


These provided Golang frameworks will double your development efficiency
Golang framework recommendation: These frameworks will make your development more efficient
Introduction:
With the popularity of Go language, more and more developers are beginning to choose Go as its own development language. When developing Go language, it is very important to choose a suitable framework, which can effectively improve development efficiency and code quality. In this article, I will introduce several Golang frameworks that I personally recommend and provide specific code examples to help readers understand and use these frameworks more deeply.
- Gin Framework
Gin is a lightweight and fast Web framework that takes advantage of the high-performance features of the Go language and is suitable for building high-performance Web applications. Gin has a concise and clear API design and good middleware support, allowing you to quickly develop robust Web services.
Here is a sample code that shows how to use the Gin framework to create a simple HTTP server and handle GET requests:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/hello", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, Gin!", }) }) r.Run() }
- Go-kit framework
Go -Kit is a powerful microservices toolkit that provides a complete set of tools and libraries for building and deploying scalable, reliable distributed systems. Go-kit has a good modular design and can easily expand and integrate various services.
The following is a sample code that demonstrates how to use the Go-kit framework to create a simple microservice and corresponding client:
package main import ( "context" "errors" "fmt" "net/http" "github.com/go-kit/kit/endpoint" "github.com/go-kit/kit/transport/http" ) func main() { greetingEndpoint := makeGreetingEndpoint() server := http.NewServer(makeHTTPHandler(greetingEndpoint)) http.Handle("/greeting", server) err := http.ListenAndServe(":8080", nil) if err != nil { fmt.Println(err) } } func makeGreetingEndpoint() endpoint.Endpoint { return func(_ context.Context, request interface{}) (interface{}, error) { req := request.(string) if req == "" { return nil, errors.New("empty request") } return fmt.Sprintf("Hello, %s!", req), nil } } func makeHTTPHandler(e endpoint.Endpoint) http.Handler { return http.NewServer( e, func(_ context.Context, r *http.Request) (interface{}, error) { return r.URL.Query().Get("name"), nil }, func(_ context.Context, w http.ResponseWriter, response interface{}) error { return http.EncodeJSONResponse(context.Background(), w, response) }, ) }
- Beego Framework
Beego is a powerful full-stack web framework with rich functions and flexible plug-in mechanism. Beego provides routing, models, ORM, middleware and other functions to easily create complex web applications.
The following is a sample code that shows how to use the Beego framework to create a simple web application and handle GET requests:
package main import ( "github.com/astaxie/beego" ) type HelloController struct { beego.Controller } func (c *HelloController) Get() { c.Data["json"] = map[string]interface{}{ "message": "Hello, Beego!", } c.ServeJSON() } func main() { beego.Router("/hello", &HelloController{}) beego.Run() }
Conclusion:
The above introduces a few I personally recommend the Golang framework. Each framework has its own characteristics and advantages. Choosing the right framework can help improve development efficiency and code quality, and matching project needs is more important. We hope that the introduction and sample code of this article can help readers better understand and apply these frameworks, so that your development can get twice the result with half the effort!
The above is the detailed content of These provided Golang frameworks will double your development efficiency. For more information, please follow other related articles on the PHP Chinese website!
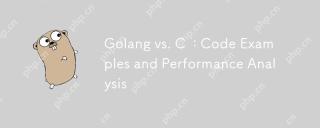
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
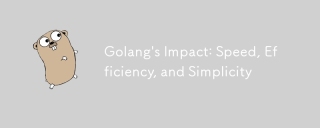
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
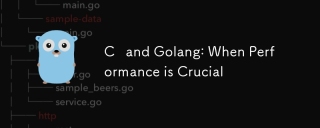
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
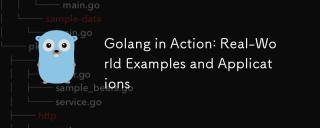
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
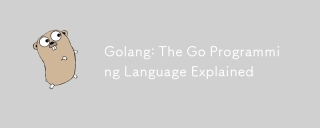
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
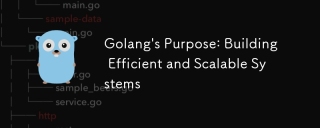
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
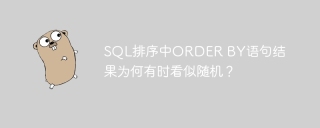
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
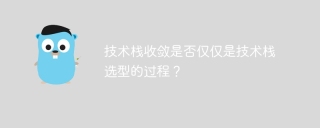
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
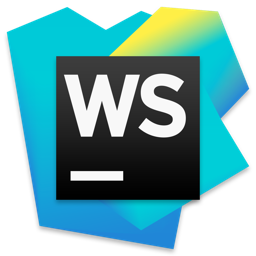
WebStorm Mac version
Useful JavaScript development tools
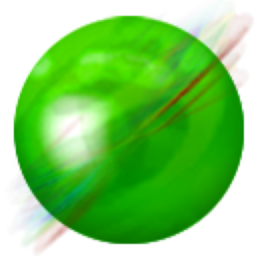
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment