


php editor Strawberry will introduce to you how to ensure the correctness of the IPv4 packets created for the local "ping" implementation. In network communication, use the Ping command to test the connectivity between hosts. However, in practical applications, we need to ensure the correctness of the IPv4 data packets sent to avoid errors or losses. To this end, we can take some measures to ensure the accuracy and completeness of data packets to ensure that we get accurate Ping results. Next, let’s take a look at these measures.
Question content
Overview
I've been working on a side project that is essentially a network troubleshooting tool. My goal is to deepen my understanding of networking basics and become proficient in using the troubleshooting tools provided by the operating system.
This is a CLI application that will get the hostname and try to diagnose the problem if any. The plan is to implement ping and traceroute first and then gradually implement other tools based on my comfort level.
However, my ping implementation is not accurate because the IPv4 packets are malformed. This is what wireshark has to say.
1 0.000000 192.168.0.100 142.250.195.132 ICMP 300 Unknown ICMP (obsolete or malformed?)
Code
This is how I implement ping
<code>package ping import ( "encoding/json" "net" "github.com/pkg/errors" ) var ( IcmpProtocolNumber uint8 = 1 IPv4Version uint8 = 4 IPv4IHL uint8 = 5 ICMPHeaderType uint8 = 8 ICMPHeaderSubtype uint8 = 0 ) type NativePinger struct { SourceIP string DestIP string } type ICMPHeader struct { Type uint8 Code uint8 Checksum uint16 } type ICMPPacket struct { Header ICMPHeader Payload interface{} } type IPv4Header struct { SourceIP string DestinationIP string Length uint16 Identification uint16 FlagsAndOffset uint16 Checksum uint16 VersionIHL uint8 DSCPAndECN uint8 TTL uint8 Protocol uint8 } type IPv4Packet struct { Header IPv4Header Payload *ICMPPacket } func (p *NativePinger) createIPv4Packet() (*IPv4Packet, error) { versionIHL := (IPv4Version << 4) | IPv4IHL icmpPacket := &ICMPPacket{ Header: ICMPHeader{ Type: ICMPHeaderType, Code: ICMPHeaderSubtype, }, } ipv4Packet := &IPv4Packet{ Header: IPv4Header{ VersionIHL: versionIHL, DSCPAndECN: 0, Identification: 0, FlagsAndOffset: 0, TTL: 64, Protocol: IcmpProtocolNumber, SourceIP: p.SourceIP, DestinationIP: p.DestIP, }, Payload: icmpPacket, } ipv4Packet.Header.Length = 40 bytes, err := json.Marshal(icmpPacket) if err != nil { return nil, errors.Wrapf(err, "error converting ICMP packet to bytes") } icmpPacket.Header.Checksum = calculateChecksum(bytes) bytes, err = json.Marshal(ipv4Packet) if err != nil { return nil, errors.Wrapf(err, "error converting IPv4 packet to bytes") } ipv4Packet.Header.Checksum = calculateChecksum(bytes) return ipv4Packet, nil } func calculateChecksum(data []byte) uint16 { sum := uint32(0) // creating 16 bit words for i := 0; i < len(data)-1; i++ { word := uint32(data[i])<<8 | uint32(data[i+1]) sum += word } if len(data)%2 == 1 { sum += uint32(data[len(data)-1]) } // adding carry bits with lower 16 bits for (sum >> 16) > 0 { sum = (sum & 0xffff) + (sum >> 16) } // taking one's compliment checksum := ^sum return uint16(checksum) } func (p *NativePinger) ResolveAddress(dest string) error { ips, err := net.LookupIP(dest) if err != nil { return errors.Wrapf(err, "error resolving address of remote host") } for _, ip := range ips { if ipv4 := ip.To4(); ipv4 != nil { p.DestIP = ipv4.String() } } // The destination address does not need to exist as unlike tcp, udp does not require a handshake. // The goal here is to retrieve the outbound IP. Source: https://stackoverflow.com/a/37382208/3728336 // conn, err := net.Dial("udp", "8.8.8.8:80") if err != nil { return errors.Wrapf(err, "error resolving outbound ip address of local machine") } defer conn.Close() p.SourceIP = conn.LocalAddr().(*net.UDPAddr).IP.String() return nil } func (p *NativePinger) Ping(host string) error { if err := p.ResolveAddress(host); err != nil { return errors.Wrapf(err, "error resolving source/destination addresses") } packet, err := p.createIPv4Packet() if err != nil { return errors.Wrapf(err, "error creating IPv4Packet") } conn, err := net.Dial("ip4:icmp", packet.Header.DestinationIP) if err != nil { return errors.Wrapf(err, "error eshtablishing connection with %s", host) } defer conn.Close() bytes, err := json.Marshal(packet) if err != nil { return errors.Wrapf(err, "error converting IPv4 packet into bytes") } _, err = conn.Write(bytes) if err != nil { return errors.Wrapf(err, "error sending ICMP echo request") } buff := make([]byte, 2048) _, err = conn.Read(buff) // The implementation doesn't proceed beyond this point if err != nil { return errors.Wrapf(err, "error receiving ICMP echo response") } return nil } </code>
Introspection
I'm not sure if the packet malformation is caused by a single cause or multiple causes. I think the problem lies in one of these two places (or both?):
-
Incorrect header length calculation I manually calculated the length to be
40 bytes (wordsize = 4 bytes)
. Write structure fields in an order that prevents structure corruption. I refer to this source for information on various types of sizes.
