php editor Banana introduces you to a common problem: the form cannot be serialized to json correctly. In development, we often need to pass form data to the backend for processing in json format. However, sometimes we encounter some problems, such as the submitted data cannot be correctly converted into json format. This may be caused by special characters in the form or incorrect formatting. In this article, we will explore some common causes and solutions to help you solve this problem and ensure that form data is serialized to json correctly.
Question content
I'm trying to create a web application in golang that allows you to enter receipt details into different forms, and then these form inputs are serialized into json objects . However, I'm having trouble serializing the form because every time I try to "submit" the receipt, I get an error message.
This is main.go
package main import ( "encoding/json" "html/template" "log" "net/http" "strconv" "github.com/gorilla/mux" ) type item struct { shortdescription string `json:"shortdescription"` price string `json:"price"` } type receipt struct { retailer string `json:"retailer"` purchasedate string `json:"purchasedate"` purchasetime string `json:"purchasetime"` items []item `json:"items"` total string `json:"total"` receiptid int `json:"receiptid"` } var receiptidcounter int var receipts = make(map[int]receipt) func main() { r := mux.newrouter() r.handlefunc("/", homehandler).methods("get") r.handlefunc("/submit", submithandler).methods("post") r.handlefunc("/receipt/{id}", receipthandler).methods("get") http.handle("/", r) log.fatal(http.listenandserve(":8080", nil)) } func homehandler(w http.responsewriter, r *http.request) { t, err := template.parsefiles("templates/home.html") if err != nil { log.println(err) http.error(w, "internal server error", http.statusinternalservererror) return } err = t.execute(w, nil) if err != nil { log.println(err) http.error(w, "internal server error", http.statusinternalservererror) } } func submithandler(w http.responsewriter, r *http.request) { decoder := json.newdecoder(r.body) var receipt receipt err := decoder.decode(&receipt) if err != nil { log.println(err) http.error(w, "bad request", http.statusbadrequest) return } receiptidcounter++ receipt.receiptid = receiptidcounter receipts[receipt.receiptid] = receipt jsonresponse, err := json.marshal(map[string]int{"receiptid": receipt.receiptid}) if err != nil { log.println(err) http.error(w, "internal server error", http.statusinternalservererror) return } w.header().set("content-type", "application/json") w.write(jsonresponse) } func receipthandler(w http.responsewriter, r *http.request) { vars := mux.vars(r) id, err := strconv.atoi(vars["id"]) if err != nil { log.println(err) http.error(w, "bad request", http.statusbadrequest) return } receipt, exists := receipts[id] if !exists { http.notfound(w, r) return } t, err := template.parsefiles("templates/receipt.html") if err != nil { log.println(err) http.error(w, "internal server error", http.statusinternalservererror) return } err = t.execute(w, receipt) if err != nil { log.println(err) http.error(w, "internal server error", http.statusinternalservererror) } }
This is my home.html, this is the html of my homepage
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>receipt input form</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1 id="receipt-input-form">receipt input form</h1> <form id="receipt-form"> <label>retailer:</label> <input type="text" name="retailer" required><br><br> <label>purchase date:</label> <input type="date" name="purchasedate" required><br><br> <label>purchase time:</label> <input type="time" name="purchasetime" required><br><br> <div id="items"> <div class="item"> <label>short description:</label> <input type="text" name="shortdescription[]" required> <label>price:</label> <input type="number" name="price[]" step="0.01" min="0" required> </div> </div> <button type="button" id="add-item-btn">add item</button><br><br> <label>total:</label> <input type="number" name="total" step="0.01" min="0" required><br><br> <button