php editor Strawberry will introduce to you a commonly used technique today - Go. This is an idiomatic way of mapping one structure to another. In programming, we often need to convert one data structure into another data structure to meet different needs. Go provides a concise and flexible way to achieve this goal. Whether processing database query results, API responses, or other data transformation scenarios, Go can help us easily complete structure mapping work. Next, let us learn about the specific usage of Go!
Question content
I am using a third-party go language library to query the user's ldap database. The library returns a resultuser slice of searchresult which I need to map to my own user structure. These two structures have different field names, I only need specific fields from resultuser. Is there a more idiomatic way in go to convert one struct to another.
I created a demo below (also linked on the go playground). Thank you in advance for any advice you may have for this go newbie!
package main import "fmt" type ( User struct { id int32 firstName string } ResultUser struct { uid int32 fname string } SearchResults []ResultUser ) func main() { results := getSearchResults() users := mapResultsToUsers(results) // <-- This is the problem fmt.Println("User struct:", users[0].id, users[0].firstName) fmt.Println("User struct:", users[1].id, users[1].firstName) } // Simulates a query to a data with a library func getSearchResults() (results SearchResults) { return append(results, ResultUser{1, "John"}, ResultUser{2, "Jane"}) } // Seems like a code smell to have to do this // Is there a more idiomatic way to do this? func mapResultsToUsers(results SearchResults) (users []User) { for _, result := range results { users = append(users, User{result.uid, result.fname}) } return users }
I've seen struct field tags but not sure if there's a better way.
Workaround
I think what you have is pretty much the best solution, although I would move the mapping into a dedicated function, like:
func fromresultuser(r *resultuser) *user { return &user{ id: r.uid, firstname: r.fname, } }
Then mapresultstousers
becomes:
func mapresultstousers(results searchresults) (users []*user) { for _, result := range results { users = append(users, fromresultuser(result)) } return users }
I've seen struct field tags but not sure if there's a better way.
You can combine something so that you can annotate your user
structure like this:
User struct { id int32 `mappedFrom:"uid"` firstName string `mappedFrom:"fname"` }
But implementing this requires a much more complex approach than fromresultuser
presented here, and requires familiarity with the reflect
package. I think, as one of my colleagues likes to say, "the juice isn't worth the squeeze."
The above is the detailed content of Go - Idiomatic way of mapping one struct to another. For more information, please follow other related articles on the PHP Chinese website!
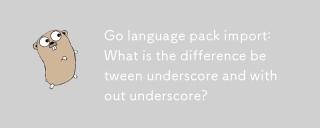
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
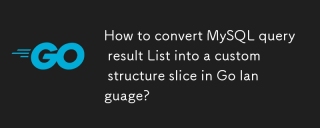
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
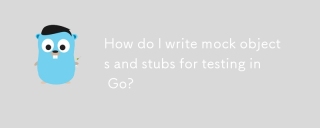
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
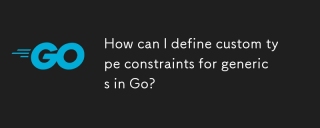
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
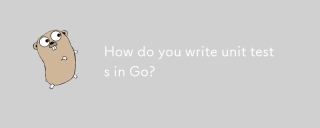
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
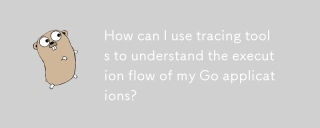
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
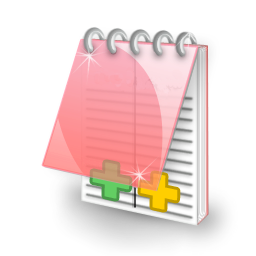
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
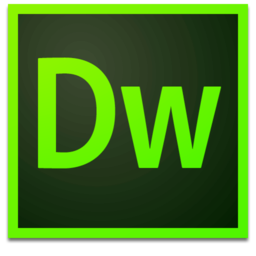
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
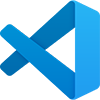
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
