php editor Xinyi introduces you how to use reflect.NewAt on interface{}. reflect.NewAt is a reflection library function in Go language, used to create a new instance on a given interface type. By using reflect.NewAt, we can dynamically create and initialize a new interface instance without specifying a specific type at compile time. This gives us greater flexibility and dynamics, allowing the code to be more versatile and extensible. The following will give you a detailed explanation of how to use reflect.NewAt to create an instance on interface{}.
Question content
package main import ( "encoding/json" "fmt" "reflect" "unsafe" ) type Stu struct { Name string `json:"name"` } func MakeStu() interface{} { return Stu{ Name: "Test", } } func main() { jsonData := []byte(`{"name":"New"}`) t1 := MakeStu() t2 := MakeStu() t3 := MakeStu() json.Unmarshal(jsonData, &t1) fmt.Println(t1) //Type of t1 becomes map[string]interface{} instead of struct Stu newPointer := reflect.New(reflect.ValueOf(t2).Type()).Interface() json.Unmarshal(jsonData, newPointer) fmt.Println(newPointer) //It works,but it need allocate memory to hold temp new variable brokenPointer := reflect.NewAt(reflect.ValueOf(t3).Type(), unsafe.Pointer(&t3)).Interface() json.Unmarshal(jsonData, brokenPointer) fmt.Println(brokenPointer) // I want to get the pointer of original type based on the existed variable t3,but it crashes. }
If I don't know the specific type of interface{} when coding, I can't use interface.(type) to cast. So how to use reflect.newat on interface{}?
If there is an interface that contains a method that returns interface{}, its specific type is struct, but I cannot determine the type of struct when coding. I need to use json.unmarshal to decode data encoded by json. I don't want to get map[string]interface{} , so I need to set the type of the receiver to a pointer to a concrete type of interface{} . Using reflect.new is simple, but consumes extra memory, so I'm curious how to use reflect.newat with an existing interface{}.
Solution
I would like to spend a few words explaining the problem.
Functions we have no control over makestu()
Returns an empty interface - not a concrete type. This will make the concrete type "invisible" to json.unmarshal()
and json.unmarshal()
treat it as an empty interface rather than a concrete type stu
. We have to communicate the concrete type to the unmarshaller somehow.
I would solve this problem using type switches:
func main() { jsondata := []byte(`{"name":"new"}`) t1 := makestu() switch c := t1.(type) { default: _ = json.unmarshal(jsondata, &c) t1 = c } fmt.println(t1) }
The type switch converts the empty interface to a concrete type, and json.unmarshal()
will treat it as a concrete type. You may still have extra allocations, but the code is more readable and you don't rely on reflection.
If I were feeling really adventurous, I would add a helper function that accepts a pointer to an empty interface, like this:
func unmarshal(data []byte, i *interface{}) (err error) { switch c := (*i).(type) { default: err = json.unmarshal(data, &c) *i = c } return err }
This will enable usage like this:
jsonData := []byte(`{"name":"New"}`) t1 := MakeStu() unmarshal(jsonData, &t1)
This looks clean to me and doesn't involve reflection, but it doesn't use reflect.newat()
as you yourself suggested. I hope you find my advice still useful.
The above is the detailed content of How to use reflect.NewAt on interface{}?. For more information, please follow other related articles on the PHP Chinese website!
