Analysis of the simplest implementation steps of Java bubble sort
Bubble sort is a simple and intuitive sorting algorithm that compares and exchanges between adjacent elements To gradually "bubble" the largest (or smallest) elements to one end of the sequence. This article will analyze in detail the simplest implementation steps of Java bubble sort and provide specific code examples.
Step 1: Define the array and array length
First, we need to define an array to be sorted and record the length of the array. Suppose our array is arr with length n.
Step 2: Implement sorting loop
The core of bubble sorting is to achieve sorting through the comparison and exchange of adjacent elements. We need to use two nested loops to implement the sorting process. The outer loop controls how many rounds of comparison and exchange are required, while the inner loop is used to perform specific element comparison and exchange operations.
Step 3: Compare adjacent elements
In each round of comparison, we need to start from the first element of the array and compare the sizes of the two adjacent elements in sequence. If adjacent elements are not in the correct order (for example, the first element is larger than the second element), the positions of the two elements need to be swapped to ensure that the larger element "bubbles" to the later position.
Step 4: Continue comparison and exchange
After a round of comparison and exchange, the largest element has "bubbled" to the last bit of the array. Next, we need to continue with the next round of comparisons and swaps, but this time we only need to consider the remaining n-1 elements. Similarly, we need to compare the sizes of adjacent elements and perform swap operations.
Step 5: Repeat the operation
We need to repeat steps 3 and 4 until the entire array is sorted. Each round of comparison and exchange operations will "bubble" the largest element to the end of the array, so we need a total of n-1 rounds of comparison and exchange.
Step 6: Output the sorting results
When all comparison and exchange operations are completed, we can output the final sorting results. At this time, the elements in the array have been arranged in ascending order.
