Easy to use NumPy function, specific code examples are required
NumPy is a very commonly used scientific computing library in Python, which provides a wealth of functions and tools to handle arrays and matrices. In this article, we will introduce some commonly used functions in NumPy and how to use them, and demonstrate their functions through specific code examples.
1. Create arrays
Using NumPy can easily create various types of arrays. The following are several common ways to create arrays:
-
Use the numpy.array function to create a one-dimensional array:
import numpy as np a = np.array([1, 2, 3, 4, 5]) print(a)
Output:
[1 2 3 4 5]
-
Use the numpy.zeros function to create an array whose elements are all 0:
b = np.zeros((3, 4)) print(b)
Output:
[[0. 0. 0. 0.] [0. 0. 0. 0.] [0. 0. 0. 0.]]
-
Use the numpy.ones function to create an array whose elements are all 0 Array of 1:
c = np.ones((2, 3)) print(c)
Output:
[[1. 1. 1.] [1. 1. 1.]]
-
Use numpy.eye function to create an identity matrix:
d = np.eye(3) print(d)
Output:
[[1. 0. 0.] [0. 1. 0.] [0. 0. 1.]]
2. Array attributes and basic operations
NumPy arrays have some commonly used attributes and basic operations. Here are some examples:
-
Shape of the array:
print(a.shape) # 输出(5,) print(b.shape) # 输出(3, 4) print(c.shape) # 输出(2, 3) print(d.shape) # 输出(3, 3)
-
Dimensions of the array:
print(a.ndim) # 输出1 print(b.ndim) # 输出2 print(c.ndim) # 输出2 print(d.ndim) # 输出2
-
Number of elements of the array:
print(a.size) # 输出5 print(b.size) # 输出12 print(c.size) # 输出6 print(d.size) # 输出9
-
Data type of array:
print(a.dtype) # 输出int64 print(b.dtype) # 输出float64 print(c.dtype) # 输出float64 print(d.dtype) # 输出float64
3. Array operations
NumPy provides a wealth of array operations. Here are some examples:
-
Addition and subtraction of arrays:
x = np.array([1, 2, 3]) y = np.array([4, 5, 6]) print(x + y) # 输出[5 7 9] print(x - y) # 输出[-3 -3 -3]
-
Multiplication and division of arrays:
print(x * y) # 输出[4 10 18] print(x / y) # 输出[0.25 0.4 0.5 ]
-
Sum of squares of arrays Square root:
print(np.square(x)) # 输出[1 4 9] print(np.sqrt(y)) # 输出[2. 2.236 2.449]
-
Matrix multiplication of arrays:
a = np.array([[1, 2], [3, 4]]) b = np.array([[5, 6], [7, 8]]) print(np.dot(a, b)) # 输出[[19 22] [43 50]]
4. Array indexing and slicing
NumPy provides Powerful features for accessing array elements, here are some examples:
-
Index of array:
a = np.array([1, 2, 3, 4, 5]) print(a[0]) # 输出1 print(a[-1]) # 输出5
-
Slice of array:
b = np.array([[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12]]) print(b[0]) # 输出[1 2 3 4] print(b[:, 0]) # 输出[1 5 9] print(b[1:3, 1:3]) # 输出[[6 7] [10 11]]
5. Array statistical operations
NumPy provides a wealth of array statistical operations. Here are some examples:
-
Calculate the sum of an array , mean and standard deviation:
a = np.array([1, 2, 3, 4, 5]) print(np.sum(a)) # 输出15 print(np.mean(a)) # 输出3.0 print(np.std(a)) # 输出1.41421356
-
Calculate the minimum and maximum values of the array:
b = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print(np.min(b)) # 输出1 print(np.max(b)) # 输出9
Summary:
This article introduces some common functions and operation methods in the NumPy library, and demonstrates their usage through specific code examples. By learning these functions and operations, you can better understand and apply the NumPy library for scientific computing and data analysis. I hope this article can help you learn NumPy!
The above is the detailed content of A concise guide to using numpy functions. For more information, please follow other related articles on the PHP Chinese website!
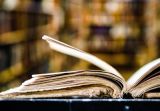
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
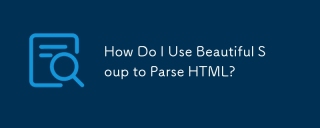
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
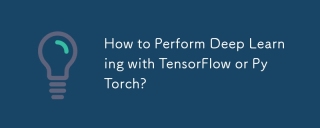
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
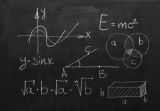
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
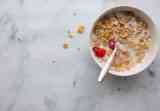
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
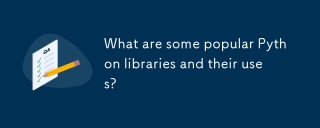
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
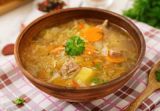
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
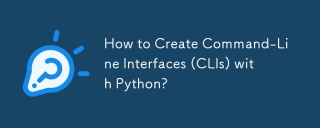
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
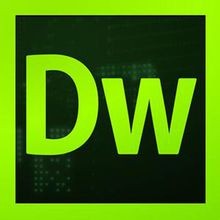
Dreamweaver CS6
Visual web development tools
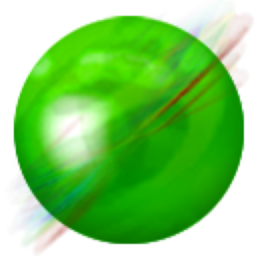
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
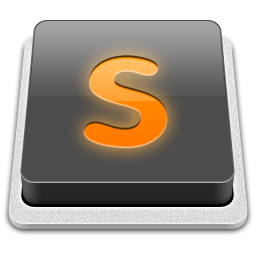
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
