In Go language, creating a CSR (Certificate Signing Request) with a specific subject order is an important task. A CSR is a file used to apply for a digital certificate from a Certificate Authority (CA). By creating the CSR correctly, we can ensure that the subjects in the certificate (such as domain name, organization name, etc.) are ordered according to our requirements. In Go language, we can use x509 package to create and process CSR. In this article, php editor Xiaoxin will introduce you to the detailed steps to help you easily create a CSR with a specific theme order in Go.
Question content
I'm trying to create a certificate signing request in go using the cryptographic library. The CSR generated by has the subject "Subject: C = IN, L = loc, O = Example Org, OU = OU1 OU = OU2, CN = example.com". I want to change the order of the topics to "Topics: C=IN, O=Example Org, OU=OU1 OU=OU2, L=loc, CN=example.com".
I generated the CSR using the following code.
package main import ( "crypto/rand" "crypto/rsa" "crypto/x509" "crypto/x509/pkix" "encoding/pem" "fmt" "os" ) func main() { privKey, err := rsa.GenerateKey(rand.Reader, 2048) if err != nil { fmt.Println(err) os.Exit(1) } csrTemplate := x509.CertificateRequest{ Subject: pkix.Name{ Country: []string{"IN"}, Organization: []string{"Example Org"}, OrganizationalUnit: []string{"OU1", "OU2"}, Locality: []string{"loc"}, CommonName: "example.com", }, EmailAddresses: []string{"[email protected]"}, } csrBytes, err := x509.CreateCertificateRequest(rand.Reader, &csrTemplate, privKey) if err != nil { fmt.Println(err) os.Exit(1) } csrPem := pem.EncodeToMemory(&pem.Block{ Type: "CERTIFICATE REQUEST", Bytes: csrBytes, }) fmt.Println(string(csrPem)) }
This code generates a CSR with the subject "Subject: C = IN, L = loc, O = Example Organization, OU = OU1 OU = OU2, CN = example.com". I can generate a CSR
with the desired topic order using the openssl command belowopenssl req -new -sha256 -key my-private-key.pem -out my-csr1.pem -subj '/C=IN/O=Org/OU=OU1/OU=OU2/L=loc/CN=example.com'
How to do the same thing in Go?
Workaround
I'm not sure why you want to put the RDNs in the topic in this specific order. In my opinion, any software that relies on a specific order is somewhat broken. Of course, broken software exists, and sometimes the only way is to fix it.
There is also a way to do this using golang. But you can't simply use the matching field names in pkix.Name because those names will be serialized in a fixed order. To get your own order you need to use ExtraNames
and then provide the RDNs in the order you need:
var ( oidCountry = []int{2, 5, 4, 6} oidOrganization = []int{2, 5, 4, 10} oidOrganizationalUnit = []int{2, 5, 4, 11} oidCommonName = []int{2, 5, 4, 3} oidLocality = []int{2, 5, 4, 7} ) csrTemplate := x509.CertificateRequest{ Subject: pkix.Name{ ExtraNames: []pkix.AttributeTypeAndValue{ { oidCountry, "IN" }, { oidOrganization, "Example Org" }, { oidOrganizationalUnit, "OU1" }, { oidOrganizationalUnit, "OU2" }, { oidLocality, "loc" }, { oidCommonName, "example.com" }, }, }, EmailAddresses: []string{"<a href="https://www.php.cn/link/89fee0513b6668e555959f5dc23238e9" class="__cf_email__" data-cfemail="4b3f2e383f0b2e332a263b272e65282426">[email protected]</a>"}, }
The above is the detailed content of Create CSR with specific topic order in go. For more information, please follow other related articles on the PHP Chinese website!
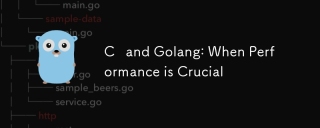
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
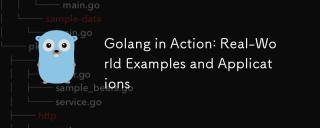
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
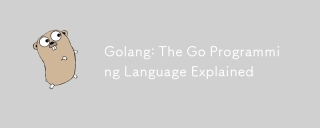
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
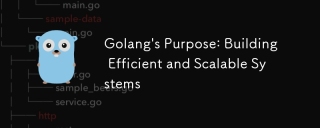
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
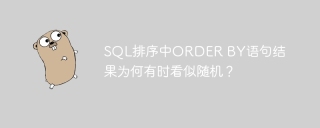
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
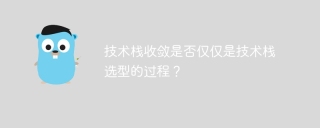
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
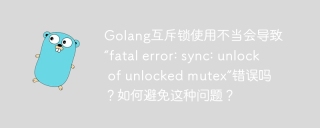
Golang ...
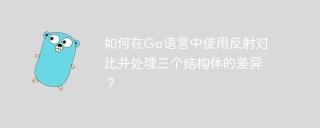
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
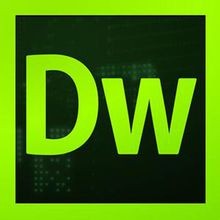
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),