


Is it possible to wrap http.ServeHttp in go to add session using alexedwards/scs/v2
php hello editor Zimo! Regarding your question about wrapping http.ServeHTTP in Go to add sessions using alexedwards/scs/v2, the answer is yes. You can use the alexedwards/scs/v2 library to manage sessions and wrap http.ServeHTTP in your Go code. This way you can add session management capabilities to use sessions in your application when handling HTTP requests. This way you can easily add authentication, authorization, and other session-related functionality to your application. Hope this answer is helpful to you!
Question content
I'm trying to add a session to an existing http server written in go. I have the following code
type httpserver struct { getroutes map[string]http.handlerfunc // pattern => handler postroutes map[string]http.handlerfunc server http.server } func (s *httpserver) run() { address := "127.0.0.1:8080" s.server = http.server{ addr: address, handler: s, readtimeout: 10 * time.second, writetimeout: 10 * time.second, maxheaderbytes: 1 << 20, } log.fatal(s.server.listenandserve()) } func (s *httpserver) servehttp(writer http.responsewriter, r *http.request) { ... }
I want to add session using https://pkg.go.dev/github.com/alexedwards/scs/v2#sessionmanager.loadandsave
The sample code in the link is
mux := http.NewServeMux() mux.HandleFunc("/put", putHandler) mux.HandleFunc("/get", getHandler) // Wrap your handlers with the LoadAndSave() middleware. http.ListenAndServe(":4000", sessionManager.LoadAndSave(mux))
The example code passes mux to loadandsave and then passes the new handler to http.listenandserve(port, newhandler). In my case, the handler came from the servehttp method that I added to the *httpserver structure. The handler in the example comes from a multiplexer.
I am new here. Is it possible to pass my servehttp method to loadandsave and use the handler returned from loadandsave? If not, is there a way to pass the http.server struct fields used in my example into http.listenandserve(port, handler) ?
Solution
Just like peter’s func (s *httpserver) run(sessionmanager *scs.sessionmanager) {
address := "127.0.0.1:8080"
// wrap your httpserver with the loadandsave middleware.
handlerwithsessions := sessionmanager.loadandsave(s)
...
}
This means that the For example:sessionmanager
is created and configured outside this method (using scs.new()) and passed in when calling run
.
There you can set the sessionmanager
to a field in the httpserver
structure.
This will allow your (s *httpserver) servehttp(writer http.responsewriter, r *http.request)
to retrieve it. package main
import (
"github.com/alexedwards/scs/v2"
"log"
"net/http"
"time"
)
type HttpServer struct {
getRoutes map[string]http.HandlerFunc // pattern => handler
postRoutes map[string]http.HandlerFunc
server http.Server
sessionManager *scs.SessionManager
}
func (s *HttpServer) Run(sessionManager *scs.SessionManager) {
address := "127.0.0.1:8080"
// Set the sessionManager field
s.sessionManager = sessionManager
// Wrap your HttpServer with the LoadAndSave middleware.
handlerWithSessions := sessionManager.LoadAndSave(s)
s.server = http.Server{
Addr: address,
Handler: handlerWithSessions, // Use the wrapped handler
ReadTimeout: 10 * time.Second,
WriteTimeout: 10 * time.Second,
MaxHeaderBytes: 1 << 20,
}
log.Fatal(s.server.ListenAndServe())
}
func (s *HttpServer) ServeHTTP(writer http.ResponseWriter, r *http.Request) {
// Access the session via the sessionManager field
session := s.sessionManager.Load(r)
// Use the session, e.g. session.Put, session.Get, etc.
// ...
}
func main() {
// Create and configure the session manager
sessionManager := scs.New()
sessionManager.Lifetime = 24 * time.Hour
// Create your custom HttpServer
httpServer := &HttpServer{
getRoutes: make(map[string]http.HandlerFunc),
postRoutes: make(map[string]http.HandlerFunc),
}
// Start the server with the session manager
httpServer.Run(sessionManager)
}
The above is the detailed content of Is it possible to wrap http.ServeHttp in go to add session using alexedwards/scs/v2. For more information, please follow other related articles on the PHP Chinese website!
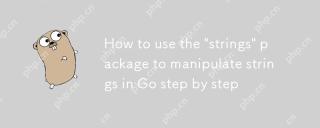
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
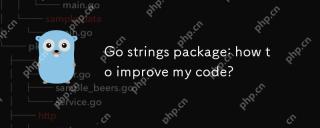
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
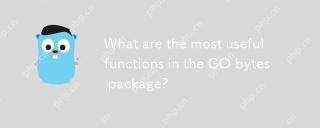
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
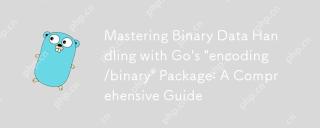
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
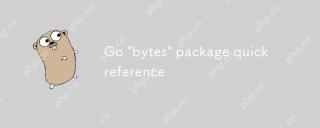
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
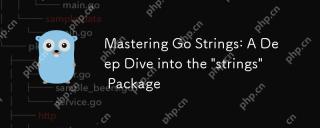
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
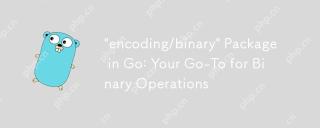
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
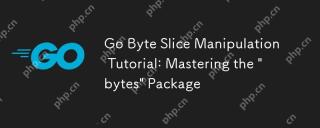
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
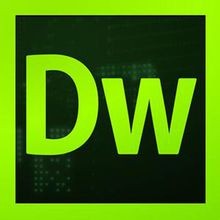
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
