


Use Python to learn the principles and practical application scenarios of selection sorting algorithm
Learn the basic ideas and applications of selection sorting through Python
Selection Sort is a simple and intuitive sorting algorithm. Its basic idea is to Select the smallest (or largest) element from the sorted data and place it at the end of the sorted area, then select the smallest (or largest) element from the remaining unsorted data and place it at the end of the sorted area, and so on, until All data is sorted.
The specific steps of selection sorting are as follows:
- First, find the smallest (or largest) element from the data to be sorted, and exchange it with the first element.
- Then, find the smallest (or largest) element from the remaining unsorted data and swap its position with the second element.
- Repeat the above steps, sequentially exchanging the minimum (or maximum) value in the remaining unsorted data with the end element of the sorted area until all data is sorted.
The following is a code example using Python to implement selection sorting:
def selection_sort(arr): n = len(arr) for i in range(n-1): min_idx = i for j in range(i+1, n): if arr[j] < arr[min_idx]: min_idx = j arr[i], arr[min_idx] = arr[min_idx], arr[i] # 测试代码 arr = [64, 25, 12, 22, 11] selection_sort(arr) print("排序后的数组:") for i in range(len(arr)): print(arr[i], end=" ")
The selection_sort
function in the above code implements the selection sorting algorithm. In each loop, min_idx
records the index of the minimum value in the current unsorted area, finds the minimum value in the unsorted area in the inner loop, and uses an exchange operation to combine it with the index of the sorted area. The last elements are swapped. Finally, through multiple loops, the entire array is sorted.
The output result of the above code is:
排序后的数组: 11 12 22 25 64
The time complexity of selection sorting is O(n^2), so it is not suitable for situations with large amounts of data. However, the implementation of selection sort is relatively simple and the code is easy to understand, so it still has certain application value in some specific scenarios.
Through the above code examples and explanations, we have learned the basic ideas and applications of selection sorting. I hope it will be helpful for you to understand and master the selection sort algorithm.
The above is the detailed content of Use Python to learn the principles and practical application scenarios of selection sorting algorithm. For more information, please follow other related articles on the PHP Chinese website!
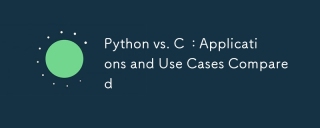
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
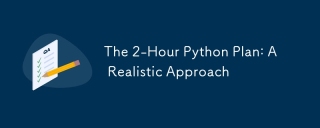
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
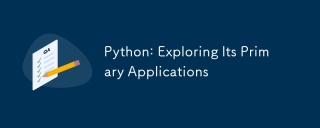
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
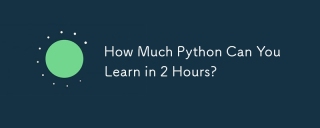
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
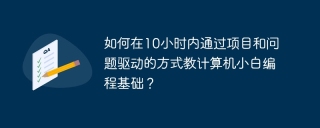
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
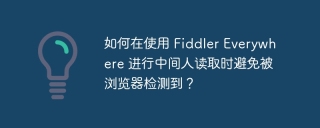
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
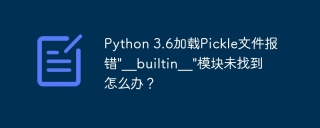
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
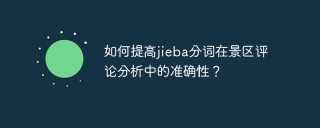
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
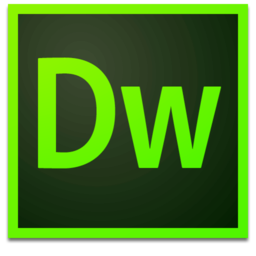
Dreamweaver Mac version
Visual web development tools
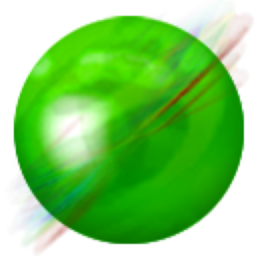
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use