Can the expiration time of Ajax request be customized?
In web development, we often use Ajax to implement asynchronous requests to dynamically load data in the page. When making Ajax requests, sometimes we need to control the timeout of the request, that is, set a time limit, and process it if no response is received within the specified time. So, can the expiration time of Ajax requests be customized? This article will introduce this problem in detail and provide specific code examples.
When using jQuery's Ajax function to make a request, we can customize the expiration time of the request by setting the timeout attribute. By default, the value of the timeout attribute is 0, that is, there is no timeout limit. If we need to set the timeout to 1 second, we can set the timeout value to 1000, as shown below:
$.ajax({ url: "example.php", timeout: 1000, success: function(data) { // 请求成功的处理逻辑 }, error: function(xhr, textStatus, errorThrown) { // 请求失败的处理逻辑 } });
In this example, we set the timeout to 1 second. If the request takes more than 1 second, the error callback function will be triggered.
In addition to using jQuery's Ajax function, we can also use the native XMLHttpRequest object to send Ajax requests and set a custom timeout. Here is a sample code:
var xhr = new XMLHttpRequest(); xhr.open("GET","example.php",true); xhr.timeout = 1000; xhr.onload = function() { if (xhr.status === 200) { // 请求成功的处理逻辑 } else { // 请求失败的处理逻辑 } }; xhr.ontimeout = function() { // 请求超时的处理逻辑 }; xhr.send();
In this example, we customize the timeout by setting the timeout property to 1000. When the request time exceeds 1 second, the ontimeout callback function will be triggered.
