Extract memory and swap information from /proc/meminfo in Golang
php editor Strawberry will introduce to you today a very practical method, which is to extract memory and exchange information from /proc/meminfo in Golang. During the Golang development process, we often need to obtain the system's memory and exchange information in order to perform some performance optimization or resource management operations. /proc/meminfo is a file that saves system memory and swap information. We can obtain the required information by reading this file. Next, I will introduce in detail how to use Golang to extract the memory and swap information in /proc/meminfo. I hope it will be helpful to you.
Question content
I want to extract the values of memtotal, memfree, memavailable, swaptotal and swapfree from /proc/meminfo in golang. The closest I've gotten so far is using fmt.sscanf() which will give me the value I want one at a time, but I also get a lot of output lines with zeros. This is the code I'm using:
package main import ( "bufio" "fmt" "os" ) func main() { f, e := os.open("/proc/meminfo") if e != nil { panic(e) } defer f.close() s := bufio.newscanner(f) for s.scan() { var n int fmt.sscanf(s.text(), "memfree: %d kb", &n) fmt.println(n) } }
This gives me the following results:
0 11260616 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
So first question, is there a way to limit the results to the one value I want (non-zero)? Or, is there a better way to solve this problem?
My /proc/meminfo file looks like this:
MemTotal: 16314336 kB MemFree: 11268004 kB MemAvailable: 13955820 kB Buffers: 330284 kB Cached: 2536848 kB SwapCached: 0 kB Active: 1259348 kB Inactive: 3183140 kB Active(anon): 4272 kB Inactive(anon): 1578028 kB Active(file): 1255076 kB Inactive(file): 1605112 kB Unevictable: 0 kB Mlocked: 0 kB SwapTotal: 4194304 kB SwapFree: 4194304 kB Dirty: 96 kB Writeback: 0 kB AnonPages: 1411704 kB Mapped: 594408 kB Shmem: 6940 kB KReclaimable: 151936 kB Slab: 253384 kB SReclaimable: 151936 kB SUnreclaim: 101448 kB KernelStack: 17184 kB PageTables: 25060 kB NFS_Unstable: 0 kB Bounce: 0 kB WritebackTmp: 0 kB CommitLimit: 12351472 kB Committed_AS: 6092984 kB VmallocTotal: 34359738367 kB VmallocUsed: 40828 kB VmallocChunk: 0 kB Percpu: 5696 kB AnonHugePages: 720896 kB ShmemHugePages: 0 kB ShmemPmdMapped: 0 kB FileHugePages: 0 kB FilePmdMapped: 0 kB HugePages_Total: 0 HugePages_Free: 0 HugePages_Rsvd: 0 HugePages_Surp: 0 Hugepagesize: 2048 kB Hugetlb: 0 kB DirectMap4k: 230400 kB DirectMap2M: 11235328 kB DirectMap1G: 14680064 kB
Solution
Note that s.Scan()
reads the input line by line. If a line does not match the format string given to fmt.Sscanf
, the program will output 0 as var n int
declared inside the loop. My suggestion is to check the first result returned by fmt.Sscanf`, which is the number of matching items. So if the first result is 1, that means there is a match and that value can be output. See a working example here: https://www.php.cn/link/25d0a45ccd9e33b6b1ef8760801b6841 p>
Edit: I tried to get as close to your code as possible. There may be other issues as the measurement units used may vary according to the Man Page. However, if the relevant value on your system is always output in "kB", then it may be enough for your use case.
The above is the detailed content of Extract memory and swap information from /proc/meminfo in Golang. For more information, please follow other related articles on the PHP Chinese website!
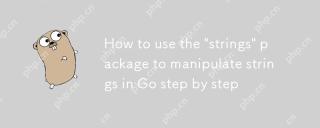
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
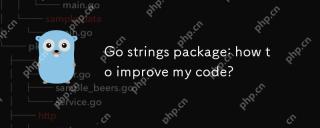
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
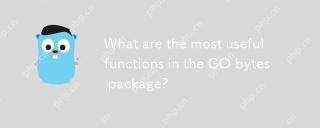
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
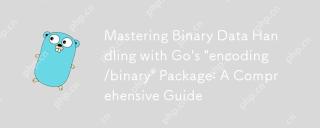
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
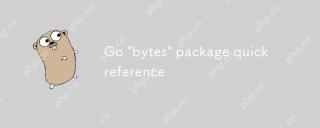
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
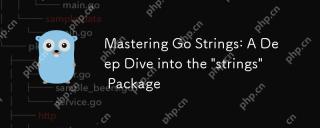
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
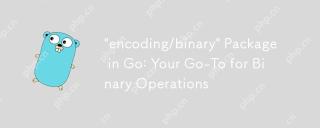
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
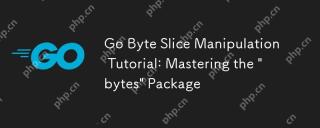
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
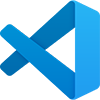
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
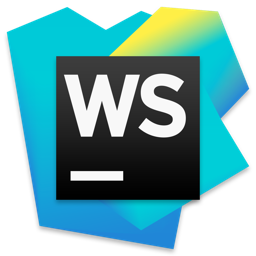
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
