Exploring the types and conversion rules of Java thread states
A thread is an execution unit in Java that can run independently and execute concurrently with other threads. In Java, threads have multiple states that reflect the behavior and conditions of the thread at different stages. This article will explore the status types and transition rules of Java threads and provide specific code examples.
The main types of Java thread states are as follows:
- New state (New): The thread is created but has not been started yet.
- Running status (Runnable): The thread is started and can run, including the status of running and about to or waiting for CPU resources.
- Blocked state (Blocked): The thread is suspended from execution, usually because it is waiting for a certain condition to occur and cannot continue to execute.
- Waiting state (Waiting): The thread suspends execution until other threads send it a wake-up notification.
- Timed Waiting state (Timed Waiting): The thread suspends execution until other threads send it a wake-up notification or the specified time arrives.
- Termination status (Terminated): The thread has completed execution or exited due to an exception.
The conversion rules between thread states are as follows:
- New state -> Running state: Call the start() method of the thread object to start the thread.
- Running status -> Blocking status: The thread is waiting for the release of a lock, or the thread calls the static sleep() method of the Thread class.
- Running status -> Waiting status: The thread calls the wait() method of the Object class.
- Running status -> Timing waiting status: The thread calls the static sleep() method of the Thread class and specifies the time.
- Blocking state -> Running state: The lock is released or the blocked thread is awakened.
- Waiting state -> Running state: Other threads send wake-up notifications to waiting threads.
- Timed waiting state -> Running state: Time arrives or other threads send wake-up notifications to the timed waiting thread.
- Running status -> Termination status: The thread has completed execution or exited due to an exception.
The following is a specific code example that demonstrates the Java thread state conversion process:
public class ThreadStateExample { public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(() -> { try { Thread.sleep(1000); // 让线程进入计时等待状态 synchronized (ThreadStateExample.class) { ThreadStateExample.class.wait(); // 让线程进入等待状态 } } catch (InterruptedException e) { e.printStackTrace(); } }); System.out.println("线程状态: " + thread.getState()); // 输出:线程状态: NEW thread.start(); System.out.println("线程状态: " + thread.getState()); // 输出:线程状态: RUNNABLE Thread.sleep(500); // 等待500毫秒,让线程执行一段时间 System.out.println("线程状态: " + thread.getState()); // 输出:线程状态: TIMED_WAITING synchronized (ThreadStateExample.class) { ThreadStateExample.class.notify(); // 唤醒等待线程 } Thread.sleep(500); // 等待500毫秒,让线程执行一段时间 System.out.println("线程状态: " + thread.getState()); // 输出:线程状态: TERMINATED } }
Run the above code, you can observe the thread state conversion process. By knowing and understanding thread status, we can better control thread behavior and avoid potential threading problems. In actual development, rational use of thread status can improve the efficiency and stability of multi-threaded programs.
Summary:
This article explores the types and conversion rules of Java thread states, and provides specific code examples. Understanding the thread state conversion rules helps us better understand and use multi-threaded programming. When writing multi-threaded programs, we can handle and optimize the different states of threads accordingly. I hope this article will be helpful to everyone’s study and practice.
The above is the detailed content of Discuss the classification of Java thread states and state transfer rules. For more information, please follow other related articles on the PHP Chinese website!