The following is a specific Java code example:
public class BubbleSort { public static void main(String[] args) { int[] arr = {5, 2, 8, 4, 1}; int n = arr.length; // 外层循环控制比较和交换的轮数 for (int i = 0; i < n - 1; i++) { // 内层循环进行具体的比较和交换操作 for (int j = 0; j < n - i - 1; j++) { // 比较相邻元素的大小 if (arr[j] > arr[j + 1]) { // 交换两个元素的位置 int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } // 输出排序结果 System.out.print("排序结果:"); for (int item: arr) { System.out.print(item + " "); } } }
In the above code, we first define an array arr to be sorted and the length n of the array. Then, the comparison and exchange operations of bubble sort are implemented through nested loops. Finally, the sorting results are output.
The time complexity of the bubble sort algorithm is O(n^2) and is rarely used in practical applications. However, as a simple sorting algorithm, it can help us understand the basic ideas and Implementation process.
The above is the detailed content of Analyze the simplest implementation steps of Java bubble sort algorithm. For more information, please follow other related articles on the PHP Chinese website!
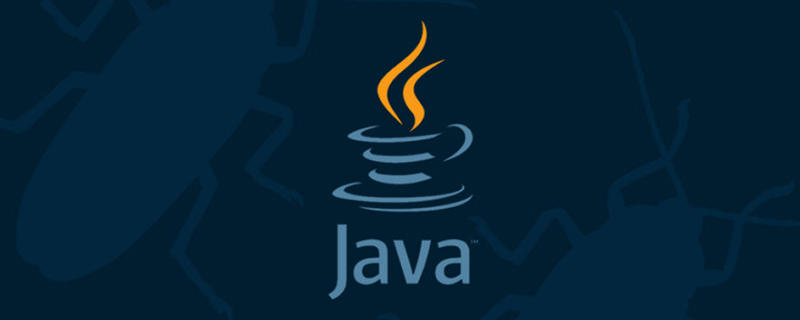
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
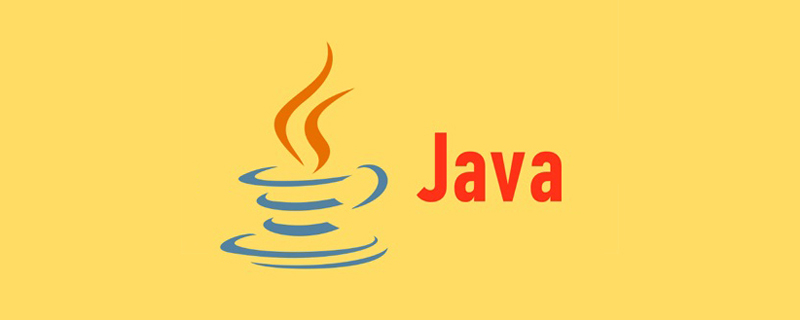
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
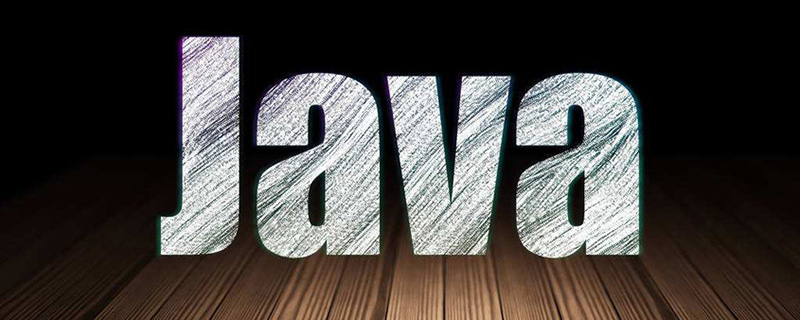
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
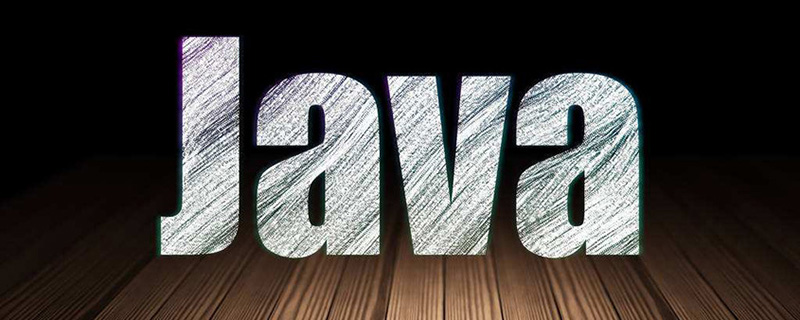
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
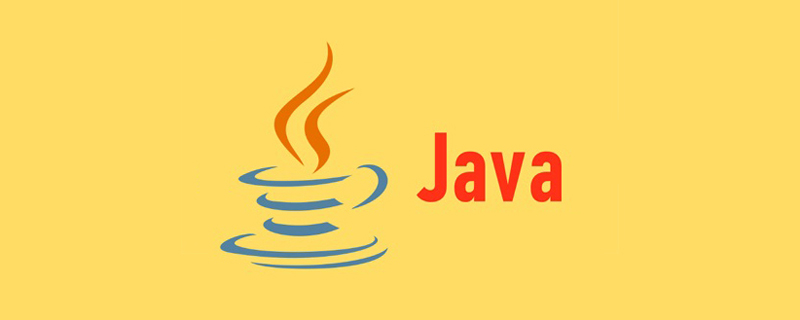
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
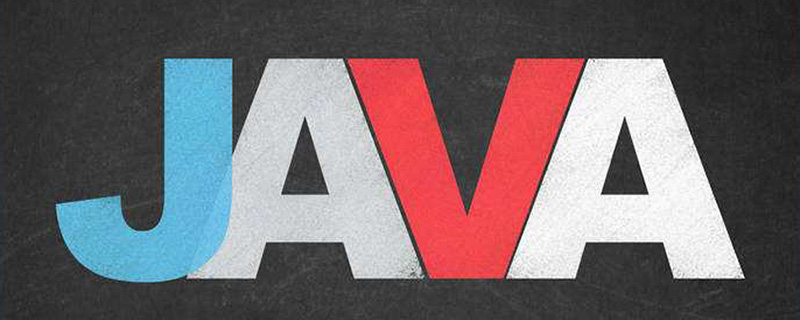
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
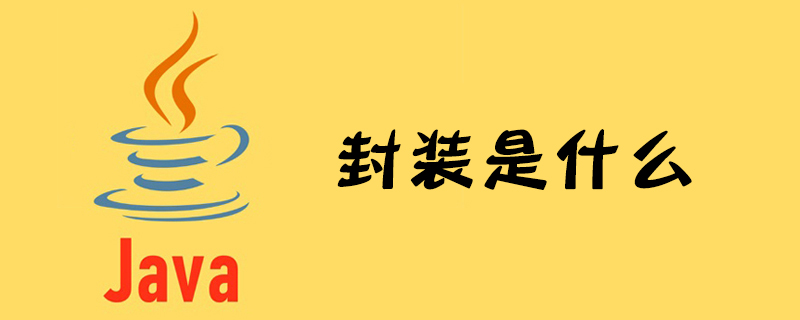
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
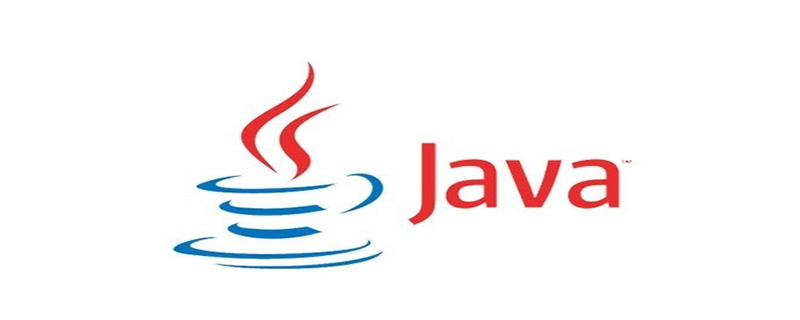
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
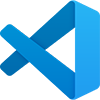
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
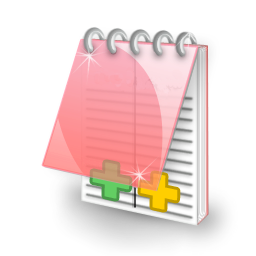
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
