Detailed explanation of the simplest implementation method of Java bubble sort
Bubble sort is a simple sorting algorithm that repeatedly exchanges two adjacent elements. Move the largest element gradually to the right to the correct position. In this article, we will analyze in detail the simplest implementation method of Java bubble sort and give specific code examples.
First, let us understand the basic idea of bubble sort. The process of bubble sorting can be described as: starting from the first element of the array, comparing two adjacent elements, and if the former element is larger than the latter element, swap their positions. After this round, the largest element will be moved to the last position of the array. Then, sort the remaining elements in the same way. Repeat this process until the entire array is sorted.
In Java, we can implement bubble sort through nested loops. The outer loop controls the number of rounds of comparison, and each round of comparison moves an element to the correct position. The inner loop is used to compare two adjacent elements and exchange them. Here is a simple bubble sort code example:
public class BubbleSort { public static void bubbleSort(int[] arr) { int n = arr.length; for (int i = 0; i < n-1; i++) { for (int j = 0; j < n-i-1; j++) { if (arr[j] > arr[j+1]) { // 交换arr[j]和arr[j+1]的位置 int temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } } } } public static void main(String[] args) { int[] arr = {64, 34, 25, 12, 22, 11, 90}; bubbleSort(arr); System.out.println("排序后的数组:"); for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } } }
In this example, we first define a bubbleSort
method that accepts an integer array as a parameter and passes the bubble sort Bubble sort implements sorting of arrays. Then, we create an array of integers in the main
method and call the bubbleSort
method to sort it. Finally, we print the sorted array using a loop.
Run the above code, the output result is:
排序后的数组: 11 12 22 25 34 64 90
As can be seen from the result, bubble sort successfully arranges the elements in the array in ascending order.