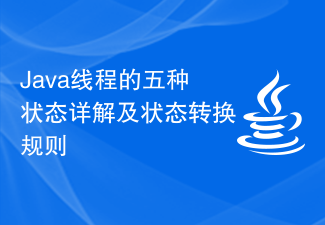
深入了解Java线程的五种状态及其转换规则一、线程的五种状态介绍在Java中,线程的生命周期可以分为五个不同的状态,包括新建状态(NEW)、就绪状态(RUNNABLE)、运行状态(RUNNING)、阻塞状态(BLOCKED)和终止状态(TERMINATED)。新建状态(NEW):当线程对象创建后,它就处于新建状态。此时,线程对象已经分配了足够的资源来执行任务
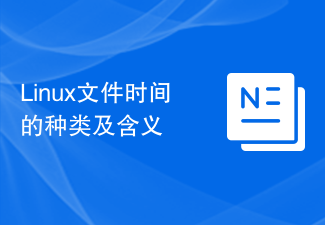
Linux文件时间的种类及含义在Linux操作系统中,每个文件都有三种不同类型的时间戳,分别是访问时间(atime)、修改时间(mtime)和改变时间(ctime)。这三种时间戳记录了文件在不同操作下的变化,下面将详细解释它们的含义并提供相应的代码示例。访问时间(atime):访问时间是指文件最后一次被访问的时间。当文件被读取、运行或浏览时,atime会更新
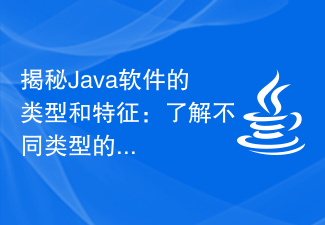
Java软件大揭秘:探寻Java软件的种类和特点,需要具体代码示例Java是一种广泛使用的计算机编程语言,具有跨平台特性、可移植性强、面向对象等优势,因此在软件开发领域中得到了广泛应用。本文将深入探讨Java软件的种类和特点,并通过具体的代码示例展示其强大的功能。一、Java应用程序Java应用程序通常是指能够直接在操作系统上运行的独立程序。它们可以通过命令
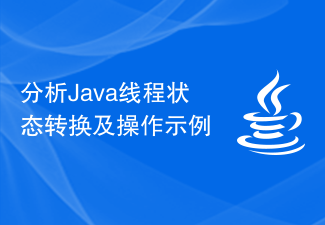
理解Java线程状态的变化与其对应的操作,需要具体代码示例在Java多线程编程中,线程的状态变化是非常重要的。了解线程的状态变化以及如何对线程进行操作,有助于我们更好地掌握多线程编程的核心概念。Java的线程状态可以分为6种:新建(New)、就绪(Runnable)、运行(Running)、阻塞(Blocked)、等待(Waiting)和终止(Termina
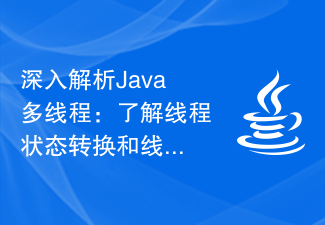
Java多线程原理解析:线程状态转换与线程间通信在Java中,多线程编程是一种常见的方法来实现并行计算和提高程序性能。多线程编程可以充分利用计算机的多核能力,使得程序能够同时执行多个任务。然而,要正确地编写多线程程序并保证其正确性和性能是一项相对复杂的任务。本文将解析Java多线程的原理,重点关注线程状态转换和线程间的通信。并且会提供具体的代码示例来阐述这些
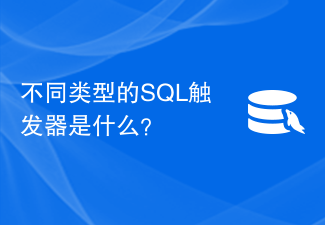
sql触发器有哪几种,需要具体代码示例。在SQL数据库中,触发器是一种特殊类型的存储过程,可以在数据库中的特定事件发生时自动执行。触发器通常用于实现数据完整性和业务逻辑约束。SQL触发器可以在数据插入、更新或删除时自动触发,执行一系列定义好的操作。SQL触发器可以分为以下几种类型:插入触发器(INSERTTrigger):当向表中插入新记录时触发。如下是一
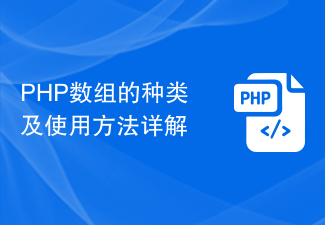
PHP数组的种类及使用方法详解PHP是一种广泛应用的服务器端脚本语言,具有强大的数组处理功能。数组在PHP中是一种非常重要的数据类型,能够有效地存储和管理大量数据。本文将对PHP数组的种类及使用方法进行详细解析,包括索引数组、关联数组、多维数组以及常用的数组操作方法,同时给出具体的代码示例。一、索引数组索引数组是PHP中最基础、常见的数组类型之一,其元素以数
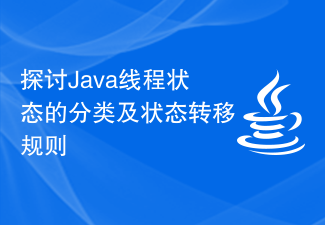
探究Java线程状态的种类和转换规则线程在Java中是一种执行单元,它可以独立运行并与其他线程并发执行。在Java中,线程具有多种状态,这些状态反映了线程在不同阶段的行为和条件。本文将探究Java线程的状态种类和转换规则,并提供具体的代码示例。Java线程状态的种类主要有以下几种:新建状态(New):线程被创建但尚未启动。运行状态(Runnable):线程被


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
