php editor Apple is here to reveal a puzzle about structure slicing: What is the difference between structure slicing and the interface slicing it implements? In the Go language, slices are a convenient and flexible data structure that can change size dynamically. Struct slicing is a special form of slicing, which stores elements of structure type. But how is it different from a slice that implements the same interface? Let us find out the answer together.
Question content
I have an interface Model
, which is implemented by struct Person
.
To get the model instance, I have the following helper function:
func newModel(c string) Model { switch c { case "person": return newPerson() } return nil } func newPerson() *Person { return &Person{} }
The above method allows me to return a Person instance of the correct type (new models can be easily added later using the same method).
When I try to do something like this to return a model slice, I get an error. Code:
func newModels(c string) []Model { switch c { case "person": return newPersons() } return nil } func newPersons() *[]Person { var models []Person return &models }
Go complains: Cannot use newPersons() (type []Person) as a return parameter of type []Model
My goal is to return a slice of whatever model type is requested (whether []Person
, []FutureModel
, []Terminator2000
, w/ e). What am I missing and how to properly implement such a solution?
Solution
This is very similar to the question I just answered: https://www.php.cn/link/2c029952e202c0e560626a4c5980d64c
The short answer is you are right. A slice of a struct is not equal to a slice of the interface implemented by the struct.
[]Person
and []Model
have different memory layouts. This is because the types they belong to have different memory layouts. Model
is an interface value, which means that in memory it is two words in size. One word represents type information and the other word represents data. Person
is a structure whose size depends on the fields it contains. In order to convert from []Person
to []Model
, you need to loop through the array and typecast each element.
Since this conversion is an O(n) operation and results in the creation of a new slice, Go refuses to perform this operation implicitly. You can do this explicitly using the following code.
models := make([]Model, len(persons)) for i, v := range persons { models[i] = Model(v) } return models
As dskinner pointed out , you most likely need a pointer to a slice, not a pointer to a slice. Pointers to slices are generally not needed.
*[]Person // pointer to slice []*Person // slice of pointers
The above is the detailed content of Structure slicing! =The interface slice it implements?. For more information, please follow other related articles on the PHP Chinese website!
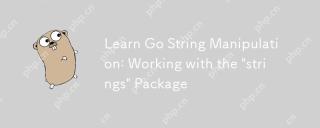
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
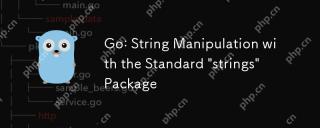
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
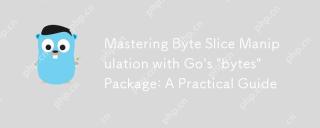
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
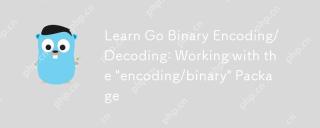
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
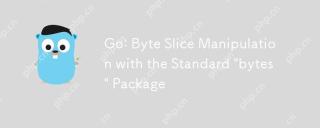
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
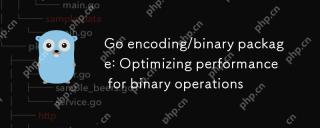
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
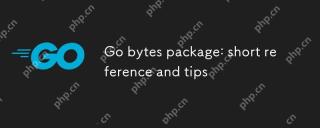
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
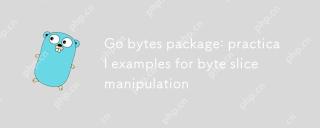
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
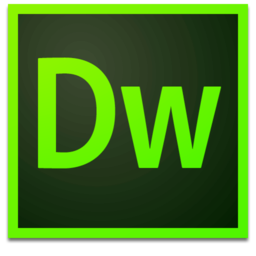
Dreamweaver Mac version
Visual web development tools
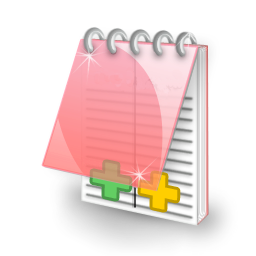
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
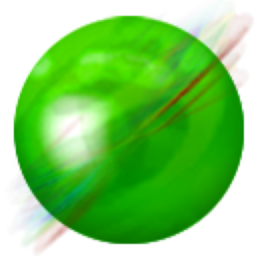
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