It should be noted that the time complexity of bubble sort is O(n^2), where n is the length of the array. This means that the performance of bubble sort may be poor when there are many elements to be sorted. Therefore, in practical applications, more complex sorting algorithms, such as quick sort or merge sort, tend to better meet the needs.
To summarize, this article analyzes in detail the simplest implementation method of Java bubble sorting and gives specific code examples. I hope that readers can better understand the principle and implementation of bubble sort through this article, as well as the practical application scenarios of bubble sort. At the same time, we also hope that readers can choose a suitable sorting algorithm to solve the problem according to specific needs.
The above is the detailed content of Simple and easy to understand Java bubble sort implementation method analysis. For more information, please follow other related articles on the PHP Chinese website!
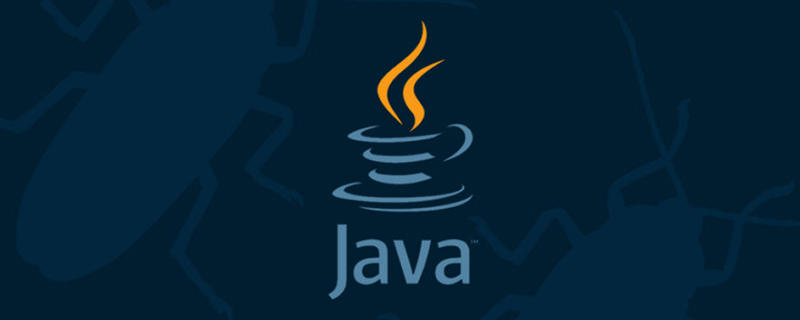
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
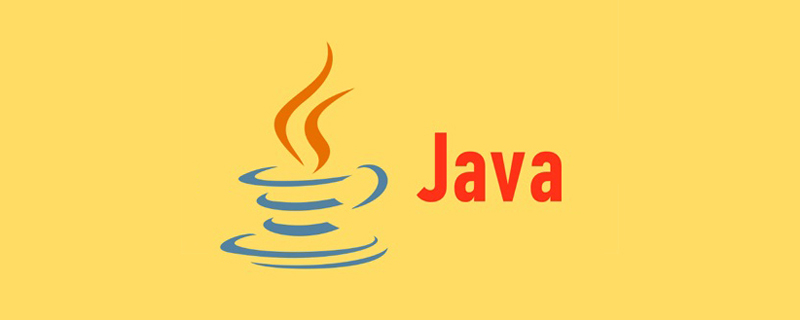
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
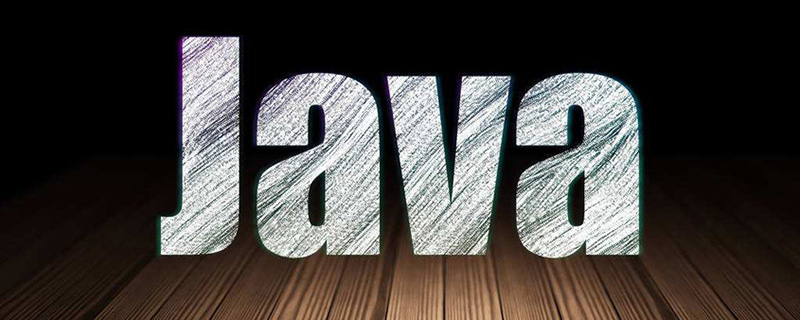
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
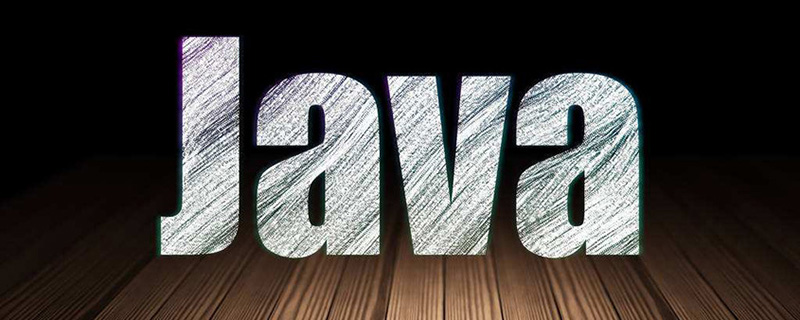
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
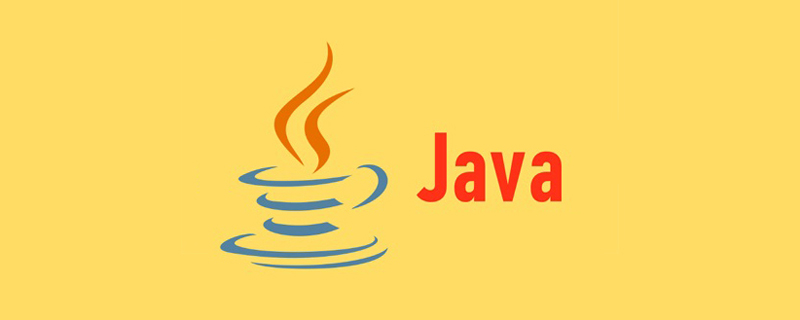
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
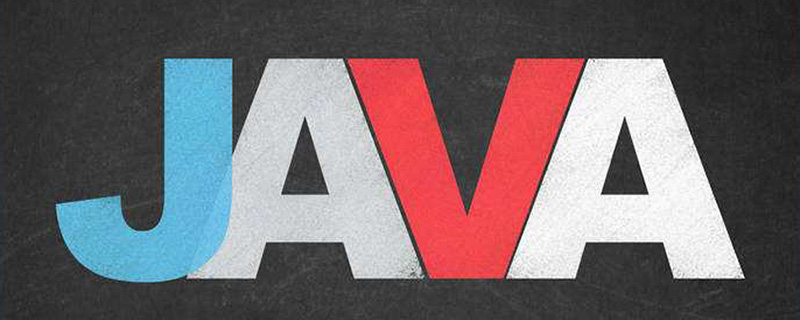
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
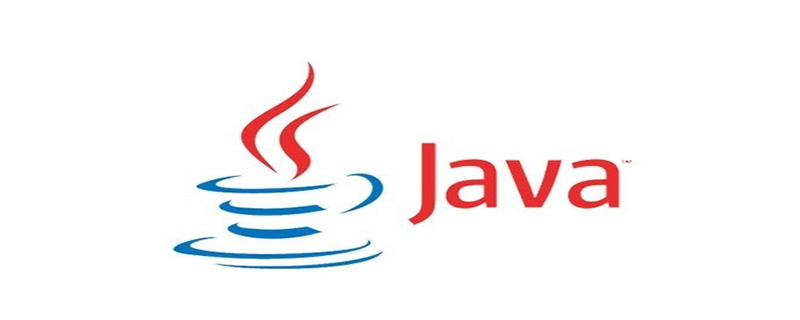
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
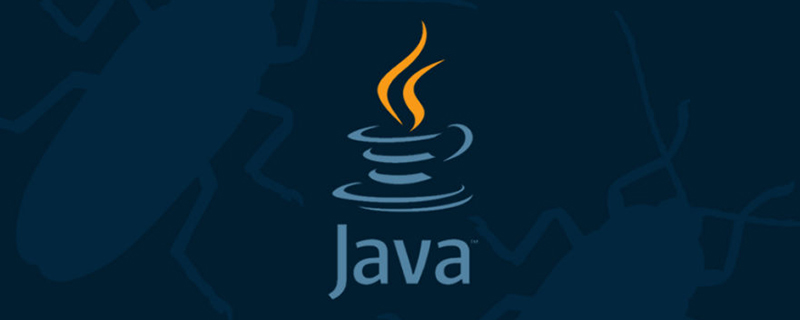
本篇文章给大家带来了关于Java的相关知识,其中主要整理了Stream流的概念和使用的相关问题,包括了Stream流的概念、Stream流的获取、Stream流的常用方法等等内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
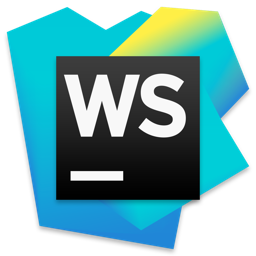
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
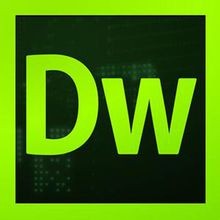
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.