<code>// 1 word (4 bytes) type ICMPHeader struct { Type uint8 // 8 bit Code uint8 // 8 bit Checksum uint16 // 16 bit } // 3 words (3*4 = 12 bytes) type ICMPPacket struct { Header ICMPHeader // 1 word Payload interface{} // 2 words } // 7 words (7*4 = 28 bytes) type IPv4Header struct { // Below group takes 4 words (each string takes 2 words) SourceIP string DestinationIP string // Following group takes 2 words (each 16 bits) Length uint16 Identification uint16 FlagsAndOffset uint16 Checksum uint16 // Below group takes 1 word (each takes 8 bits) VersionIHL uint8 DSCPAndECN uint8 TTL uint8 Protocol uint8 } // 10 words (40 bytes) type IPv4Packet struct { Header IPv4Header // 7 words as calculated above Payload ICMPPacket // 3 words as calculated above } </code>
- Checksum calculation is incorrectI implemented the Internet checksum algorithm. If this isn't what I'm supposed to be doing here, please tell me.
There are some parts missing in the implementation, such as configuring counts, assigning sequence numbers to packets, etc., but before that the basic implementation needs to be fixed, i.e. receiving responses for ICMP ECHO packets. Good to know where I made the mistake.
Thanks!
Updated August 24, 2023
Taking into account the advice I got in the comments, I have updated the code to fix the byte order and use raw bytes for the source address, destination address. However, this alone doesn't solve the problem, the packet is still malformed, so there must be something else going on.
Solution
I finally got it working. I should talk about a few issues with the code.
Serialization problem
As Andy correctly pointed out, I'm sending a JSON object, not raw bytes in network byte order. This is fixed using binary.Write(buf, binary.BigEndian, field)
However, since this method only works with fixed-size values, I have to do this for each struct field, making the code repetitive and somewhat ugly.
Structural optimization and serialization are different issues.
I am aware of the practice of combining the Version
and IHL
fields together to optimize memory, that's why I have this single field VersionIHL
in my structure. But when serializing, the field values (4 and 5 in this case) will be serialized individually, and I didn't do that. Instead, I convert the entire VersionIHL
field's value into bytes.
As a result, I found myself sending an unexpected octet 69
in the byte stream from combining 4
and 5
grouped together 0100 0101
.
Incomplete ICMP packet
My ICMP structure does not contain identifier and sequence number fields. The information provided in the ICMP datagram header section on Wikipedia feels a bit generic. However, I found the details on the RFC page (page 14) to be much more insightful.
This feels strange considering the importance of the serial number of the ping utility. During implementation, I often found myself wondering where the serial number was placed appropriately in the code. It wasn't until I stumbled across the RFC page that I had a clear idea of when and where to incorporate serial numbers.
For anyone who might be interested, here is the function code I've put it together.
The above is the detailed content of How do I ensure the correctness of the IPv4 packets created for the native 'ping' implementation?. For more information, please follow other related articles on the PHP Chinese website!
