There are three methods for converting arrays to List in Java: "asList(static method", "for loop traversal", and "Java 8's Stream API": 1. Use the asList() static method of the Arrays class , returns a fixed-length List, which cannot be added or deleted; 2. Use a for loop to traverse the array and add it to the List one by one; 3. Use the Stream API introduced in Java 8.
In Java, you can use the following methods to convert an array to a List:
- Use the asList() static method of the Arrays class
String[] array = {"apple", "banana", "orange"}; List<string> list = Arrays.asList(array);</string>
This method is the most commonly used one and can quickly convert an array into a List. It should be noted that the asList() method returns a fixed-length List and cannot be added or deleted. .If you need to modify the List, you can do so by creating a new ArrayList object:
String[] array = {"apple", "banana", "orange"}; List<string> list = new ArrayList(Arrays.asList(array));</string>
- Use a for loop to traverse the array and add it to the List one by one
String[] array = {"apple", "banana", "orange"}; List<string> list = new ArrayList(); for (String item : array) { list.add(item); }</string>
This method is more cumbersome, but it can flexibly control the order and conditions of addition.
- Using the Stream API of Java 8
String[] array = {"apple", "banana", "orange"}; List<string> list = Arrays.stream(array).collect(Collectors.toList());</string>
This method uses the Stream API introduced by Java 8 The Stream API can easily complete the conversion from an array to a List, but it should be noted that the array must be converted to a Stream object before the Collectors.toList() method can be used.
The above is the detailed content of What are the methods to convert array to List in Java?. For more information, please follow other related articles on the PHP Chinese website!
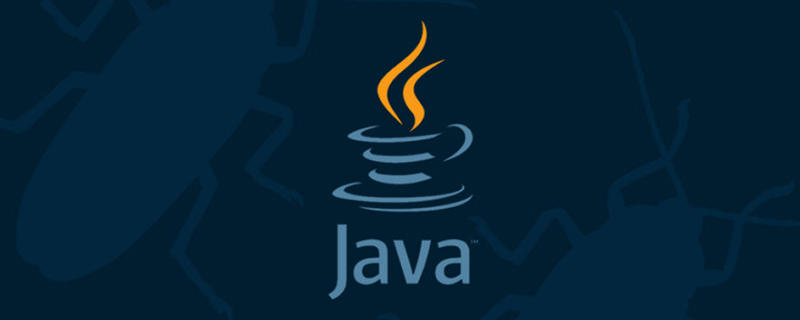
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
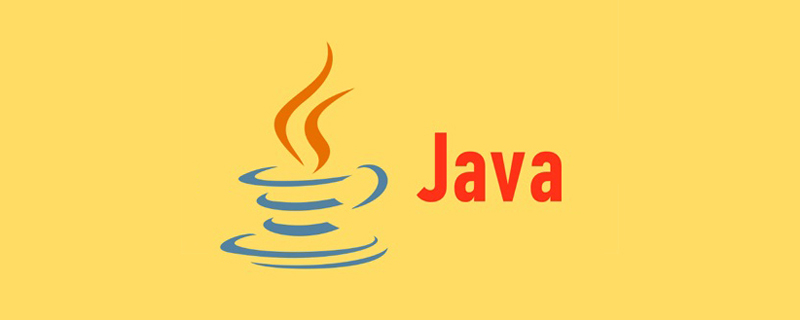
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
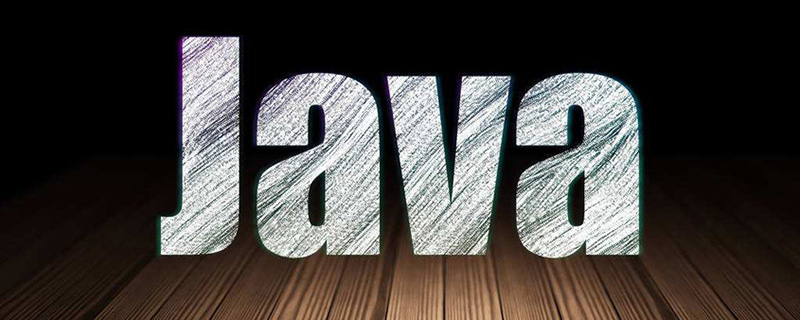
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
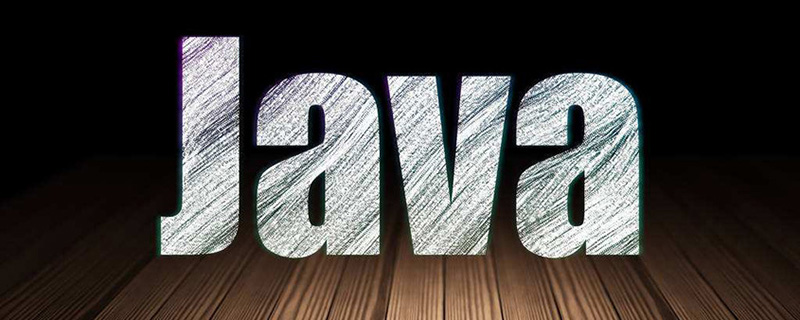
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
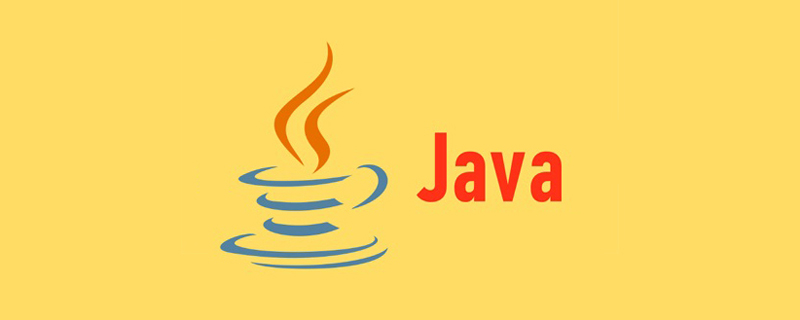
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
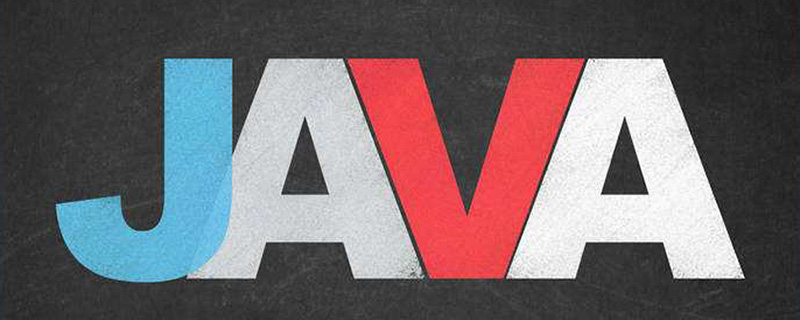
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
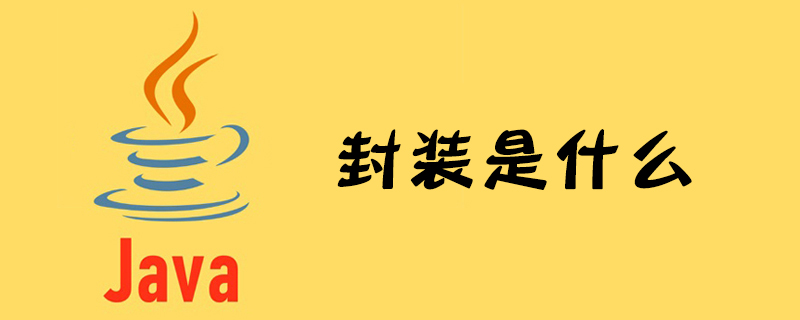
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
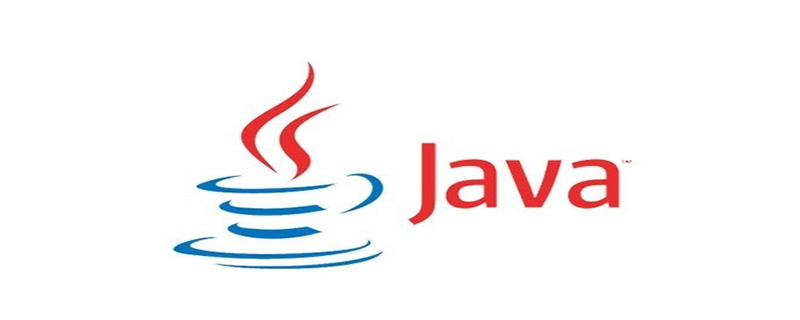
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