type="submit">submit</button> </form> <script> $(document).ready(function() { var itemcount = 1; $('#add-item-btn').click(function() { itemcount++; var newitem = '<div class="item"><label>short description:</label>' + '<input type="text" name="shortdescription[]" required>' + '<label>price:</label>' + '<input type="number" name="price[]" step="0.01" min="0" required>' + '<button type="button" class="remove-item-btn">remove item</button>' + '</div>'; $('#items').append(newitem); }); $(document).on('click', '.remove-item-btn', function() { $(this).parent().remove(); itemcount--; }); $('#receipt-form').submit(function(event) { event.preventdefault(); var form = $(this).serializearray(); var items = []; $('.item').each(function() { var item = {}; item.shortdescription = $(this).find('input[name="shortdescription[]"]').val(); item.price = $(this).find('input[name="price[]"]').val(); items.push(item); }); form.push({ name: "items", value: json.stringify(items) }); $.ajax({ type: "post", url: "/submit", data: form, success: function(response) { window.location.href = "/receipt?id=" + response.receiptid; }, error: function(xhr, status, error) { console.log(xhr.responsetext); } }); }); }); </script> </body> </html>
This is my receipt.html, this is the html of the receipt page after submitting the receipt.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Receipt Details</title> </head> <body> <h1 id="Receipt-Details">Receipt Details</h1> <ul> <li>Retailer: {{.Retailer}}</li> <li>Purchase Date: {{.PurchaseDate}}</li> <li>Purchase Time: {{.PurchaseTime}}</li> <li>Items:</li> <ul> {{range .Items}} <li>{{.ShortDescription}} - {{.Price}}</li> {{end}} </ul> <li>Total: {{.Total}}</li> </ul> </body> </html>
I tried different serialization methods but nothing worked. When I fill out the receipt form and click submit, I expect that I will be taken to the receipt page that displays the unique details of that receipt. But I just got an error, my most recent error was this:
inInvalid character "r" looking for beginning of value
Workaround
Please update your home.html
as follows. I changed the submit request content type to application/json
because the submithandler
in the server is looking for json
.
$('#receipt-form').submit(function(event) { event.preventDefault(); var form = $(this).serializeArray(); var formObject = {}; $.each(form, function(i, v) { if (v.name != "price[]" && v.name != "shortDescription[]") { formObject[v.name] = v.value; } }); var items = []; $('.item').each(function() { var item = {}; item.shortDescription = $(this).find('input[name="shortDescription[]"]').val(); item.price = $(this).find('input[name="price[]"]').val(); items.push(item); }); formObject["items"] = items; $.ajax({ type: "POST", url: "/submit", contentType: "application/json; charset=utf-8", dataType: "json", data: JSON.stringify(formObject), success: function(response) { window.location.href = "/receipt?id=" + response.receiptID; }, error: function(xhr, status, error) { console.log(xhr.responseText); } }); });
The above is the detailed content of Unable to properly serialize form to json. For more information, please follow other related articles on the PHP Chinese website!
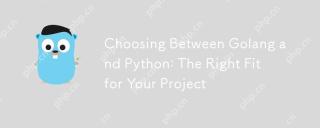
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
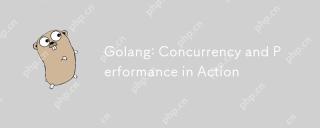
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
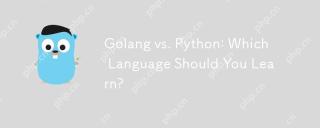
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
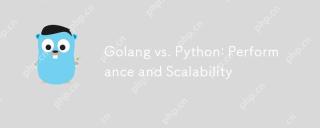
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
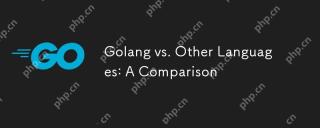
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
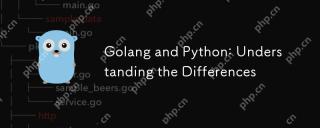
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
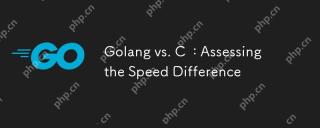
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
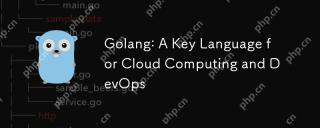
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
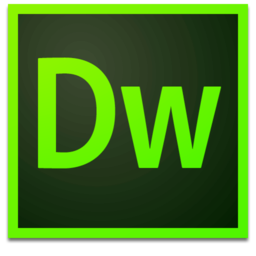
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
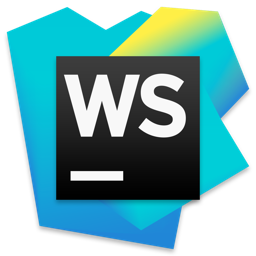
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.