It should be noted that using timeout to set the timeout is not absolutely accurate. Because Ajax requests are asynchronous, it depends on the network environment and the server's response time. So, even if you set a short timeout, the request may still time out if the server takes too long to respond.
To summarize, the expiration time of Ajax requests can be customized. By setting the timeout attribute, we can control the timeout period of the request. This function can be easily implemented whether using jQuery's Ajax function or the native XMLHttpRequest object. However, it should be noted that the timeout is not absolutely accurate and depends on the network environment and server response time. During actual development, an appropriate timeout should be set according to specific circumstances to ensure user experience and system stability.
(Note: The url and example.php in the code examples provided in this article are for demonstration purposes only and need to be modified according to the actual situation)
The above is the detailed content of Can I customize the expiration time of Ajax requests?. For more information, please follow other related articles on the PHP Chinese website!
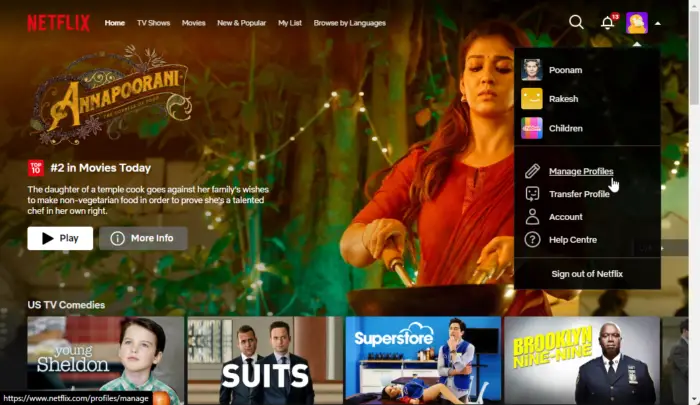
Netflix上的头像是你流媒体身份的可视化代表。用户可以超越默认的头像来展示自己的个性。继续阅读这篇文章,了解如何在Netflix应用程序中设置自定义个人资料图片。如何在Netflix中快速设置自定义头像在Netflix中,没有内置功能来设置个人资料图片。不过,您可以通过在浏览器上安装Netflix扩展来实现此目的。首先,在浏览器上安装Netflix扩展的自定义个人资料图片。你可以在Chrome商店买到它。安装扩展后,在浏览器上打开Netflix并登录您的帐户。导航至右上角的个人资料,然后单击
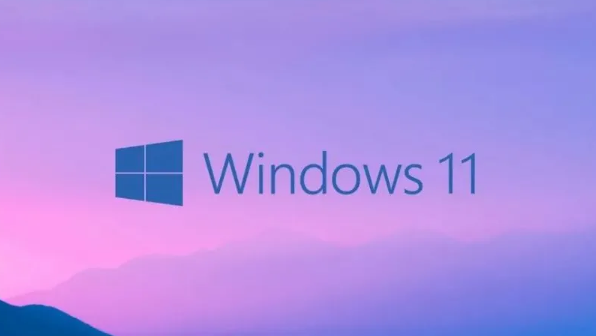
Win11如何自定义背景图片?在最新发布的win11系统中,里面有许多的自定义功能,但是很多小伙伴不知道应该如何使用这些功能。就有小伙伴觉得背景图片比较单调,想要自定义背景图,但是不知道如何操作自定义背景图,如果你不知道如何定义背景图片,小编下面整理了Win11自定义背景图片步骤,感兴趣的话一起往下看看把!Win11自定义背景图片步骤1、点击桌面win按钮,在弹出的菜单中点击设置,如图所示。2、进入设置菜单,点击个性化,如图所示。3、进入个性化,点击背景,如图所示。4、进入背景设置,点击浏览图片