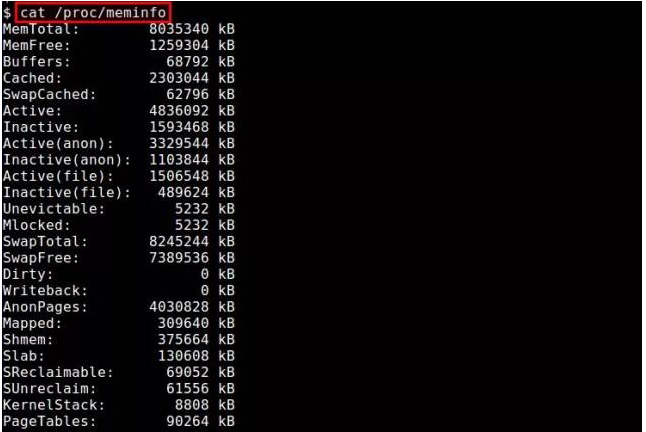
Q:我有一个问题,我想要监视Linux系统的内存使用情况。在Linux下有哪些可用的视图或命令行工具可以使用呢?A:在Linux系统中,有多种方法可以监视内存使用情况。下面是一些通过视图工具或命令行来查看内存使用情况的方法。/proc/meminfo:最简单的方法是查看/proc/meminfo文件。这个虚拟文件会动态更新,并提供了关于内存使用情况的详细信息。它列出了各种内存指标,可以满足你对内存使用情况的大部分需求。另外,你还可以通过/proc//statm和/proc//status来查看进
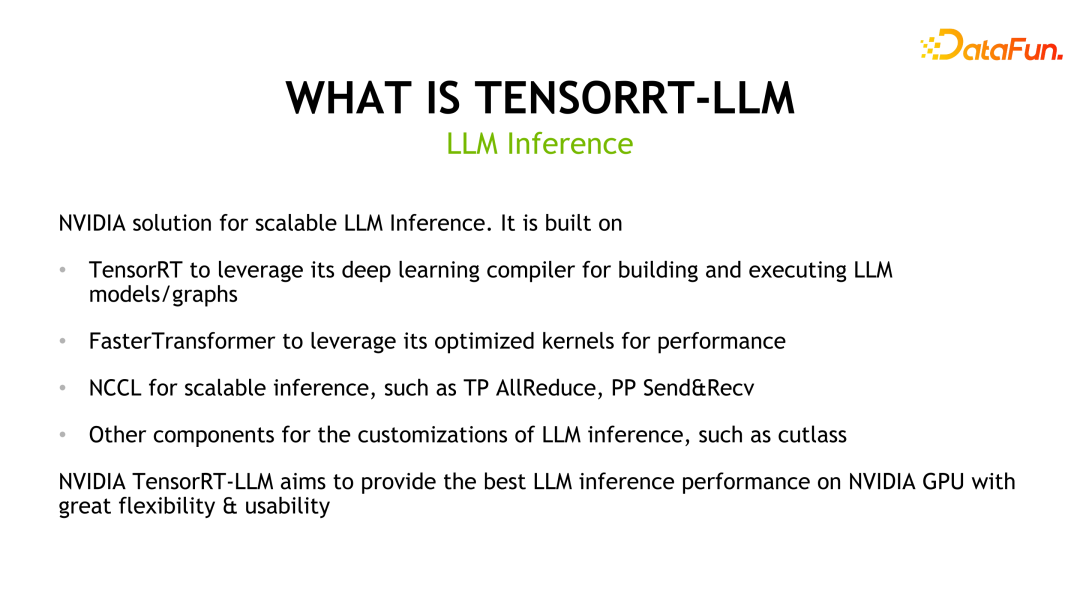
一、TensorRT-LLM的产品定位TensorRT-LLM是NVIDIA为大型语言模型(LLM)开发的可扩展推理方案。它基于TensorRT深度学习编译框架构建、编译和执行计算图,并借鉴了FastTransformer中高效的Kernels实现。此外,它还利用NCCL实现设备间的通信。开发者可以根据技术发展和需求差异,定制算子以满足特定需求,例如基于cutlass开发定制的GEMM。TensorRT-LLM是NVIDIA官方推理方案,致力于提供高性能并不断完善其实用性。TensorRT-LL
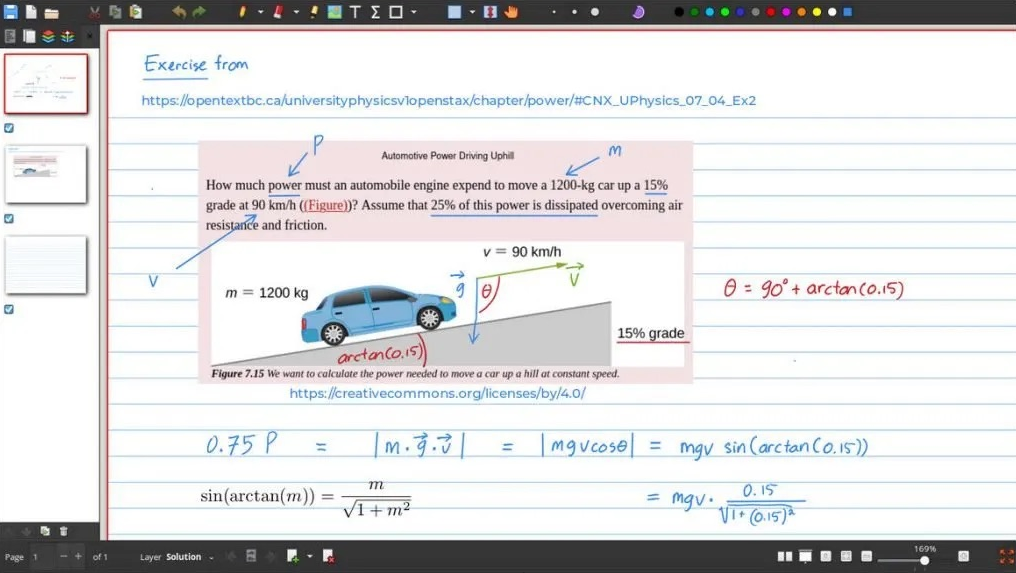
