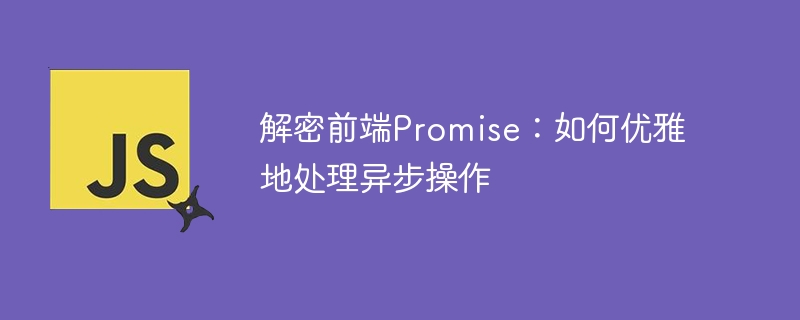
Decrypting front-end Promise: How to handle asynchronous operations gracefully
Introduction:
In front-end development, we often encounter situations that require asynchronous operations, such as Get data from the server, send HTTP requests, handle user input, and more. In JavaScript, these asynchronous operations can be handled elegantly using Promise objects. This article will provide an in-depth analysis of how Promise works and how to use Promise to achieve clearer and more readable asynchronous code.
1. What is Promise?
Promise is a design pattern and implementation mechanism introduced in ES6 for managing asynchronous operations. It can encapsulate asynchronous operations into an object and organize and manage these operations in a chained call manner, making the code easier to understand and maintain. Promise has three states: pending, fulfilled, and rejected.
2. Basic usage of Promise
- Creating a Promise object
First, we can create a Promise object through the Promise constructor. The constructor accepts a function as a parameter, which will be executed immediately, and accepts two functions as parameters, namely resolve and reject. The resolve function is used to change the Promise object from the waiting state to the completion state, and the reject function changes the Promise object from the waiting state to the rejection state.
const promise = new Promise((resolve, reject) => {
// 异步操作
// 操作成功时调用resolve
// 操作失败时调用reject
})
- Chained calling Promise
Promise provides the then method for chained calling. The then method accepts two parameters, namely the success callback function and the failure callback function. If the asynchronous operation succeeds, that is, the resolve function is called, the success callback function will be executed; if the asynchronous operation fails, that is, the reject function is called, the failure callback function will be executed.
promise.then(
function(data) {
// 异步操作成功时执行的代码
},
function(error) {
// 异步操作失败时执行的代码
}
)
- Promise chain call
Promise provides a very important feature, which is that multiple asynchronous operations can be organized and managed through chain calls. After returning a new Promise instance in the then method, you can continue to call the then method on the Promise instance, and so on.
promise.then(
function(data) {
// 第一个异步操作成功时执行的代码
return newPromise;
}
).then(
function(data) {
// 第二个异步操作成功时执行的代码
}
)
3. Advantages of Promise
- Improve code readability and maintainability
Using Promise can split and organize the logic of asynchronous operations. Makes the code clearer and easier to understand. Use the then method to make chain calls. Each then method can handle the success or failure of an asynchronous operation, making the logic more modular.
- Solving the callback hell problem
The traditional callback function method will cause the callback hell problem when processing multiple asynchronous operations, making the code difficult to maintain and expand. Using Promise can solve the callback hell problem through chain calls and clearly express the dependencies between asynchronous operations.
- Uniform exception handling
Promise provides a catch method for uniformly handling exceptions in asynchronous operations. By adding a catch method at the end of the chain of calls, exceptions that occur in the entire chain of calls can be captured to facilitate error handling.
4. Further application of Promise
In addition to the basic usage, Promise also has some further application techniques to make the code more concise and easier to maintain.
- Processing multiple asynchronous operations in parallel
In some cases, we need to perform multiple asynchronous operations at the same time and wait for them all to complete before performing other operations. Promise provides the Promise.all method, which accepts an array of Promise instances as parameters and returns a new Promise instance. When all Promise instances enter the completion state, the Promise instance will enter the completion state.
const promises = [promise1, promise2, promise3];
Promise.all(promises)
.then(function(data) {
// 所有异步操作都成功完成时执行的代码
})
.catch(function(error) {
// 任何一个异步操作失败时执行的代码
});
- Processing the first completed asynchronous operation
In some cases, we only need the result of the first completed asynchronous operation without waiting for all asynchronous operations to complete. Promise provides the Promise.race method, which accepts an array of Promise instances as a parameter and returns a new Promise instance. When any of the Promise instances enters the completion state, the Promise instance will enter the completion state.
const promises = [promise1, promise2, promise3];
Promise.race(promises)
.then(function(data) {
// 最快的一个异步操作完成时执行的代码
})
.catch(function(error) {
// 如果最快的一个异步操作失败时执行的代码
});
Conclusion:
Using Promise can handle asynchronous operations in the front end more elegantly, promote the readability and maintainability of the code, solve the callback hell problem, and provide convenient errors processing method. At the same time, Promise can also be used in scenarios such as processing multiple asynchronous operations in parallel and processing the first completed asynchronous operation. Mastering the use of Promise will help improve the efficiency and code quality of front-end development.
The above is the detailed introduction of this article about decrypting front-end Promise. I hope it will be helpful to readers when dealing with asynchronous operations.
The above is the detailed content of Front-end Promise decryption: Tips for elegantly handling asynchronous operations. For more information, please follow other related articles on the PHP Chinese website!