There is an interface declaration and many structures that implement it
type datainterface interface { get(string) string } type dataa struct { d map[string]string } func (d *dataa) get(key string) string { return d.d[key] } func (d *dataa) getid() string { return d.get("id") } type datab struct { d map[string]string } func (d *datab) get(key string) string { return d.d[key] } func (d *datab) getfile() string { return d.get("file") } type datac...
Also includes datac,d,e...
I will store these datax
struct instances into type dataslice []datainterface
Now if I want to get datax
I can do this:
type dataslice []datainterface func (d dataslice) geta() []*dataa { var ret []*dataa for _, di := range d { if v, ok := di.(*dataa); ok { ret = append(ret, v) } } return ret } func (d dataslice) getb() []*datab { var ret []*datab for _, di := range d { if v, ok := di.(*datab); ok { ret = append(ret, v) } } return ret } func (d dataslice) getc() .....
Obviously there is a lot of duplicate code here:
var ret []*datax for _, di := range d { if v, ok := di.(*datax); ok { ret = append(ret, v) } }
So I thought I could use generics to solve this problem, then I defined this function:
func GetDataX[T any] (d DataInterface) *T { return d.(*T) }
But an error occurred: impossible type assertion: '*t' does not implement 'datainterface
So I want to know is this really impossible? Or can it be done some other way?
Correct answer
You should be able to use the following code to meet your needs:
package main import "fmt" // interface type DataInterface interface { Get(string) string } // struct implementing the interface type DataA struct { d map[string]string } func (d DataA) Get(key string) string { return d.d[key] } type DataB struct { d map[string]string } func (d DataB) Get(key string) string { return d.d[key] } type DataSlice []DataInterface func GetDataX[T any](d DataInterface) T { return d.(T) } func main() { a := DataA{map[string]string{"a": "1"}} b := DataB{map[string]string{"b": "2"}} ds := DataSlice{a, b} for _, v := range ds { if value, ok := v.(DataA); ok { fmt.Printf("A\t%q\n", GetDataX[DataA](value)) continue } if value, ok := v.(DataB); ok { fmt.Printf("B\t%q\n", GetDataX[DataB](value)) continue } // add unknown type handling logic here } }
First, I simplified the code to only consider the dataa
and datab
structures. I then changed the Pointer Receiver to a Value Receiver since you are not changing the state of the actual instance passed to the method. Because of this change, getdatax
works successfully and you can get all the information for similar structures.
If this solves your problem or you need something else, please let me know, thank you!
The above is the detailed content of How to convert an interface to a specified type using generics. For more information, please follow other related articles on the PHP Chinese website!
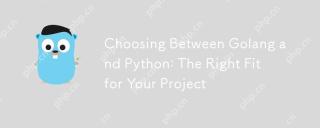
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
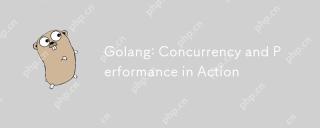
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
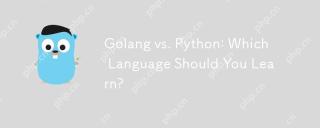
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
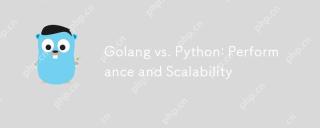
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
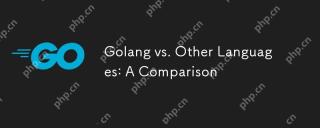
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
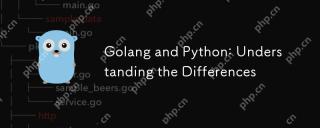
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
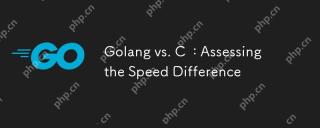
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
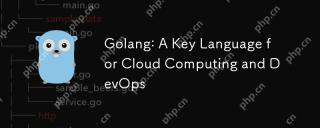
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool