


Avoid pitfalls: Precautions and common mistakes in mastering Golang pointers
Precautions and common mistakes of Golang pointers to help you avoid pitfalls
In Golang, a pointer is a special data type that stores a variable the address of. Through pointers, we can directly access and modify the value stored at the corresponding address. Using pointers can improve program efficiency and flexibility, but it is also prone to errors. This article will introduce the precautions for Golang pointers and help you avoid possible pitfalls through specific code examples.
- Don’t return pointers to local variables
When we want to return a pointer, we must be careful not to return pointers to local variables. Because the local variable's memory is released when the function ends, returning its pointer will result in an invalid pointer reference.
Sample code:
func createPointer() *int { i := 10 return &i // 错误!返回了局部变量的指针 } func main() { p := createPointer() fmt.Println(*p) // 会导致编译错误或者运行时错误 }
The correct approach is to use the new
keyword or use the make
function to create a pointer.
func createPointer() *int { i := 10 return new(int) // 创建一个新的指针 } func main() { p := createPointer() *p = 10 fmt.Println(*p) // 输出 10 }
- Don’t dereference nil pointers
In Golang, a nil pointer is a null pointer, that is, a pointer that points to no valid address. When we dereference a nil pointer, it causes a runtime error.
Sample code:
func main() { var p *int fmt.Println(*p) // 运行时错误 }
In order to avoid dereferencing a nil pointer, we can ensure that the pointer is valid by determining whether it is nil before using it.
func main() { var p *int if p != nil { fmt.Println(*p) } else { fmt.Println("p is nil") } }
- Pay attention to the type comparison of pointers
In Golang, the type of pointers also needs to be paid attention to. Pointers of different types cannot be compared directly.
Sample code:
func main() { var p1 *int var p2 *int if p1 == p2 { // 编译错误 fmt.Println("p1 和 p2 相等") } }
In order to compare the values of pointers, we need to convert the pointers to the same type.
func main() { var p1 *int var p2 *int if p1 == (*int)(unsafe.Pointer(p2)) { fmt.Println("p1 和 p2 相等") } }
- Avoid null references of pointers
When using pointers, be careful to avoid null references of pointers, that is, pointers do not point to valid memory addresses. If you use a null-referenced pointer for dereference or assignment, a runtime error will result.
Sample code:
func main() { var p *int *p = 10 // 运行时错误 }
In order to avoid the null reference of the pointer, we can use the new
keyword or the make
function to create the pointer and assign the corresponding memory space.
func main() { p := new(int) *p = 10 // 指针指向了有效的内存地址 fmt.Println(*p) // 输出 10 }
- Pay attention to the passing of pointers
In Golang, when calling a function, parameters are passed by value. If the argument type is a pointer, a copy of the pointer is passed, not the pointer itself. This means that modifications to the pointer within the function will not affect the original pointer.
Sample code:
func modifyPointer(p *int) { i := 10 p = &i } func main() { var p *int modifyPointer(p) fmt.Println(p) // 输出空指针 nil }
In order to modify the value of a pointer inside a function, we need to pass a pointer to the pointer or use a reference to the pointer.
func modifyPointer(p *int) { i := 10 *p = i } func main() { var p *int modifyPointer(&p) fmt.Println(*p) // 输出 10 }
Through the above precautions and sample code, I hope it can help you understand the use of Golang pointers and avoid common mistakes. When you use pointers correctly, they become a powerful tool in your programming, improving the efficiency and flexibility of your program.
The above is the detailed content of Avoid pitfalls: Precautions and common mistakes in mastering Golang pointers. For more information, please follow other related articles on the PHP Chinese website!
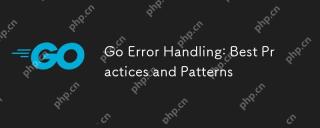
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
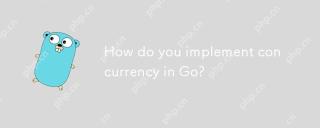
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
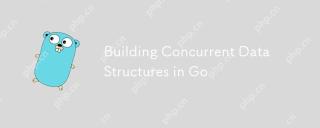
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
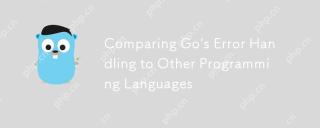
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand
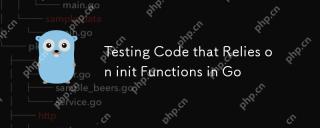
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
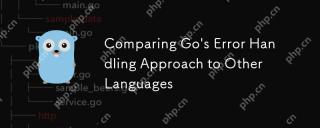
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
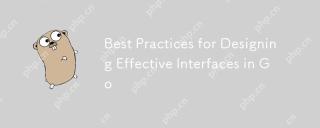
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
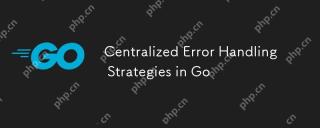
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
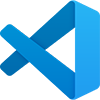
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
