


Java线程状态转换的原理和应用场景
引言:
Java作为一种多线程编程语言,使用线程可以实现并发执行,提高程序的执行效率。在Java中,线程的状态是非常重要的概念,它决定了线程可以执行的操作和被操作的状态。本文将从原理和应用场景两个方面来分析Java线程状态转换。
一、线程状态转换的原理
在Java中,线程有六种状态:New、Runnable、Blocked、Waiting、Timed Waiting和Terminated。线程状态是通过调用不同方法来实现转换的。
- New状态:
当我们创建一个线程对象并调用start()方法时,线程处于New状态。处于New状态的线程还没有开始运行,它可以通过调用start()方法来启动。
代码示例:
Thread thread = new Thread(); thread.start();
- Runnable状态:
当线程被启动后,它会进入Runnable状态。处于Runnable状态的线程正在Java虚拟机中运行,它可能正在执行,也可能在等待某些资源。
代码示例:
public class MyThread implements Runnable{ public void run(){ // 线程执行的代码逻辑 } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); } }
- Blocked状态:
如果一个线程在等待获取锁,而其他线程已经获得了该锁,则该线程将进入Blocked状态。处于Blocked状态的线程将会等待其他线程释放锁,然后竞争获取锁。
代码示例:
public class MyThread implements Runnable{ public void run(){ synchronized (lock){ // 获取锁之后执行的代码逻辑 } } } public class Main{ public static void main(String[] args){ MyThread myThread1 = new MyThread(); MyThread myThread2 = new MyThread(); Thread thread1 = new Thread(myThread1); Thread thread2 = new Thread(myThread2); thread1.start(); thread2.start(); } }
- Waiting状态:
当线程等待某些条件满足时,它将进入Waiting状态。等待的条件可以是另一个线程的通知或者指定时间的到达。
代码示例:
public class MyThread implements Runnable{ public void run(){ synchronized (lock){ try{ lock.wait(); // 等待其他线程的通知 }catch(InterruptedException e){ e.printStackTrace(); } } } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); // 唤醒等待的线程 synchronized (lock){ lock.notify(); } } }
- Timed Waiting状态:
当线程在等待指定时间后还未能获取到锁或者其他条件未满足时,它将进入Timed Waiting状态。
代码示例:
public class MyThread implements Runnable{ public void run(){ try{ Thread.sleep(2000); // 等待2秒钟 }catch(InterruptedException e){ e.printStackTrace(); } } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); } }
- Terminated状态:
当线程的run()方法执行完毕时,线程将进入Terminated状态。处于Terminated状态的线程已经结束执行。
代码示例:
public class MyThread implements Runnable{ public void run(){ // 线程执行的代码逻辑 } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); try{ thread.join(); // 等待线程执行完成 }catch(InterruptedException e){ e.printStackTrace(); } } }
二、线程状态转换的应用场景
线程状态的转换在多线程编程中有着广泛的应用场景。根据线程状态的不同,我们可以实现不同的线程行为。
- 使用Blocked状态实现线程之间的互斥访问:
在某些应用场景中,我们需要保证多个线程对共享资源的互斥访问。我们可以利用Blocked状态来实现线程之间的互斥访问。
代码示例:
public class MyThread implements Runnable{ public void run(){ synchronized(lock){ // 代码操作 } } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread1 = new Thread(myThread); Thread thread2 = new Thread(myThread); thread1.start(); thread2.start(); } }
- 使用Waiting状态实现线程之间的协作:
在线程之间实现协作,需要在某个线程等待其他线程的通知后再继续执行。这时可以利用Waiting状态来实现线程之间的协作。
代码示例:
public class MyThread implements Runnable{ public void run(){ synchronized(lock){ try{ lock.wait(); // 等待其他线程的通知 }catch(InterruptedException e){ e.printStackTrace(); } // 继续执行 } } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); // 唤醒等待的线程 synchronized (lock){ lock.notify(); } } }
- 使用Timed Waiting状态实现定时任务的执行:
在某些场景中,我们需要定时地执行某些任务,这时可以利用Timed Waiting状态来实现定时执行。
代码示例:
public class MyThread implements Runnable{ public void run(){ try{ Thread.sleep(2000); // 等待2秒钟 }catch(InterruptedException e){ e.printStackTrace(); } // 执行定时任务 } } public class Main{ public static void main(String[] args){ MyThread myThread = new MyThread(); Thread thread = new Thread(myThread); thread.start(); } }
总结:
本文通过介绍Java线程状态转换的原理和应用场景,详细阐释了Java线程状态的转换过程,并给出了相应的代码示例。了解线程状态转换的原理和应用场景对于进行多线程编程非常重要,希望本文能够对读者有所帮助。
The above is the detailed content of In-depth discussion of the mechanism and scope of application of Java thread state changes. For more information, please follow other related articles on the PHP Chinese website!
