What are the practical applications of the XOR notation in Golang?
What exactly does the XOR operator in Golang do?
The XOR symbol (^) is a binary operator in Golang, used to perform bitwise XOR operation on two binary numbers. In Golang, the XOR operator ^ can be used for integer types (including signed and unsigned integer types) as well as Boolean types. The XOR operator is widely used in computer science. This article will introduce in detail the specific role of the XOR operator in Golang and provide corresponding code examples.
- Bitwise operation
XOR operator ^ is used in Golang to perform bitwise XOR operation on two binary numbers. The bitwise XOR operation compares each bit of the two operands. If the bits at the corresponding positions are the same, the corresponding bits in the result are 0; if the bits at the corresponding positions are different, the corresponding bits in the result are 1.
Here is a simple example that demonstrates how to use the XOR operator to perform a bitwise XOR operation on two integers:
package main import "fmt" func main() { var a uint8 = 5 // 二进制表示为 00000101 var b uint8 = 3 // 二进制表示为 00000011 result := a ^ b // 结果为二进制 00000110,十进制为 6 fmt.Println(result) }
- Negation operation
The XOR operator^ can also be used to negate a binary number. This is because when a binary number is XORed with another binary number, the result is 0 if the corresponding bits of the two binary numbers are the same, and 1 if the corresponding bits are different. Therefore, if a binary number is XORed with a binary number that is all ones, the inversion operation can be achieved.
Here is an example that demonstrates how to use the XOR operator to negate the operation:
package main import "fmt" func main() { var a uint8 = 10 // 二进制表示为 00001010 var b uint8 = 255 // 二进制表示为 11111111 result := a ^ b // 结果为二进制 11110101,十进制为 245 fmt.Println(result) }
- Exchange values
The XOR operator^ can also be used To exchange the values of two variables. This is because the XOR operator is reflexive and transitive. By bitwise XORing two variables, you can swap their values without using intermediate variables.
The following is an example that demonstrates how to use the XOR operator to exchange the values of two integer variables:
package main import "fmt" func main() { var a, b uint8 = 5, 10 a = a ^ b // a 等于 a 和 b 的异或结果 b = a ^ b // b 等于 (a 和 b 的异或结果) 和 b 的异或结果,即 a a = a ^ b // a 等于 (a 和 b 的异或结果) 和 (a 和 b 的异或结果) 的异或结果,即 b fmt.Println(a, b) // 输出 10 5 }
Through the above example, we can see that the values of variables a and b The values have been successfully exchanged.
Summary:
XOR operator^ has a variety of application scenarios in Golang. It can be used for bitwise operations. It performs bitwise XOR operations on two binary numbers. It can perform inversion operations. The inversion operation can be achieved by XORing a binary number with a binary number of all 1s. It can also perform the inversion operation through XOR. The operator exchanges the values of two variables without using an intermediate variable. These application scenarios make the XOR operator ^ one of the important and practical operators in Golang.
The above is a detailed introduction to the XOR operator in Golang and the corresponding code examples. I hope it can be helpful to readers.
The above is the detailed content of What are the practical applications of the XOR notation in Golang?. For more information, please follow other related articles on the PHP Chinese website!
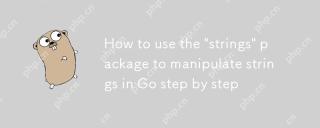
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
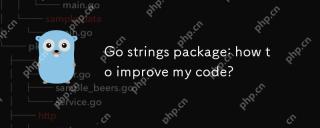
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
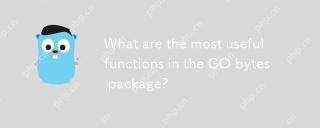
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
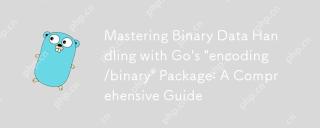
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
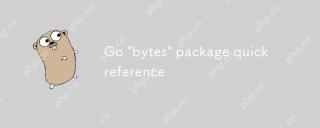
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
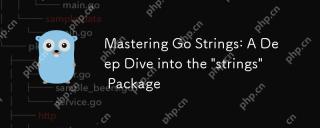
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
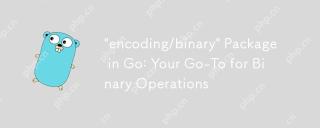
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
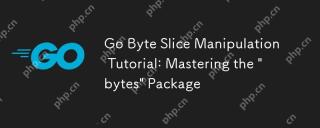
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
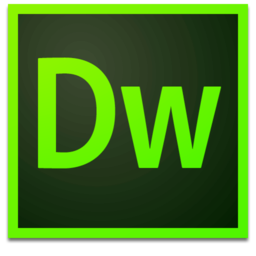
Dreamweaver Mac version
Visual web development tools
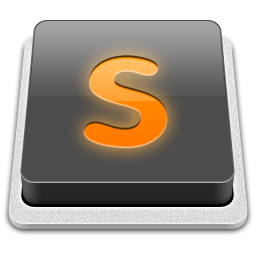
SublimeText3 Mac version
God-level code editing software (SublimeText3)
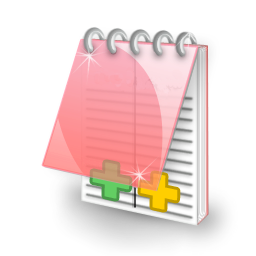
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