“我们将介绍几款适用于Linux系统的白板应用程序,相信这些信息对您会非常有帮助。请继续阅读!”一般来说,数字白板是一种用于大型互动显示面板的工具,常见的设备类型包括平板电脑、大屏手机、触控笔记本和表面显示设备等。当教师使用白板时,您可以使用触控笔、手写笔、手指甚至鼠标在设备屏幕上进行绘画、书写或操作元素。这意味着您可以在白板上拖动、点击、删除和绘画,就像在纸上使用笔一样。然而,要实现这一切,需要有一款软件来支持这些功能,并实现触控和显示之间的精细协调。目前市面上有许多商业应用可以完成这项工作。
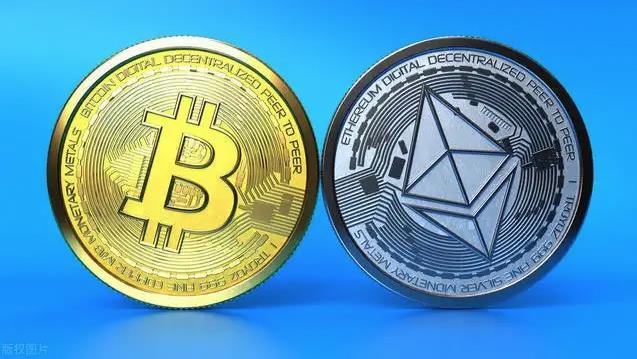
ZRX(0x)是一个基于以太坊区块链的开放协议,用于实现分布式交易和去中心化交易所(DEX)功能。作为0x协议的原生代币,ZRX可用于支付交易费用、治理协议变更和获取平台优惠。1.ZRX币升值空间展望:从技术角度来看,ZRX作为0x协议的核心代币,在去中心化交易所的应用逐渐增多,市场对其认可度也在增加。以下是几个关键因素,有助于提升ZRX币的价值空间:市场需求潜力大、社区活跃度高、开发者生态繁荣等。这些因素共同促进了ZRX的广泛应用和使用,进而推动了其市场价格的上升。市场需求的增长潜力,意味着更
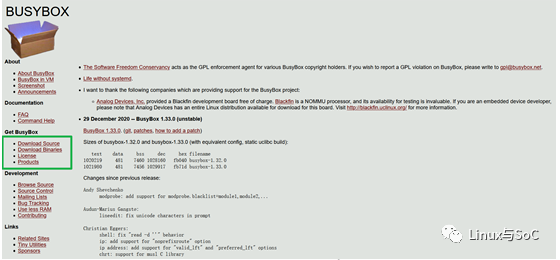
busybox概述众所周知,在Linux环境下,一切皆文件,文件可以表示一切。而文件系统则是这些普通组件的集合。在嵌入式领域中,常常使用基于busybox构建的rootfs来构建文件系统。busybox诞生至今已有近20年的历史,如今已成为嵌入式行业中主流的rootfs构建工具。busybox的代码是完全开源的。你可以进入官方网站,点击”GetBusyBox”下面的”DownloadSource”进入源码下载界面。“官方网站链接:https://busybox.net/”2.busybox的配置