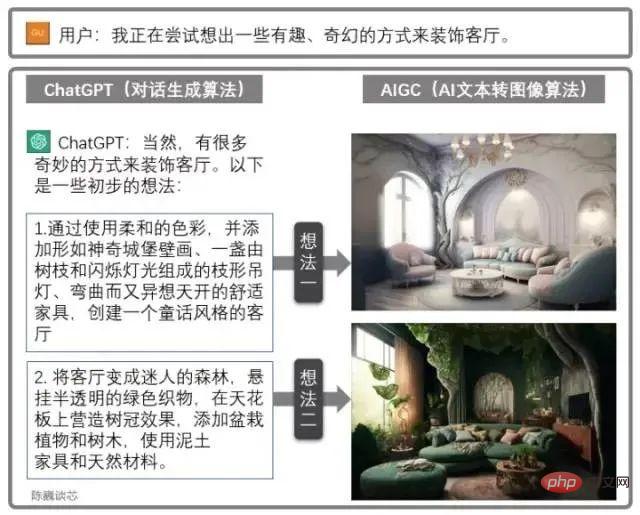
去年12月1日,OpenAI推出人工智能聊天原型ChatGPT,再次赚足眼球,为AI界引发了类似AIGC让艺术家失业的大讨论。ChatGPT是一种专注于对话生成的语言模型。它能够根据用户的文本输入,产生相应的智能回答。这个回答可以是简短的词语,也可以是长篇大论。其中GPT是GenerativePre-trainedTransformer(生成型预训练变换模型)的缩写。通过学习大量现成文本和对话集合(例如Wiki),ChatGPT能够像人类那样即时对话,流畅的回答各种问题。(当然回答速度比人还是
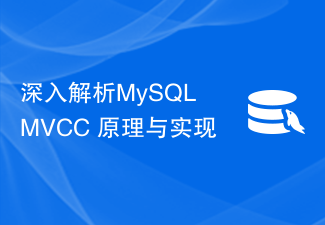
深入解析MySQLMVCC原理与实现MySQL是目前最流行的关系型数据库管理系统之一,它提供了多版本并发控制(MultiversionConcurrencyControl,MVCC)机制来支持高效并发处理。MVCC是一种在数据库中处理并发事务的方法,可以提供高并发和隔离性。本文将深入解析MySQLMVCC的原理与实现,并结合代码示例进行说明。一、M
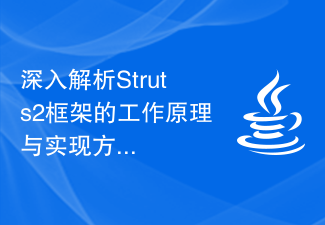
解读Struts2框架的原理及实现方式引言:Struts2作为一种流行的MVC(Model-View-Controller)框架,被广泛应用于JavaWeb开发中。它提供了一种将Web层与业务逻辑层分离的方式,并且具有灵活性和可扩展性。本文将介绍Struts2框架的基本原理和实现方式,同时提供一些具体的代码示例来帮助读者更好地理解该框架。一、框架原理:St
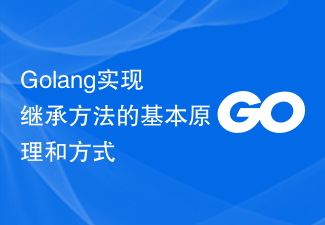
Golang继承方法的基本原理与实现方式在Golang中,继承是面向对象编程的重要特性之一。通过继承,我们可以使用父类的属性和方法,从而实现代码的复用和扩展性。本文将介绍Golang继承方法的基本原理和实现方式,并提供具体的代码示例。继承方法的基本原理在Golang中,继承是通过嵌入结构体的方式实现的。当一个结构体嵌入另一个结构体时,被嵌入的结构体就拥有了嵌
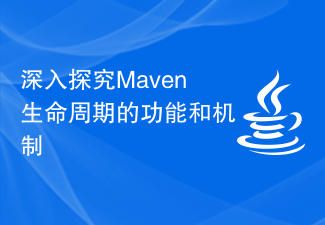
深入理解Maven生命周期的作用与原理Maven是一款非常流行的项目管理工具,它使用一种灵活的构建模型来管理项目的构建、测试和部署等任务。Maven的核心概念之一就是生命周期(Lifecycle),它定义了一系列阶段(Phase)和每个阶段的目标(Goal),帮助开发人员和构建工具按照预定的顺序执行相关操作。Maven的生命周期主要分为三套:Clean生命周
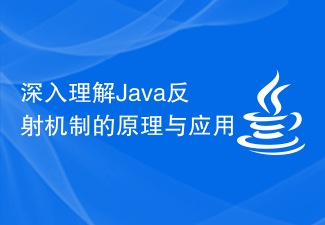
深入理解Java反射机制的原理与应用一、反射机制的概念与原理反射机制是指在程序运行时动态地获取类的信息、访问和操作类的成员(属性、方法、构造方法等)的能力。通过反射机制,我们可以在程序运行时动态地创建对象、调用方法和访问属性,而不需要在编译时知道类的具体信息。反射机制的核心是java.lang.reflect包中的类和接口。其中,Class类代表一个类的字节
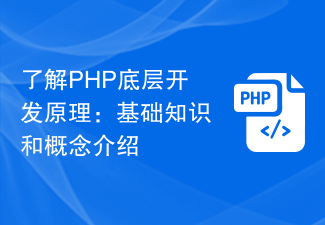
了解PHP底层开发原理:基础知识和概念介绍作为一名PHP开发者,了解PHP底层开发原理是非常重要的。正因为如此,本文将介绍PHP底层开发的基础知识和概念,帮助读者更好地理解和应用PHP。一、什么是PHP?PHP(全称:HypertextPreprocessor)是一门开源的脚本语言,主要用于Web开发。它可以嵌入到HTML文档中,通过服务器解释执行,并生成
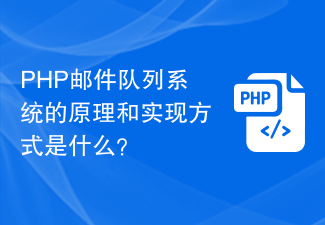
PHP邮件队列系统的原理和实现方式是什么?随着互联网的发展,电子邮件已经成为人们日常生活和工作中必不可少的通信方式之一。然而,随着业务的增长和用户数量的增加,直接发送电子邮件可能会导致服务器性能下降、邮件发送失败等问题。为了解决这个问题,可以使用邮件队列系统来通过串行队列的方式发送和管理电子邮件。邮件队列系统的实现原理如下:邮件入队列当需要发送邮件时,不再直


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
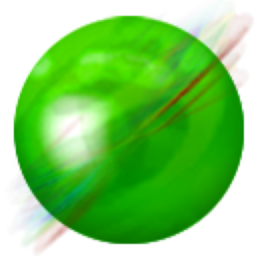
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
