How to perform rounding operation in Golang division operation requires specific code examples
In Go language, the division operator/
is used for calculation The quotient of two numbers. But sometimes we need to round the result to get the integer part or the decimal part with a specific precision. This article will introduce how to perform rounding operations in Golang and provide specific code examples.
Method 1: Use forced type conversion
package main import ( "fmt" ) func main() { dividend := 10 divisor := 3 quotient := float64(dividend) / float64(divisor) result := int(quotient) fmt.Println(result) // 输出结果为3 }
In the above example, we first convert the dividend and divisor to floating point types respectively, and then perform the division operation to obtain a quotient of floating point type. Next, we cast this floating point number to the integer type int
to get the rounded result.
Method 2: Use the function in the math package
package main import ( "fmt" "math" ) func main() { dividend := 10 divisor := 3 result := math.Floor(float64(dividend) / float64(divisor)) fmt.Println(int(result)) // 输出结果为3 }
In this example, we use the Floor
function in the math package to round down. First, the dividend and divisor are converted to floating point number types, and then the division operation is performed to obtain the quotient. Then, this floating point number is rounded down through the Floor
function. The returned result is still a floating point number, so we need to convert it to an integer type.
In addition to the Floor
function, there is also the Ceil
function that can be used for rounding up, and the Round
function that can be used for rounding. You can choose the appropriate function to perform rounding operations according to the specific situation.
Summary:
The methods for performing division operations and rounding operations in Golang mainly include forced type conversion and functions in the math package. Casting is suitable for simple rounding operations, while the functions in the math package provide more choices for rounding methods. Just choose the appropriate method according to your specific needs. The above code examples are for reference only, and the specific implementation can be adjusted according to the actual situation.
The above is the detailed content of How to perform division and rounding operations in Golang. For more information, please follow other related articles on the PHP Chinese website!
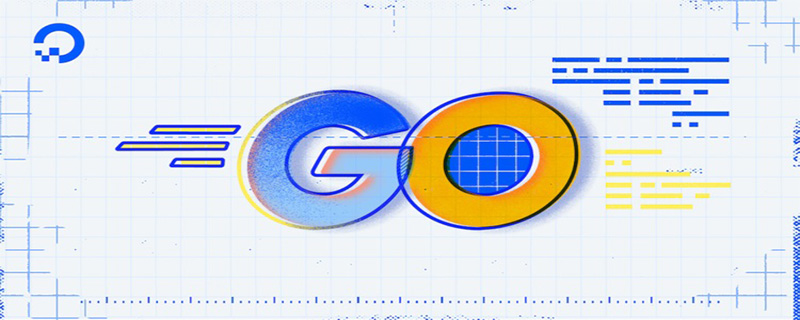
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
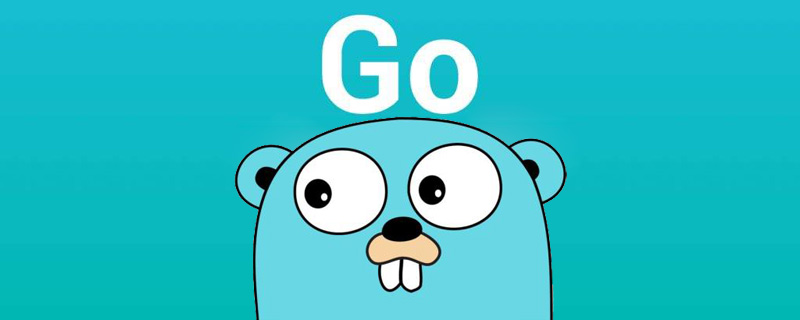
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
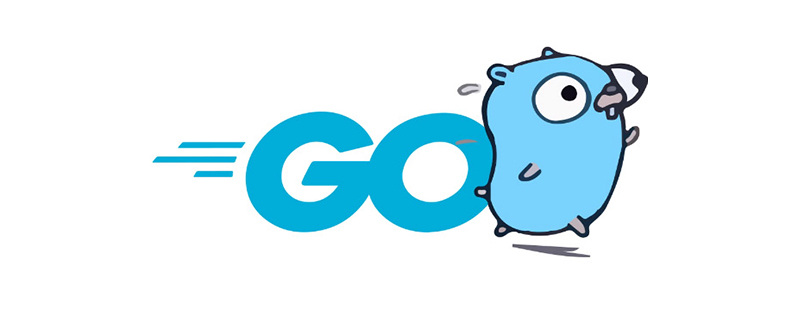
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
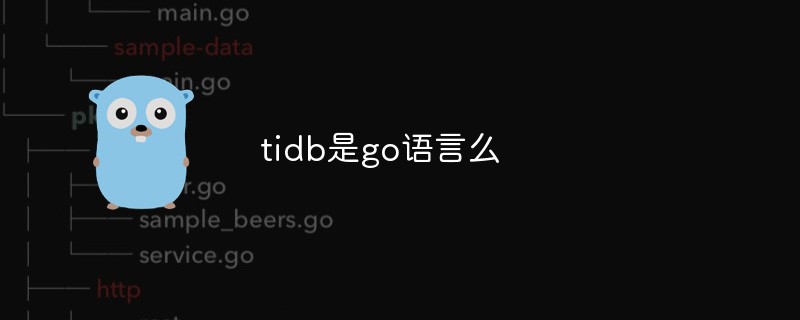
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
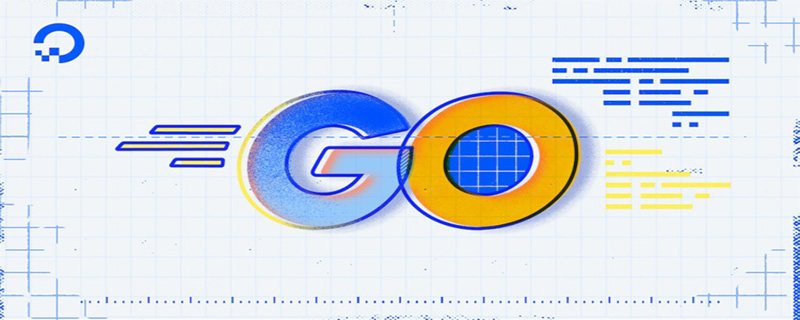
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
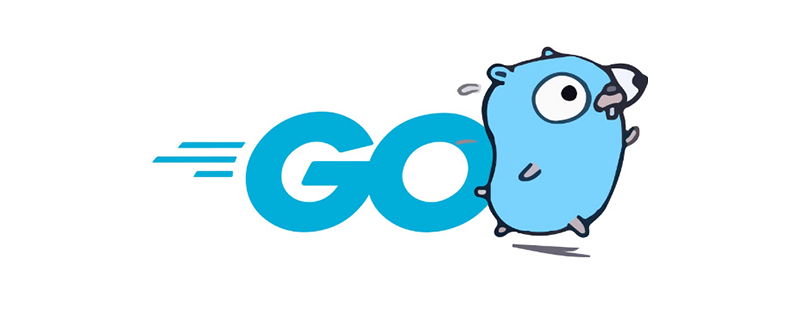
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
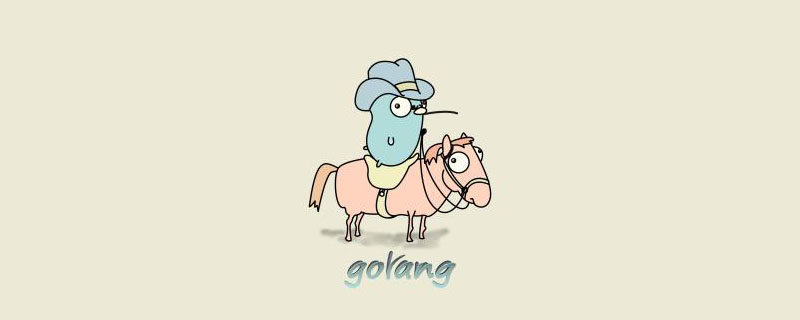
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
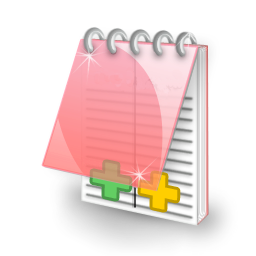
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
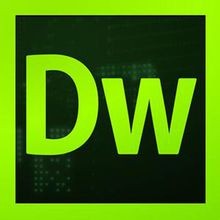
Dreamweaver CS6
Visual web development tools
