For example, if I have the number 35565, the output is 3565.
So, my code snippet gets the single digits, but I don't know how to keep the previous number to check with the next number.
for { num = num / 10 fmt.Print(num) if num/10 == 0 { break } }
Correct answer
This method breaks the number into numbers from right to left, stores them as integer slices, and then iterates over the numbers from left to right to Construct numbers with "consecutively unique" digits.
I initially tried breaking the number from left to right, but didn't know how to handle the placeholder zeros; breaking it up right to left, I knew how to capture those zeros.
// unique removes sequences of repeated digits from non-negative x, // returning only "sequentially unique" digits: // 12→12, 122→12, 1001→101, 35565→3565. // // Negative x yields -1. func unique(x int) int { switch { case x < 0: return -1 case x <= 10: return x } // -- Split x into its digits var ( mag int // the magnitude of x nDigits int // the number of digits in x digits []int // the digits of x ) mag = int(math.Floor(math.Log10(float64(x)))) nDigits = mag + 1 // work from right-to-left to preserve place-holding zeroes digits = make([]int, nDigits) for i := nDigits - 1; i >= 0; i-- { digits[i] = x % 10 x /= 10 } // -- Build new, "sequentially unique", x from left-to-right var prevDigit, newX int for _, digit := range digits { if digit != prevDigit { newX = newX*10 + digit } prevDigit = digit } return newX }
This is a go playground for testing.
Can be adapted to handle negative numbers by flipping the negative sign at the beginning and restoring it at the end.
The above is the detailed content of How to print a number that is different from the previous number?. For more information, please follow other related articles on the PHP Chinese website!
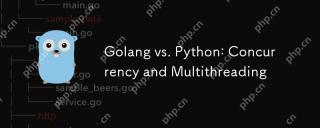
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
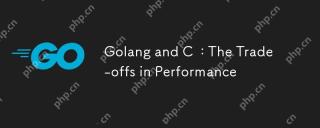
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
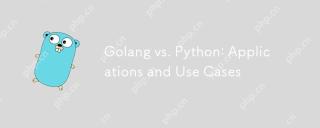
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
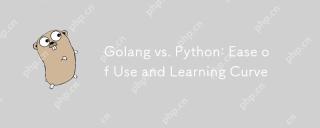
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
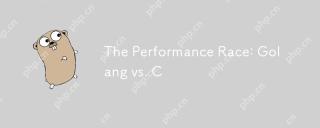
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
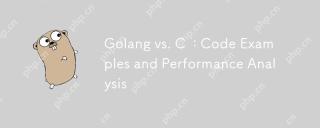
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
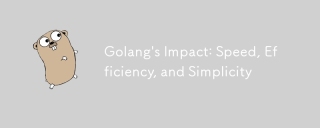
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
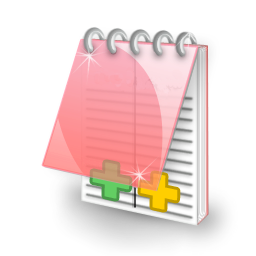
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
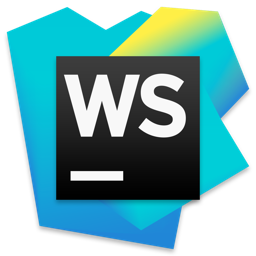
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment