the constructor is assigned to the empty object, so that the empty object can be accessed through this inside the constructor. Finally, the code in the constructor is executed and name and age are assigned to the properties of the new object.
Therefore, the object person1 created by the new operator has the attributes name and age defined in the constructor Person, and these attributes can be accessed through the dot operator.
It should be noted that do not explicitly return an object inside the constructor. If the constructor returns an object, the instance created by the new operator will be the returned object, not the newly created object. For example:
// 定义一个构造函数 function Person(name, age) { this.name = name; this.age = age; // 错误示例:返回一个对象 return { name: 'Error', age: -1 }; } // 使用new操作符创建一个实例对象 var person1 = new Person('Tom', 18); // 此时person1实际上是一个普通的对象,而不是Person的实例 console.log(person1 instanceof Person); // 输出 false console.log(person1.name); // 输出 'Error' console.log(person1.age); // 输出 -1
In the above example, the constructor Person explicitly returns an object, so person1 is not actually an instance of Person, but an ordinary object.
To sum up, the new operator is used to create a new instance object and initialize the properties of the object in the constructor. The instance object created through the new operator inherits the prototype object of the constructor and can access the properties and methods defined in the constructor.
The above is the detailed content of How does the new operator work in js?. For more information, please follow other related articles on the PHP Chinese website!
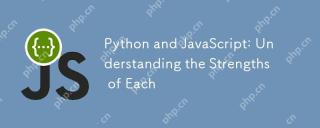
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
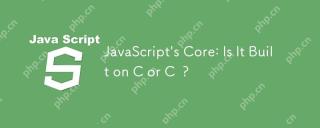
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
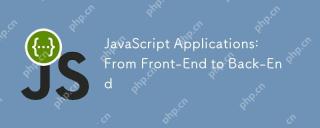
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
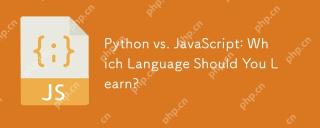
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
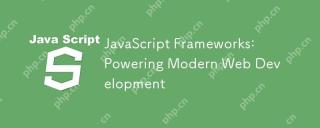
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
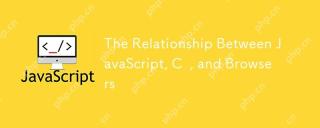
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
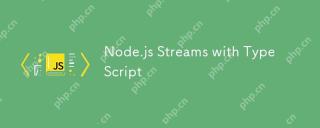
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
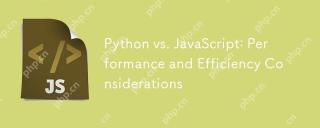
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
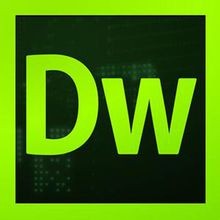
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
