


Application tips for decrypting Guava cache: an artifact to improve application performance
Guava cache usage tips
Guava cache is a high-performance memory cache that can significantly improve application performance. It provides a variety of caching strategies, and you can choose the most appropriate caching strategy according to different scenarios.
Basic use of Guava cache
The basic use of Guava cache is very simple and only requires a few lines of code.
import com.google.common.cache.CacheBuilder; import com.google.common.cache.CacheLoader; import com.google.common.cache.LoadingCache; public class GuavaCacheExample { public static void main(String[] args) { // 创建一个缓存,最大容量为100个元素,过期时间为10秒 LoadingCache<String, String> cache = CacheBuilder.newBuilder() .maximumSize(100) .expireAfterAccess(10, TimeUnit.SECONDS) .build(new CacheLoader<String, String>() { @Override public String load(String key) { // 从数据库中加载数据 return loadFromDatabase(key); } }); // 将数据放入缓存中 cache.put("key1", "value1"); // 从缓存中获取数据 String value = cache.get("key1"); // 判断缓存中是否存在数据 boolean exists = cache.getIfPresent("key1") != null; } private static String loadFromDatabase(String key) { // 模拟从数据库中加载数据 return "value" + key; } }
Caching strategy of Guava cache
Guava cache provides a variety of caching strategies, and you can choose the most appropriate caching strategy according to different scenarios.
Size-based caching strategy
Size-based caching strategy refers to deciding whether to put data into the cache based on the size of the cache. Guava cache provides two size-based caching strategies:
- maximumSize(): Set the maximum capacity of the cache. When the cache reaches maximum capacity, the oldest data put into the cache will be evicted.
- weigher(): Set the cache weight function. The weight function can calculate the weight of data based on its size or other factors. When the cache reaches its maximum capacity, the data with the greatest weight will be evicted.
Time-based caching strategy
The time-based caching strategy refers to deciding whether to put data into the cache based on the expiration time of the data. Guava cache provides two time-based caching strategies:
- expireAfterWrite(): Set the expiration time of data in the cache. When the data stored in the cache exceeds the expiration time, it will be eliminated.
- expireAfterAccess(): Set the expiration time after the data is accessed in the cache. When the data is accessed in the cache, the expiration time is recalculated.
Reference-based caching strategy
Reference-based caching strategy refers to deciding whether to put data into the cache based on the reference count of the data. Guava cache provides two reference-based caching strategies:
- weakKeys(): Set cache keys to weak references. When a key is garbage collected, the data in the cache will be evicted.
- softValues(): Set cached values to soft references. When a value is garbage collected, the data in the cache is evicted.
Tips for using Guava cache
When using Guava cache, you can pay attention to the following points:
- Choose the appropriate caching strategy: Choose the most appropriate caching strategy according to different scenarios.
- Set the cache capacity reasonably: The cache capacity should not be too large, otherwise it will occupy too much memory.
- Set the expiration time reasonably: The expiration time should not be too long, otherwise the data in the cache may become invalid.
- Pay attention to concurrent access to the cache: If the cache is accessed by multiple threads at the same time, you need to consider the concurrency control of the cache.
- Clear the cache regularly: Cleaning the cache regularly can prevent the data in the cache from becoming outdated.
Conclusion
Guava cache is a high-performance memory cache that can significantly improve application performance. Through reasonable use of Guava cache, the performance and scalability of the application can be effectively improved.
The above is the detailed content of Application tips for decrypting Guava cache: an artifact to improve application performance. For more information, please follow other related articles on the PHP Chinese website!
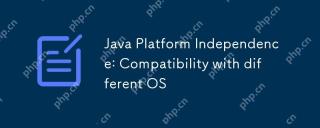
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
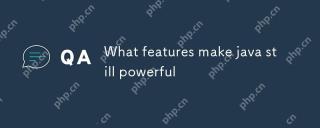
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
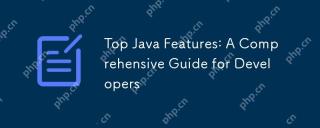
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
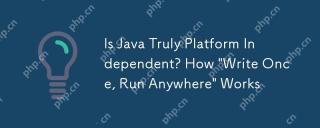
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
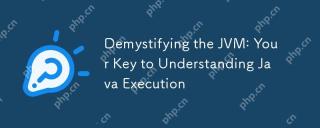
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
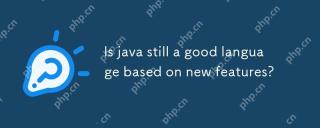
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
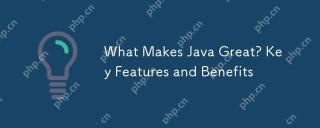
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
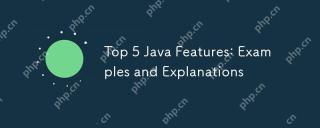
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
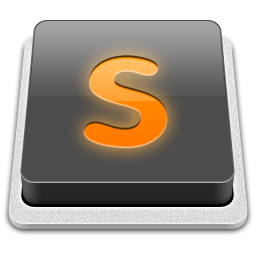
SublimeText3 Mac version
God-level code editing software (SublimeText3)
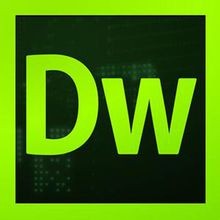
Dreamweaver CS6
Visual web development tools
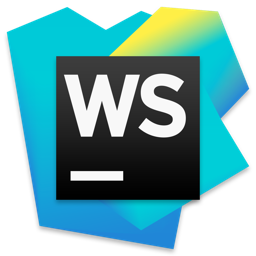
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
