Common data structures and usage in Golang standard library
Commonly used data structures and applications in the Golang standard library
Introduction:
Golang is a concise and efficient programming language, and its standard library contains various Commonly used data structures, such as arrays, slices, maps, stacks, etc. This article will introduce commonly used data structures and their applications in the Golang standard library, and provide corresponding code examples.
1. Array:
An array is a fixed-size data structure that stores a series of elements of the same type. Arrays in Golang are defined as follows:
var arrayName [size]dataType
Among them, arrayName is the name of the array, size is the size of the array, and dataType is the data type of the array elements. The following is an example:
var numbers [5]int
We can access the elements of the array through subscripts. The subscripts start from 0. The following is an example:
numbers[0] = 10
With the above code, we access the elements of the array numbers The first element is assigned a value of 10.
2. Slice:
A slice is a variable-length data structure that is built around the concept of dynamic arrays. Slices can automatically expand and provide convenient operation methods. Slices in Golang are defined as follows:
var sliceName []dataType
Among them, sliceName is the name of the slice, and dataType is the data type of the slice element. Here is an example:
var fruits []string
We can create a slice using the built-in make function as shown below:
numbers := make([]int, 5)
With the above code, we create a slice numbers with length 5 and elements The type is int.
Slices support accessing and modifying elements by index, the following is an example:
numbers[0] = 10
We can also use the append function to add elements to the slice, as follows:
numbers = append(numbers, 20)
3. Map:
Map is an unordered collection of key-value pairs, in which each element consists of a unique key and a corresponding value. The mapping in Golang is defined as follows:
var mapName map[keyType]valueType
Among them, mapName is the name of the mapping, keyType is the data type of the key, and valueType is the data type of the value. The following is an example:
var fruits map[string]int
We can use the make function to create a map as follows:
numbers := make(map[string]int)
With the above code, we create a map numbers where the data type of the key is string , the data type of the value is int.
We can use keys to access values in the map. The following is an example:
numbers["apple"] = 3
With the above code, we set the value corresponding to the key "apple" to 3.
4. Stack:
The stack is a special data structure in which elements are operated in last-in-first-out (LIFO) order. There is no built-in stack data structure in Golang, but we can simulate the behavior of the stack through slicing. The following is an example:
type Stack []int func (s *Stack) Push(value int) { *s = append(*s, value) } func (s *Stack) Pop() (int, error) { if len(*s) == 0 { return 0, errors.New("stack is empty") } index := len(*s) - 1 value := (*s)[index] *s = (*s)[:index] return value, nil }
Through the above code, we define a slice of Stack type and implement the Push and Pop methods to simulate the push and pop operations of the stack.
5. Summary:
This article introduces common data structures and their applications in the Golang standard library, including arrays, slices, maps and stacks. By understanding and using these data structures, we can program more efficiently and solve various practical problems.
The above is an introduction to common data structures and applications in the Golang standard library. I hope it will be helpful to readers. If you want to learn more about the detailed implementation and more usage methods of these data structures, it is recommended to refer to Golang official documentation and other related resources.
The above is the detailed content of Common data structures and usage in Golang standard library. For more information, please follow other related articles on the PHP Chinese website!
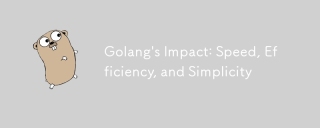
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
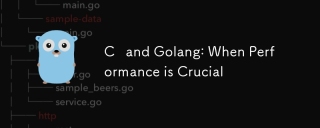
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
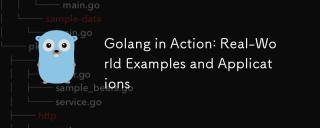
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
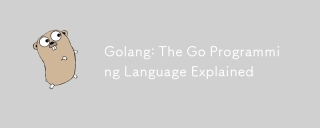
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
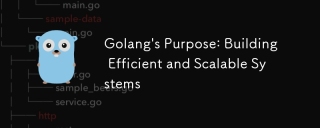
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
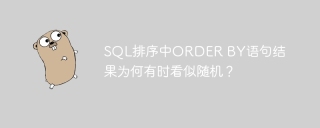
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
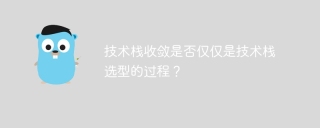
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
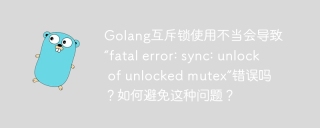
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
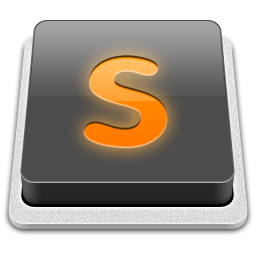
SublimeText3 Mac version
God-level code editing software (SublimeText3)
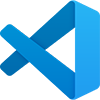
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft