Quickly master the installation method of Scipy library, you need specific code examples
Overview:
Scipy is a powerful Python scientific computing library for numerical calculations and statistics. Analysis, optimization, etc. provide rich functions. It is built on Numpy, so before using Scipy, you need to install the Numpy library. This article will introduce the installation method of Scipy in detail and provide specific code examples to help readers quickly master the installation and use of Scipy.
Installation steps:
-
Ensure that the Python environment is installed:
First, before installing Scipy, we need to ensure that the Python environment has been installed. You can enter the following command in the terminal (or command prompt) to check the installation of Python:python --version
If a message similar to "Python 3.7.2" is output, Python has been successfully installed.
-
Install Numpy library:
Scipy library is based on Numpy, so before installing Scipy, you need to install the Numpy library first. You can use the following command to install Numpy:pip install numpy
-
Install the Scipy library:
After installing Numpy, we can install the Scipy library. Scipy can be installed using the following command:pip install scipy
Code sample:
Below we will demonstrate how to use some common functions in the Scipy library to help readers better understand Scipy Instructions.
-
Integral function (integrate) example:
The integral function in the Scipy library can be used to solve the integral of a one-variable or multi-variable function. The following is an example code that calculates the integral value of a function over a specified interval:import numpy as np from scipy import integrate def f(x): return np.sin(x) result, error = integrate.quad(f, 0, np.pi) # 计算 sin(x) 在 0 到 pi 的积分 print("结果:", result) print("误差:", error)
-
Linear algebra function (linalg) example:
The linear algebra function in the Scipy library is provided Functions such as matrix operations and linear equation solving. The following is an example code to solve a system of linear equations:import numpy as np from scipy import linalg A = np.array([[1, 2], [3, 4]]) # 系数矩阵 b = np.array([5, 6]) # 常数矩阵 x = linalg.solve(A, b) # 求解 Ax = b 的解 print("解:", x)
-
Interpolation function (interpolate) example:
The interpolation function in the Scipy library can be used to generate a curve interpolation. The following is a sample code that generates an interpolation curve of a sin function and draws a graph:import numpy as np from scipy import interpolate import matplotlib.pyplot as plt x = np.linspace(0, 2 * np.pi, 10) # 生成 0 到 2π 的等间距数据 y = np.sin(x) # 对应的sin函数值 f = interpolate.interp1d(x, y) # 生成插值函数 x_new = np.linspace(0, 2 * np.pi, 100) # 生成更多的数据点 y_new = f(x_new) # 对应的插值函数值 plt.plot(x, y, 'o', label='原始数据') plt.plot(x_new, y_new, label='插值曲线') plt.legend() plt.show()
Conclusion:
This article introduces the installation method of the Scipy library, with specific code Example. By studying these sample codes, readers can quickly master the basic usage of Scipy and start applying the Scipy library in fields such as data analysis, scientific computing, and machine learning. I hope this article can be helpful to readers and provide guidance for future study and practice.
The above is the detailed content of A simple guide to installing Scipy library. For more information, please follow other related articles on the PHP Chinese website!
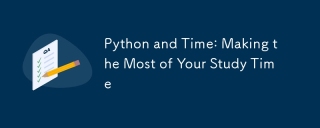
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
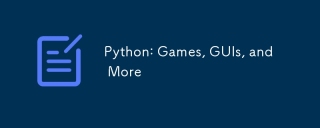
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
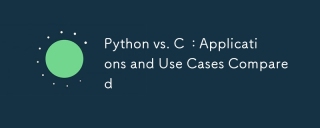
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
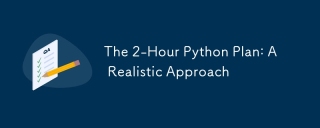
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
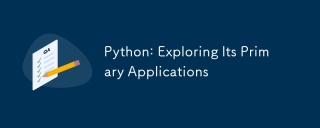
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
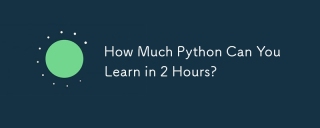
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
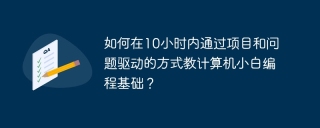
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
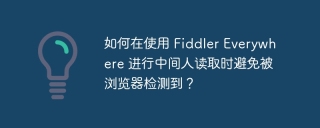
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!
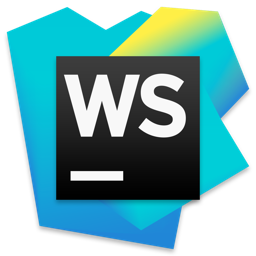
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment