php Xiaobian Yuzai introduces you to a common data structure algorithm - "Intra-row reverse linked list". In this algorithm, we need to reverse the order of nodes in a linked list. Through concise and efficient code implementation, we can complete this operation in one line, completely reversing the order of the linked list. This algorithm is very useful in actual programming and can play an important role in both data processing and algorithm design. Let’s learn about this wonderful algorithm together!
Question content
I just found the solution for reverse linked list using one line in go on leetcode. It does work, but I don't understand how to implement it.
That's it:
func reverselist(head *listnode) (prev *listnode) { for head != nil { prev, head, head.next = head, head.next, prev } return }
For example, let the list be [1->2->3->4->5->nil]
.
I know it works like this:
-
First execute
head.next = prev
(head.next = nil
, so nowhead = [1->nil]
) -
Then,
prev = head
(In this stepprev = [1->nil]
just likehead
in the previous step ) -
head = head.next
This is magic. Forprev
in the second step of go, usehead = [1->nil]
, but after this stephead = [2->3-> ;4->5->nil]
So when head != nil
it iterates and in the second step prev = [2->1->nil]
, head = [3->4->5->nil]
and so on.
This line can be expressed as:
for head != nil { a := *head prev, a.Next = &a, prev head = head.Next }
Am I right? Why is this happening?
Solution
The variable on the left side of the expression will be assigned to the value on the right side of the expression at that time. This is a clever use of language.
To make it easier to understand, let’s look at an example.
set up
This is our link list: 1 -> 2 -> 3 -> 4 -> None
Before the function is executed,
- The head is *node 1
- prev is zero (uninitialized)
- head.next is *node 2
Step by step
prev, head, head.next = head, head.next, prev
Let’s break it down,
- prev (nil) = head (*node 1)
- head (*Node 1) = head.next (*Node 2)
- head.next (*node 2) = prev (nil)
Next iteration,
- prev (*node 1) = head (*node 2)
- head (*Node 2) = head.next (*Node 3)
- head.next (*node 3) = prev (*node 1)
Summary
Basically, it reverses head.next
to the previous node and moves prev and head to the next node.
Compare this to a textbook algorithm in go to be clear:
func reverseList(head *ListNode) *ListNode { var prev *ListNode for head != nil { nextTemp := head.Next head.Next = prev prev = head head = nextTemp } return prev }
The above is the detailed content of Reverse linked list in one row. For more information, please follow other related articles on the PHP Chinese website!
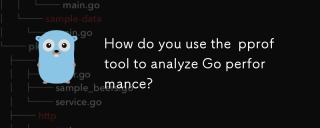
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
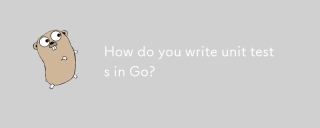
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
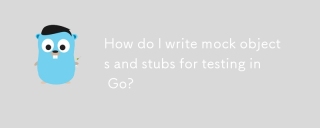
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
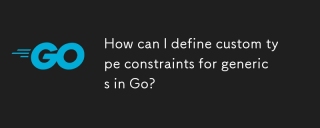
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
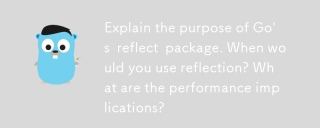
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
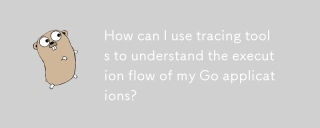
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
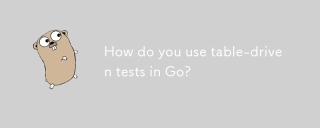
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
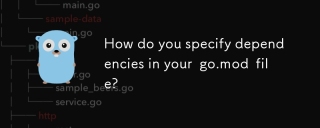
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
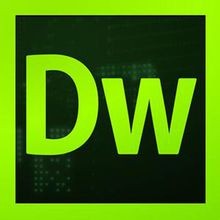
Dreamweaver CS6
Visual web development tools
