


Learn the basic knowledge and skills of HTML responsive layout, starting from scratch
Learn the basic knowledge and skills of HTML responsive layout
With the popularity of mobile devices, responsive layout has become an essential skill for designing and developing websites. Responsive layout allows the website to automatically adjust layout and display effects under different screen sizes, providing a better user experience. This article will introduce how to learn the basic knowledge and skills of HTML responsive layout from scratch, and provide specific code examples.
1. HTML media queries
Media queries are one of the cornerstones of responsive layout. It can apply different style sheets based on the device's screen size, orientation, resolution and other characteristics. Media queries are defined using the @media rule. Here is a simple media query example:
@media screen and (max-width: 600px) {
body {
background-color: lightblue;
}
}
The meaning of this code is to set the background color of the body to light blue when the screen width is less than or equal to 600 pixels. Various CSS properties and values can be used in media queries to achieve complex layout adjustments.
2. Fluid layout
Fluid layout is a common responsive layout mode, which automatically adjusts the size and order of web page content according to the screen width. In a fluid layout, the width of an element is generally a percentage relative to its parent element. The following is a simple fluid layout example:
<div class="container"> <div class="box">Box 1</div> <div class="box">Box 2</div> <div class="box">Box 3</div> </div> <style> .container { width: 100%; display: flex; flex-wrap: wrap; } .box { width: 33.33%; background-color: lightgray; padding: 10px; box-sizing: border-box; } @media screen and (max-width: 600px) { .box { width: 50%; } } </style>
In the above code, the container element uses flex layout, and the box elements are arranged according to percentages. When the screen width is less than or equal to 600 pixels, the width of the box element is adjusted to 50% through media queries.
3. Elastic Grid Layout
Elastic grid layout is a more advanced responsive layout mode that uses CSS grid layout features to achieve automatic adjustment of multiple columns. Flexible grid layout automatically adjusts the grid's column count and size based on the screen width and element size. The following is a simple elastic grid layout example:
<div class="container"> <div class="box">Box 1</div> <div class="box">Box 2</div> <div class="box">Box 3</div> </div> <style> .container { display: grid; grid-template-columns: repeat(auto-fit, minmax(200px, 1fr)); grid-gap: 10px; } .box { background-color: lightgray; padding: 10px; box-sizing: border-box; } </style>
In the above code, the container element uses grid layout and defines the minimum and maximum width of each column through the grid-template-columns attribute. Through the repeat function and the auto-fit keyword, the effect of automatically adjusting the number of columns can be achieved.
4. CSS Framework
In addition to manually writing HTML and CSS code, you can also use some ready-made CSS frameworks to simplify the development of responsive layout. Commonly used CSS frameworks include Bootstrap, Foundation, etc. These frameworks provide a rich set of components and styles that help quickly build responsive layouts. The following is an example using the Bootstrap framework:
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css"> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-6 col-md-4">Box 1</div> <div class="col-sm-6 col-md-4">Box 2</div> <div class="col-sm-6 col-md-4">Box 3</div> </div> </div> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/js/bootstrap.bundle.min.js"></script> </body> </html>
In the above code, Bootstrap's grid system is used to implement responsive layout. Through the col class and different screen breakpoints, you can define the width and arrangement of elements under different screen sizes.
Summary:
Learning HTML responsive layout from scratch requires mastering basic knowledge and skills such as media queries, fluid layout, and elastic grid layout. Through continuous practice and experimentation, you can deepen your understanding of responsive layout and improve your layout capabilities. In addition, using CSS frameworks can speed up development and improve efficiency. I hope the code examples provided in this article can help readers better learn and practice HTML responsive layout.
The above is the detailed content of Learn the basic knowledge and skills of HTML responsive layout, starting from scratch. For more information, please follow other related articles on the PHP Chinese website!

HTML is the cornerstone of building web page structure. 1. HTML defines the content structure and semantics, and uses, etc. tags. 2. Provide semantic markers, such as, etc., to improve SEO effect. 3. To realize user interaction through tags, pay attention to form verification. 4. Use advanced elements such as, combined with JavaScript to achieve dynamic effects. 5. Common errors include unclosed labels and unquoted attribute values, and verification tools are required. 6. Optimization strategies include reducing HTTP requests, compressing HTML, using semantic tags, etc.

HTML is a language used to build web pages, defining web page structure and content through tags and attributes. 1) HTML organizes document structure through tags, such as,. 2) The browser parses HTML to build the DOM and renders the web page. 3) New features of HTML5, such as, enhance multimedia functions. 4) Common errors include unclosed labels and unquoted attribute values. 5) Optimization suggestions include using semantic tags and reducing file size.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.

The role of HTML is to define the structure and content of a web page through tags and attributes. 1. HTML organizes content through tags such as , making it easy to read and understand. 2. Use semantic tags such as, etc. to enhance accessibility and SEO. 3. Optimizing HTML code can improve web page loading speed and user experience.

HTMLisaspecifictypeofcodefocusedonstructuringwebcontent,while"code"broadlyincludeslanguageslikeJavaScriptandPythonforfunctionality.1)HTMLdefineswebpagestructureusingtags.2)"Code"encompassesawiderrangeoflanguagesforlogicandinteract

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
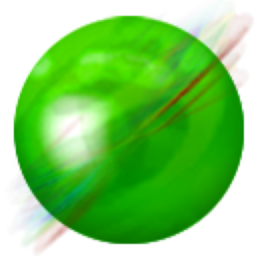
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use
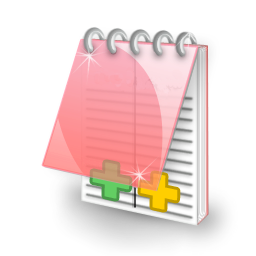
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function