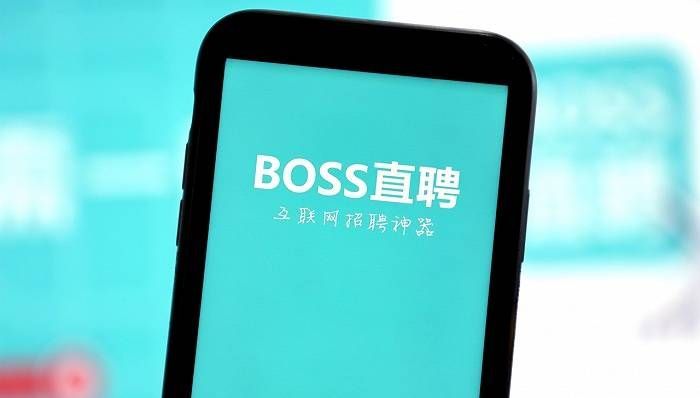
BOSS直聘怎么创建多个简历?BOSS直聘是很多小伙伴找工作的一大招聘平台,为用户们提供了非常多便利的求职服务。各位在使用BOSS直聘的时候,可以创建多个不同的简历,以便投送到不同的工作岗位上,获取到更高成功率的求职操作,各位如果对此感兴趣的话,就随小编一起来看看BOSS直聘双简历创建教程吧。BOSS直聘怎么创建多个简历1.登录Boss直聘:在您的电脑或手机上,登录您的Boss直聘账户。2.进入简历管理:在Boss直聘首页,点击“简历管理”,进入简历管理页面。3.创建新简历:在简历管理页面,点击

最近,我正在进行一个项目,遇到了一个问题。在ARM上运行的ThreadX与DSP通信时采用了消息队列的方式传递消息(最终实现使用了中断和共享内存的方法)。然而,在实际的操作过程中,发现ThreadX经常崩溃。经过排查,发现问题出在传递消息的结构体没有考虑字节对齐的问题上。我想顺便整理一下关于C语言中字节对齐的问题,并与大家分享。一、概念字节对齐与数据在内存中的位置有关。如果一个变量的内存地址恰好是它长度的整数倍,那么它就被称为自然对齐。例如,在32位CPU下,假设一个整型变量的地址为0x0000
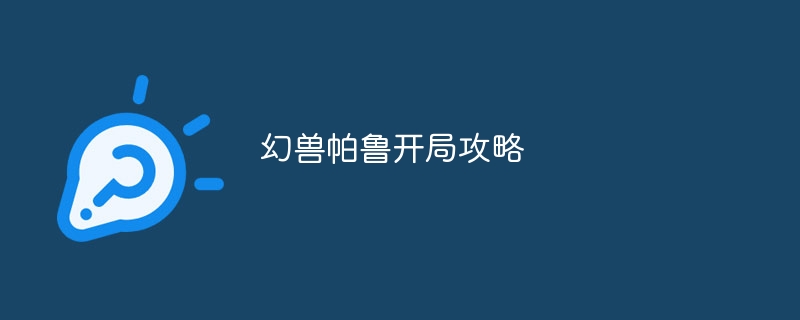
幻兽帕鲁游戏开局就可以拿三个宝箱和两个宠物蛋,拿完以后就可以开始建家了,建家的地址一定要选好,要不然开局会发现自己的家没有了,然后就是捕捉一直适合自己的帕鲁,作为自己的伙伴或者打工人即可。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
