Detailed explanation of Linux device model (4)_sysfs
1 Introduction
sysfs is a RAM-based file system that is used in conjunction with Kobject to export Kernel data structures and attributes to user space and provide access support for these data structures in the form of file directory structures.
sysfs has all the attributes of a file system, but this article focuses on its characteristics in the Linux device model. Therefore, we will not cover too many file system implementation details, but only introduce the role and use of sysfs in the device model. Specifically include:
- The relationship between sysfs and Kobject
- The concept of attribute
- File system operation interface of sysfs
2. The relationship between sysfs and Kobject
In the article "Linux Device Model_Kobject", it is mentioned that each Kobject corresponds to a directory in sysfs. Therefore, when adding Kobject to the Kernel, the create_dir interface will call the create directory interface of the sysfs file system to create a directory corresponding to the Kobject. The relevant code is as follows:
1: /* lib/kobject.c, line 47 */ 2: static int create_dir(struct kobject *kobj) 3: { 4: int error = 0; 5: error = sysfs_create_dir(kobj); 6: if (!error) { 7: error = populate_dir(kobj); 8: if (error) 9: sysfs_remove_dir(kobj); 10: } 11: return error; 12: } 13: 14: /* fs/sysfs/dir.c, line 736 */ 15: ** 16: * sysfs_create_dir - create a directory for an object. 17: * @kobj: object we're creating directory for. 18: */ 19: int sysfs_create_dir(struct kobject * kobj) 20: { 21: enum kobj_ns_type type; 22: struct sysfs_dirent *parent_sd, *sd; 23: const void *ns = NULL; 24: int error = 0; 25: ... 26: }
3. attribute
3.1 Function overview of attribute
In sysfs, why is there the concept of attribute? In fact, it corresponds to kobject, referring to the "attributes" of kobject. we know,
The directory in sysfs describes kobject, and kobject is the embodiment of a specific data type variable (such as struct device). Therefore, the attributes of kobject are the attributes of these variables. It can be anything, a name, an internal variable, a string, etc. Attributes are provided in the form of files in the sysfs file system, that is, all attributes of a kobject are presented in the form of files in its corresponding sysfs directory. These files are generally readable and writable, and the modules in the kernel that define these attributes will record and return the values of these attributes based on the read and write operations in user space.
To summarize: the so-called attibute is a method for information interaction between kernel space and user space. For example, if a driver defines a variable and hopes that the user space program can modify the variable to control the driver's running behavior, the variable can be opened as a sysfs attribute.
In the Linux kernel, attributes are divided into ordinary attributes and binary attributes, as follows:
1: /* include/linux/sysfs.h, line 26 */ 2: struct attribute { 3: const char *name; 4: umode_t mode; 5: #ifdef CONFIG_DEBUG_LOCK_ALLOC 6: bool ignore_lockdep:1; 7: struct lock_class_key *key; 8: struct lock_class_key skey; 9: #endif 10: }; 11: 12: /* include/linux/sysfs.h, line 100 */ 13: struct bin_attribute { 14: struct attribute attr; 15: size_t size; 16: void *private; 17: ssize_t (*read)(struct file *, struct kobject *, struct bin_attribute *, 18: char *, loff_t, size_t); 19: ssize_t (*write)(struct file *,struct kobject *, struct bin_attribute *, 20: char *, loff_t, size_t); 21: int (*mmap)(struct file *, struct kobject *, struct bin_attribute *attr, 22: struct vm_area_struct *vma); 23: };
The struct attribute is an ordinary attribute. The sysfs file generated using this attribute can only be read and written in the form of a string (I will explain why later). On the basis of struct attribute, struct bin_attribute adds read, write and other functions, so the sysfs file it generates can be read and written in any way.
After talking about the basic concepts, we have to ask two questions:
How does Kernel turn attributes into files in sysfs?
How to pass the read and write operations on sysfs files in user space to Kernel?
Let’s take a look at the process.