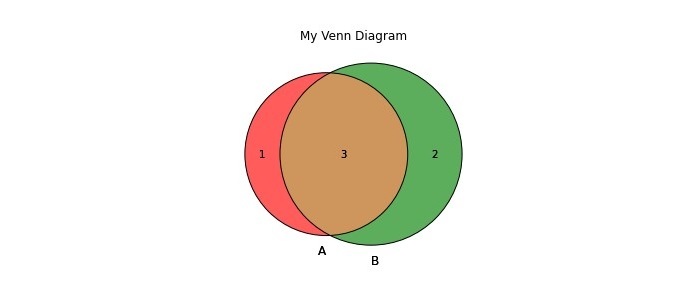
维恩图是用来表示集合之间关系的图。要创建维恩图,我们将使用matplotlib。Matplotlib是一个在Python中常用的数据可视化库,用于创建交互式的图表和图形。它也用于制作交互式的图像和图表。Matplotlib提供了许多函数来自定义图表和图形。在本教程中,我们将举例说明三个示例来自定义Venn图。Example的中文翻译为:示例这是一个创建两个维恩图交集的简单示例;首先,我们导入了必要的库并导入了venns。然后我们将数据集创建为Python集,之后,我们使用“venn2()”函数创
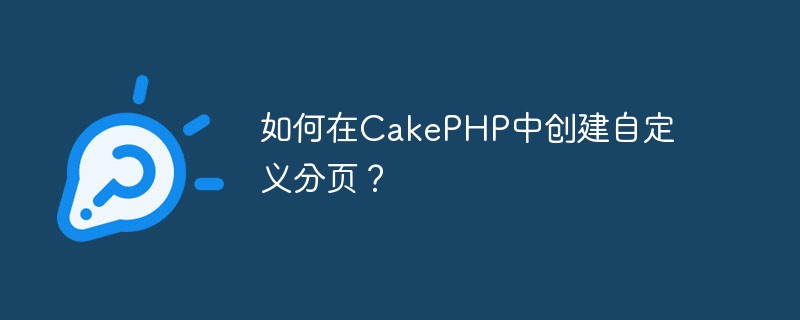
CakePHP是一个强大的PHP框架,为开发人员提供了很多有用的工具和功能。其中之一是分页,它可以帮助我们将大量数据分成几页,从而简化浏览和操作。默认情况下,CakePHP提供了一些基本的分页方法,但有时你可能需要创建一些自定义的分页方法。这篇文章将向您展示如何在CakePHP中创建自定义分页。步骤1:创建自定义分页类首先,我们需要创建一个自定义分页类。这个
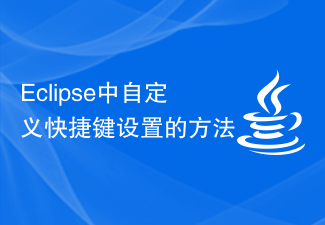
如何在Eclipse中自定义快捷键设置?作为一名开发人员,在使用Eclipse进行编码时,熟练掌握快捷键是提高效率的关键之一。Eclipse作为一款强大的集成开发环境,不仅提供了许多默认的快捷键,还允许用户根据自己的偏好进行个性化的定制。本文将介绍如何在Eclipse中自定义快捷键设置,并给出具体的代码示例。打开Eclipse首先,打开Eclipse,并进入
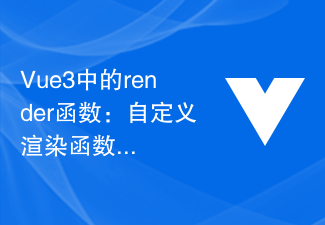
Vue是一款流行的JavaScript框架,它提供了许多方便的功能和API以帮助开发者构建交互式的前端应用程序。随着Vue3的发布,render函数成为了一个重要的更新。本文将介绍Vue3中render函数的概念、用途和如何使用它自定义渲染函数。什么是render函数在Vue中,template是最常用的渲染方式,但是在Vue3中,可以使用另外一种方式:r
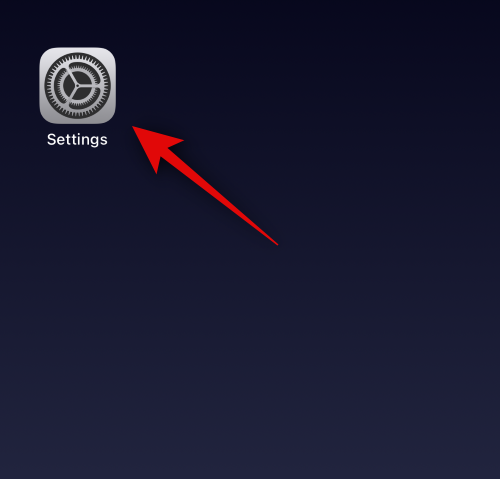
适用于iPhone的iOS17更新为AppleMusic带来了一些重大变化。这包括在播放列表中与其他用户协作,在使用CarPlay时从不同设备启动音乐播放等。这些新功能之一是能够在AppleMusic中使用交叉淡入淡出。这将允许您在曲目之间无缝过渡,这在收听多个曲目时是一个很棒的功能。交叉淡入淡出有助于改善整体聆听体验,确保您在音轨更改时不会受到惊吓或退出体验。因此,如果您想充分利用这项新功能,以下是在iPhone上使用它的方法。如何為AppleMusic啟用和自定Crossfade您需要最新的
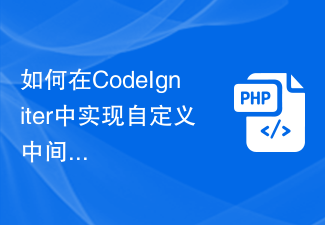
如何在CodeIgniter中实现自定义中间件引言:在现代的Web开发中,中间件在应用程序中起着至关重要的作用。它们可以用来执行在请求到达控制器之前或之后执行一些共享的处理逻辑。CodeIgniter作为一个流行的PHP框架,也支持中间件的使用。本文将介绍如何在CodeIgniter中实现自定义中间件,并提供一个简单的代码示例。中间件概述:中间件是一种在请求


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
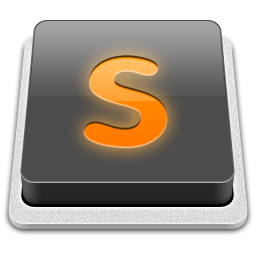
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.