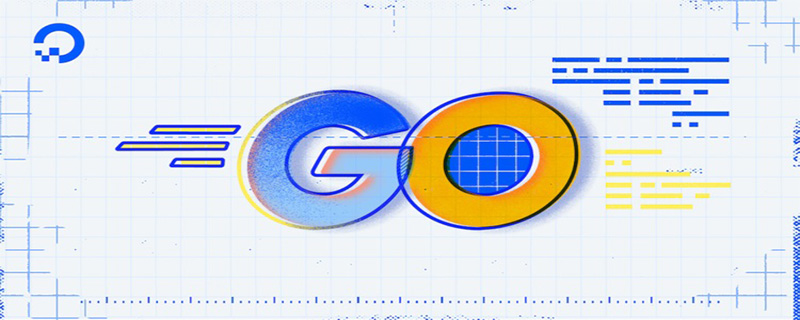
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
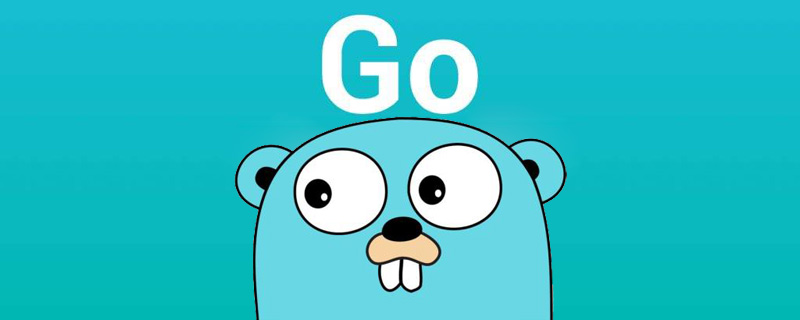
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
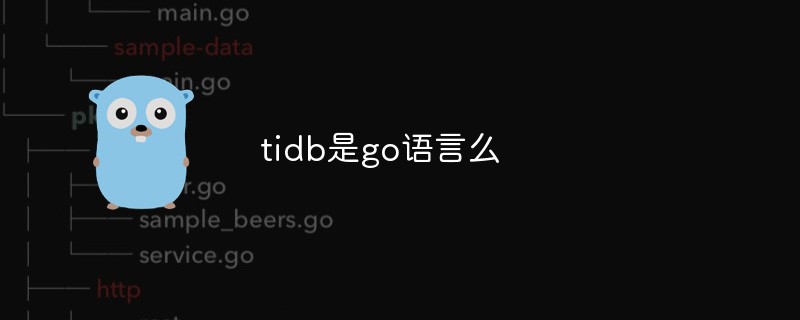
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
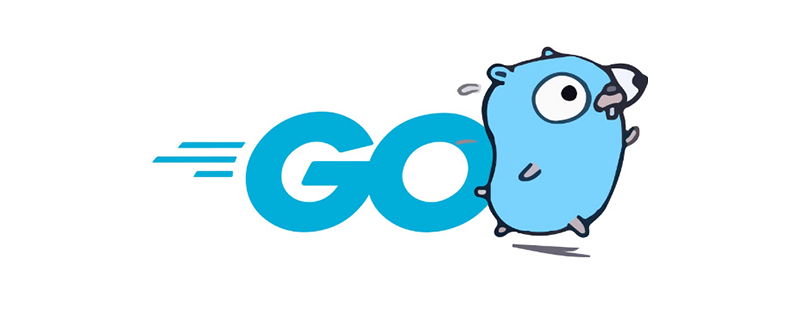
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
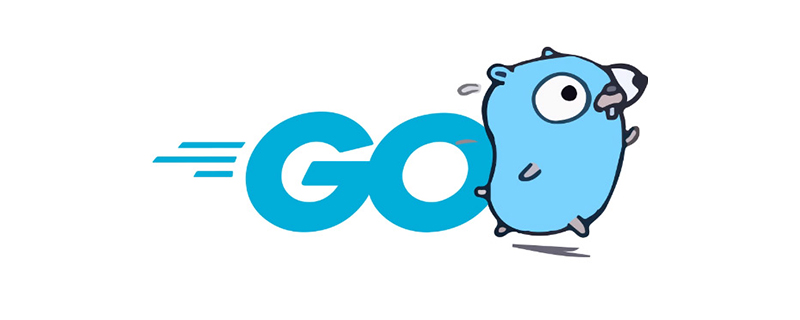
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
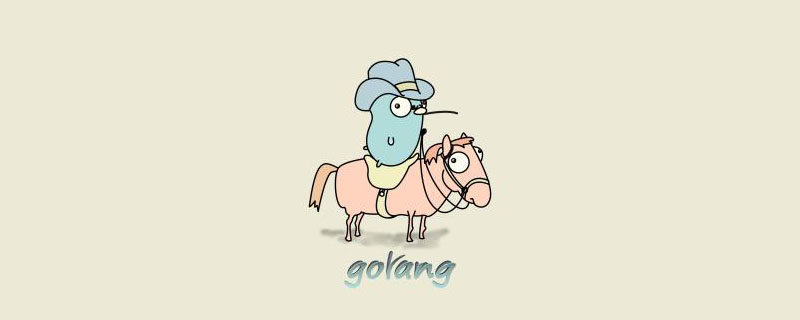
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
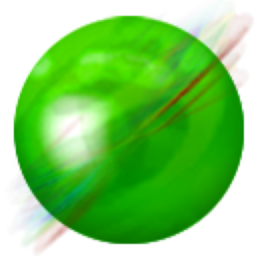
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
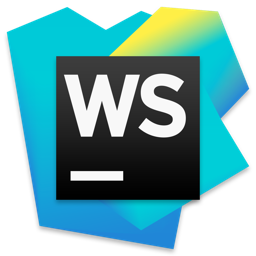
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.