3.2 Creation of attibute file
In the Linux kernel, the creation of the attribute file is completed by the sysfs_create_file interface in fs/sysfs/file.c. There is nothing special about the implementation of this interface. Most of them are file system-related operations and have nothing to do with the device model. Many relationships will be omitted here.
3.3 Read and write of attibute files
When we see the prototype of struct attribute in Chapter 3.1, we may mutter that the structure is very simple. name represents the file name, mode represents the file mode, and other fields are used by the kernel to debug Kernel Lock. Then the file Where is the interface for operation?
Don’t worry, let’s go to the fs/sysfs directory to look at the sysfs-related code logic.
All file systems will define a struct file_operations variable to describe the operation interface of this file system, and sysfs is no exception:
1: /* fs/sysfs/file.c, line 472 */ 2: const struct file_operations sysfs_file_operations = { 3: .read = sysfs_read_file, 4: .write = sysfs_write_file, 5: .llseek = generic_file_llseek, 6: .open = sysfs_open_file, 7: .release = sysfs_release, 8: .poll = sysfs_poll, 9: };
The read operation of the attribute file will be transferred from VFS to the read (that is, sysfs_read_file) interface of sysfs_file_operations. Let us take a rough look at the processing logic of this interface.
1: /* fs/sysfs/file.c, line 127 */ 2: static ssize_t 3: sysfs_read_file(struct file *file, char __user *buf, size_t count, loff_t *ppos) 4: { 5: struct sysfs_buffer * buffer = file->private_data; 6: ssize_t retval = 0; 7: 8: mutex_lock(&buffer->mutex); 9: if (buffer->needs_read_fill || *ppos == 0) { 10: retval = fill_read_buffer(file->f_path.dentry,buffer); 11: if (retval) 12: goto out; 13: } 14: ... 15: } 16: /* fs/sysfs/file.c, line 67 */ 17: static int fill_read_buffer(struct dentry * dentry, struct sysfs_buffer * buffer) 18: { 19: struct sysfs_dirent *attr_sd = dentry->d_fsdata; 20: struct kobject *kobj = attr_sd->s_parent->s_dir.kobj; 21: const struct sysfs_ops * ops = buffer->ops; 22: ... 23: count = ops->show(kobj, attr_sd->s_attr.attr, buffer->page); 24: ... 25: }
“
read处理看着很简单,sysfs_read_file从file指针中取一个私有指针(注:大家可以稍微留一下心,私有数据的概念,在VFS中使用是非常普遍的),转换为一个struct sysfs_buffer类型的指针,以此为参数(buffer),转身就调用fill_read_buffer接口。
而fill_read_buffer接口,直接从buffer指针中取出一个struct sysfs_ops指针,调用该指针的show函数,即完成了文件的read操作。
那么后续呢?当然是由ops->show接口接着处理咯。而具体怎么处理,就是其它模块(例如某个driver)的事了,sysfs不再关心(其实,Linux大多的核心代码,都是只提供架构和机制,具体的实现,也就是苦力,留给那些码农吧!这就是设计的魅力)。
不过还没完,这个struct sysfs_ops指针哪来的?好吧,我们再看看open(sysfs_open_file)接口吧。
”
1: /* fs/sysfs/file.c, line 326 */ 2: static int sysfs_open_file(struct inode *inode, struct file *file) 3: { 4: struct sysfs_dirent *attr_sd = file->f_path.dentry->d_fsdata; 5: struct kobject *kobj = attr_sd->s_parent->s_dir.kobj; 6: struct sysfs_buffer *buffer; 7: const struct sysfs_ops *ops; 8: int error = -EACCES; 9: 10: /* need attr_sd for attr and ops, its parent for kobj */ 11: if (!sysfs_get_active(attr_sd)) 12: return -ENODEV; 13: 14: /* every kobject with an attribute needs a ktype assigned */ 15: if (kobj->ktype && kobj->ktype->sysfs_ops) 16: ops = kobj->ktype->sysfs_ops; 17: else { 18: WARN(1, KERN_ERR "missing sysfs attribute operations for " 19: "kobject: %s\n", kobject_name(kobj)); 20: goto err_out; 21: } 22: 23: ... 24: 25: buffer = kzalloc(sizeof(struct sysfs_buffer), GFP_KERNEL); 26: if (!buffer) 27: goto err_out; 28: 29: mutex_init(&buffer->mutex); 30: buffer->needs_read_fill = 1; 31: buffer->ops = ops; 32: file->private_data = buffer; 33: ... 34: }
“
哦,原来和ktype有关系。这个指针是从该attribute所从属的kobject中拿的。再去看一下”Linux设备模型_Kobject”中ktype的定义,还真有一个struct sysfs_ops的指针。
我们注意一下14行的注释以及其后代码逻辑,如果从属的kobject(就是attribute文件所在的目录)没有ktype,或者没有ktype->sysfs_ops指针,是不允许它注册任何attribute的!
经过确认后,sysfs_open_file从ktype中取出struct sysfs_ops指针,并在随后的代码逻辑中,分配一个struct sysfs_buffer类型的指针(buffer),并把struct sysfs_ops指针保存在其中,随后(注意哦),把buffer指针交给file的private_data,随后read/write等接口便可以取出使用。嗯!惯用伎俩!
”
顺便看一下struct sysfs_ops吧,我想你已经能够猜到了。
1: /* include/linux/sysfs.h, line 124 */ 2: struct sysfs_ops { 3: ssize_t (*show)(struct kobject *, struct attribute *,char *); 4: ssize_t (*store)(struct kobject *,struct attribute *,const char *, size_t); 5: const void *(*namespace)(struct kobject *, const struct attribute *); 6: };
attribute文件的write过程和read类似,这里就不再多说。另外,上面只分析了普通attribute的逻辑,而二进制类型的呢?也类似,去看看fs/sysfs/bin.c吧,这里也不说了。
讲到这里,应该已经结束了,事实却不是如此。上面read/write的数据流,只到kobject(也就是目录)级别哦,而真正需要操作的是attribute(文件)啊!这中间一定还有一层转换!确实,不过又交给其它模块了。 下面我们通过一个例子,来说明如何转换的。
4. sysfs在设备模型中的应用总结
让我们通过设备模型class.c中有关sysfs的实现,来总结一下sysfs的应用方式。
首先,在class.c中,定义了Class所需的ktype以及sysfs_ops类型的变量,如下:
1: /* drivers/base/class.c, line 86 */ 2: static const struct sysfs_ops class_sysfs_ops = { 3: .show = class_attr_show, 4: .store = class_attr_store, 5: .namespace = class_attr_namespace, 6: }; 7: 8: static struct kobj_type class_ktype = { 9: .sysfs_ops = &class_sysfs_ops, 10: .release = class_release, 11: .child_ns_type = class_child_ns_type, 12: };
由前面章节的描述可知,所有class_type的Kobject下面的attribute文件的读写操作,都会交给class_attr_show和class_attr_store两个接口处理。以class_attr_show为例:
1: /* drivers/base/class.c, line 24 */ 2: #define to_class_attr(_attr) container_of(_attr, struct class_attribute, attr) 3: 4: static ssize_t class_attr_show(struct kobject *kobj, struct attribute *attr, 5: char *buf) 6: { 7: struct class_attribute *class_attr = to_class_attr(attr); 8: struct subsys_private *cp = to_subsys_private(kobj); 9: ssize_t ret = -EIO; 10: 11: if (class_attr->show) 12: ret = class_attr->show(cp->class, class_attr, buf); 13: return ret; 14: }
该接口使用container_of从struct attribute类型的指针中取得一个class模块的自定义指针:struct class_attribute,该指针中包含了class模块自身的show和store接口。下面是struct class_attribute的声明:
1: /* include/linux/device.h, line 399 */ 2: struct class_attribute { 3: struct attribute attr; 4: ssize_t (*show)(struct class *class, struct class_attribute *attr, 5: char *buf); 6: ssize_t (*store)(struct class *class, struct class_attribute *attr, 7: const char *buf, size_t count); 8: const void *(*namespace)(struct class *class, 9: const struct class_attribute *attr); 10: };
因此,所有需要使用attribute的模块,都不会直接定义struct attribute变量,而是通过一个自定义的数据结构,该数据结构的一个成员是struct attribute类型的变量,并提供show和store回调函数。然后在该模块ktype所对应的struct sysfs_ops变量中,实现该本模块整体的show和store函数,并在被调用时,转接到自定义数据结构(struct class_attribute)
The above is the detailed content of Detailed explanation of Linux device model (4)_sysfs. For more information, please follow other related articles on the PHP Chinese website!
