In the Go language, how to gracefully stop the listening server is a common problem. When we need to stop the server, we want to be able to gracefully close all connections without affecting requests being processed. This article will introduce several common methods to help you solve this problem. First, we can use a semaphore to control the shutdown of the server. The os package in Go language provides a Signal method that can be used to capture signals sent by the operating system. We can trigger the shutdown of the server by listening to the os.Interrupt signal, that is, Ctrl C. In the signal processing function, we can perform some cleanup work, such as closing the database connection, saving data, etc. Then, call the server's Shutdown method, which will wait for existing requests to be processed before shutting down the server. This way, we can gracefully stop the listening server. It is worth noting that if the server cannot be shut down within a certain period of time, we can use the WithTimeout method in the context package to set a timeout. After this time is exceeded, the server will be forced to shut down. Using this approach, we can ensure that the server shuts down gracefully under any circumstances. In addition to using semaphores, we can also use a bool type variable to control the shutdown of the server. When the server starts, we can define a global bool variable, such as isShutdown. Then, in the function that handles the request, we can check the value of this variable, and if it is true, return immediately and stop processing the current request. When shutting down the server, we set isShutdown to true and then wait for all requests to be processed before exiting the program. This method is relatively simple, but requires adding additional logic judgments to the function that handles the request, which may slightly affect performance. To sum up, whether using a semaphore or a bool variable, we can implement the function of gracefully stopping the listening server in the Go language. Which method to choose depends on your specific needs and the complexity of your project
Question content
I've been trying to find a way to gracefully stop a listening server in go. Because listen.accept
blocks, it is necessary to close the listening socket to signal the end, but I can't differentiate between that error and any other errors because the related errors are not exported.
Can I do better? See serve()
in the following code in
fixme
package main import ( "io" "log" "net" "time" ) // echo server struct type echoserver struct { listen net.listener done chan bool } // respond to incoming connection // // write the address connected to then echo func (es *echoserver) respond(remote *net.tcpconn) { defer remote.close() _, err := io.copy(remote, remote) if err != nil { log.printf("error: %s", err) } } // listen for incoming connections func (es *echoserver) serve() { for { conn, err := es.listen.accept() // fixme i'd like to detect "use of closed network connection" here // fixme but it isn't exported from net if err != nil { log.printf("accept failed: %v", err) break } go es.respond(conn.(*net.tcpconn)) } es.done <- true } // stop the server by closing the listening listen func (es *echoserver) stop() { es.listen.close() <-es.done } // make a new echo server func newechoserver(address string) *echoserver { listen, err := net.listen("tcp", address) if err != nil { log.fatalf("failed to open listening socket: %s", err) } es := &echoserver{ listen: listen, done: make(chan bool), } go es.serve() return es } // main func main() { log.println("starting echo server") es := newechoserver("127.0.0.1:18081") // run the server for 1 second time.sleep(1 * time.second) // close the server log.println("stopping echo server") es.stop() }
This will print
2012/11/16 12:53:35 Starting echo server 2012/11/16 12:53:36 Stopping echo server 2012/11/16 12:53:36 Accept failed: accept tcp 127.0.0.1:18081: use of closed network connection
I want to hide the accept failed
message, but obviously I don't want to cover up other errors that accept
could be reported. I could of course look at the error test for use of closed network connection
but that would be really ugly. I could set a flag to indicate that I'm about to close and ignore the error if it's set, I guess - is there a better way?
Workaround
Check for some "time to stop" flag in the loop after the accept()
call and then flip it from main
, Then connect to your listening port to get the server socket "without getting stuck". This is very similar to the old "self-pipeline trick".
The above is the detailed content of How to stop listening on a server in Go. For more information, please follow other related articles on the PHP Chinese website!
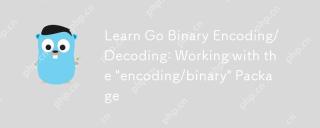
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
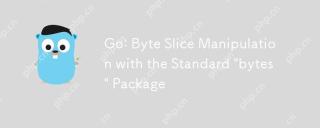
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
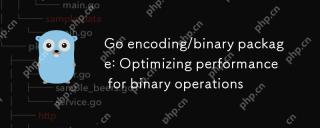
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
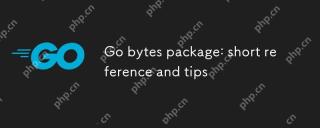
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
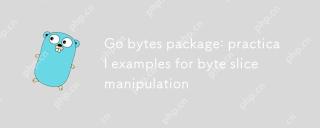
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.
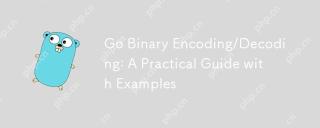
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
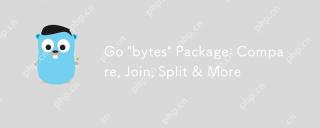
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
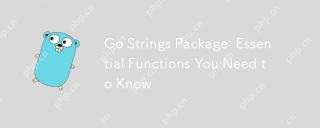
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
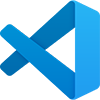
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
