


Go language crawler project development guide: sharing of practical experience and practical skills
Practical Guide: Sharing practical experience in developing crawler projects using Go language
Introduction: With the development of the Internet, the era of information explosion has arrived. In this information age, we often need to obtain various data from the Internet, and crawlers are a very effective way. This article will share practical experience in developing crawler projects using Go language and provide specific code examples.
1. Introduction to Go language
Go language is a programming language developed by Google. It combines the safety of statically typed languages and the convenience of dynamically typed languages. The Go language has an efficient concurrency mechanism and excellent performance, making it one of the preferred languages for developing crawler projects.
2. The basic process of developing a crawler project in Go language
-
Send an HTTP request: Use the http package of the Go language to send an HTTP request to obtain the web page content.
package main import ( "fmt" "io/ioutil" "net/http" ) func getHTML(url string) (string, error) { resp, err := http.Get(url) if err != nil { return "", err } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { return "", err } return string(body), nil } func main() { url := "https://www.example.com" html, err := getHTML(url) if err != nil { fmt.Println("Error:", err) return } fmt.Println(html) }
-
Parse web page content: Use the html package in the standard library of Go language to parse web page content and extract the required data.
package main import ( "fmt" "golang.org/x/net/html" "io/ioutil" "net/http" "strings" ) func getHTML(url string) (string, error) { resp, err := http.Get(url) if err != nil { return "", err } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { return "", err } return string(body), nil } func parseHTML(html string) { doc, err := html.Parse(strings.NewReader(html)) if err != nil { fmt.Println("Error:", err) return } var parse func(n *html.Node) parse = func(n *html.Node) { if n.Type == html.ElementNode && n.Data == "a" { for _, a := range n.Attr { if a.Key == "href" { fmt.Println(a.Val) } } } for c := n.FirstChild; c != nil; c = c.NextSibling { parse(c) } } parse(doc) } func main() { url := "https://www.example.com" html, err := getHTML(url) if err != nil { fmt.Println("Error:", err) return } parseHTML(html) }
-
Store data: Store the parsed data in a file or database.
package main import ( "encoding/csv" "fmt" "golang.org/x/net/html" "io/ioutil" "net/http" "os" "strings" ) func getHTML(url string) (string, error) { resp, err := http.Get(url) if err != nil { return "", err } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { return "", err } return string(body), nil } func parseHTML(html string) []string { doc, err := html.Parse(strings.NewReader(html)) if err != nil { fmt.Println("Error:", err) return nil } var links []string var parse func(n *html.Node) parse = func(n *html.Node) { if n.Type == html.ElementNode && n.Data == "a" { for _, a := range n.Attr { if a.Key == "href" { links = append(links, a.Val) } } } for c := n.FirstChild; c != nil; c = c.NextSibling { parse(c) } } parse(doc) return links } func saveData(links []string) { file, err := os.Create("links.csv") if err != nil { fmt.Println("Error:", err) return } defer file.Close() writer := csv.NewWriter(file) defer writer.Flush() for _, link := range links { writer.Write([]string{link}) } } func main() { url := "https://www.example.com" html, err := getHTML(url) if err != nil { fmt.Println("Error:", err) return } links := parseHTML(html) saveData(links) fmt.Println("Data saved successfully!") }
3. Things to note when developing crawler projects using Go language
- Use an appropriate concurrency model: Since crawler projects need to handle a large number of requests at the same time, use A suitable concurrency model can improve efficiency. The goroutine and channel mechanisms of the Go language can easily implement concurrent programming and make full use of the performance advantages of multi-core processors.
- Set an appropriate delay: In order to avoid excessive pressure on the website being crawled, an appropriate delay should be set to avoid being blocked by the target website.
- Add exception handling: In crawler projects, you often encounter some unexpected errors, such as network connection interruption, parsing errors, etc. In order to improve the robustness of the program, appropriate exception handling should be added.
- Comply with the website’s crawler rules: During the process of crawling web pages, you should abide by the website’s crawler rules to avoid infringing on the rights of others.
Conclusion: Using Go language to develop crawler projects can efficiently and quickly obtain data on the Internet. Through the practical experience sharing and specific code examples in this article, we hope to help readers better develop Go language crawler projects and improve the efficiency of data acquisition. At the same time, during the development of crawler projects, you must abide by laws, regulations and ethics, and protect the rights and interests of others.
The above is the detailed content of Go language crawler project development guide: sharing of practical experience and practical skills. For more information, please follow other related articles on the PHP Chinese website!
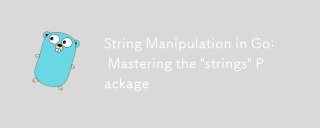
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
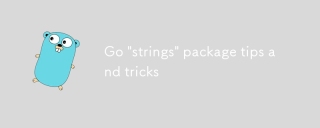
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
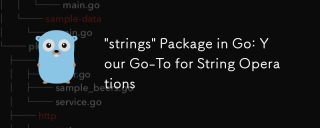
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
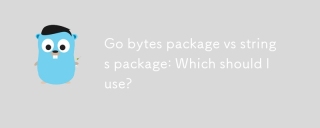
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
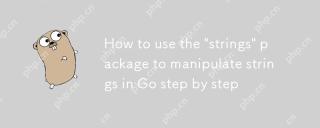
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
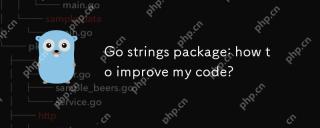
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
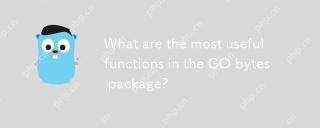
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
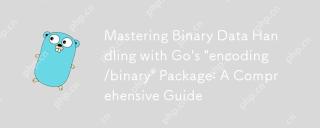
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
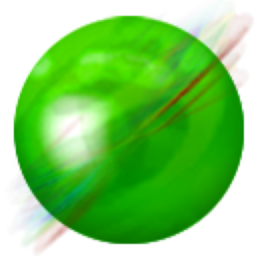
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