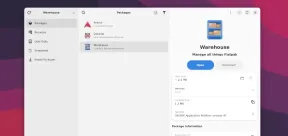
A GUI for Effortless Flatpak Management: Introducing Warehouse Managing a growing collection of Flatpak applications can be cumbersome using only the command line. Enter Warehouse, a user-friendly graphical interface designed to streamline Flatpak a
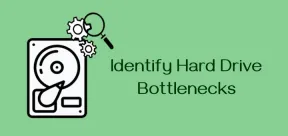
This article provides a comprehensive guide to identifying and resolving hard drive bottlenecks in Linux systems. Experienced server administrators will find this particularly useful. Slow disk operations can severely impact application performance,
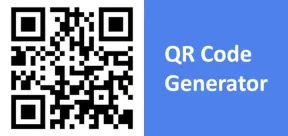
Efficient QR code generation tool under Linux system In today's digital world, QR codes have become a way to quickly and conveniently share information, simplifying data access from URLs, texts, contacts, Wi-Fi credentials, and even payment information. Linux users can use a variety of tools to create QR codes efficiently. Let's take a look at some popular QR code generators that can be used directly on Linux systems. QRencode QRencode is a lightweight command line tool for generating QR codes on Linux. It is well-received for its simplicity and efficiency and is popular with Linux users who prefer direct methods. Using QRencode, you can use the URL,
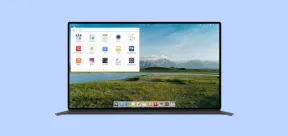
Elementary OS 8 Circe: A Smooth and Stylish Linux Experience Elementary OS, a Ubuntu-based Linux distribution, has evolved from a simple theme pack into a fully-fledged, independent operating system. Known for its user-friendly interface, elegant de
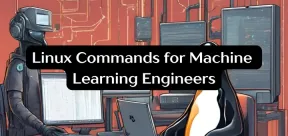
Mastering Linux is crucial for any machine learning (ML) engineer. Its command-line interface offers unparalleled flexibility and control, streamlining workflows and boosting productivity. This article outlines essential Linux commands, explained fo
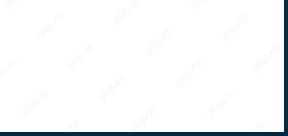
Arch Linux: A Beginner's Command-Line Cheat Sheet Arch Linux offers unparalleled control but can feel daunting for newcomers. This cheat sheet provides essential commands to confidently manage your system. System Information & Updates These com
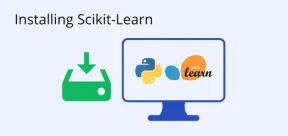
This guide provides a comprehensive walkthrough of installing and using the Scikit-learn machine learning library on Linux systems. Scikit-learn (sklearn) is a powerful, open-source Python library offering a wide array of tools for various machine l
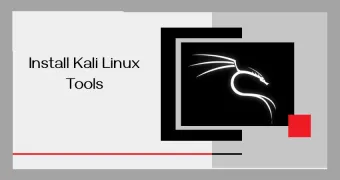
This guide explains how to leverage Docker for accessing Kali Linux tools, a safer and more efficient alternative to outdated methods like Katoolin. Katoolin is no longer actively maintained and may cause compatibility problems on modern systems. Do


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
