


PHP backend design: exploration of security and permission control
With the popularity of the Internet and Web applications, PHP has become one of the most popular backend languages. However, the development of PHP backend involves very important security and permission control issues.
In this article, we will explore security and permission control in PHP backend design, and provide specific code examples to help readers better understand these issues.
1. Security Issues
When it comes to PHP security issues, it mainly involves the following aspects:
- SQL injection attack
SQL injection is an attack method that exploits vulnerabilities in web applications to manipulate or view data by manipulating the input of SQL queries. To avoid SQL injection attacks, we need to protect ourselves while writing code.
The following is a simple SQL injection example:
$username = $_POST['username']; $sql = "SELECT * FROM user WHERE username='$username'";
In this example, the attacker can bypass the user by entering ' or 1=1 --
Enter the content and obtain the data of the entire user table. To prevent this from happening, we need to use prepared statements in PHP.
The modified sample code is as follows:
$username = $_POST['username']; $stmt = $pdo->prepare("SELECT * FROM user WHERE username=?"); $stmt->execute([$username]); $user = $stmt->fetch();
In this example, we use the preprocessing statement in PDO to separate the user input content from the SQL statement. This can effectively avoid SQL injection attacks.
- XSS attack
XSS attack is a technique that exploits vulnerabilities in web applications and allows an attacker to inject HTML tags or JavaScript code into the user's browser. In order to avoid XSS attacks, we need to use the htmlspecialchars() function to filter user input.
The following is a simple XSS attack example:
echo "Welcome, " . $_GET['username'] . "!";
The attacker can pass a JavaScript code as the username parameter, for example: http://localhost/welcome.php?username =<script>alert("XSS!")</script>
, so that an alert box can pop up in the user's browser.
In order to avoid this situation, we need to use the htmlspecialchars() function to filter the content entered by the user. The modified code is as follows:
echo "Welcome, " . htmlspecialchars($_GET['username'], ENT_QUOTES, 'UTF-8') . "!";
In this example, we use the htmlspecialchars() function to filter user input, so that XSS attacks can be avoided.
- CSRF attack
A CSRF attack is a type of vulnerability that exploits web applications. An attacker can construct a page or URL that allows users to execute without their knowledge. certain operations. To avoid CSRF attacks, we need to use CSRF tokens or the same origin policy.
The following is a simple CSRF attack example:
<!-- 在攻击者的网站上 --> <img src="/static/imghwm/default1.png" data-src="http://localhost/delete.php?id=1?x-oss-process=image/resize,p_40" class="lazy" alt="PHP backend design: exploration of security and permission control" >
The attacker allows the user to access this page by sending an email to the user or publishing a blog post. This page will delete the record with id 1 without the user's knowledge.
To avoid this happening, we need to use CSRF tokens or same-origin policy. The sample code is as follows:
<!-- 在表单中添加CSRF令牌 --> <form action="delete.php" method="POST"> <input type="hidden" name="token" value="<?php echo md5(session_id()); ?>"> <input type="hidden" name="id" value="1"> <button type="submit" class="btn btn-danger">删除</button> </form>
In this example, we use a CSRF token to prevent malicious attackers from constructing pages or URLs to attack our system.
2. Permission control issues
When it comes to PHP permission control issues, it mainly involves the following aspects:
- Authentication
In order to ensure the security of the system, the user's identity must be authenticated. Authentication is required before handling sensitive operations.
The sample code is as follows:
if (! check_user_permission('admin')) { die("Permission denied!"); } // 进行敏感操作
In this example, we use the check_user_permission() function to check whether the user has permission to operate. If the user does not have permission, the operation is terminated.
- Role Control
Different users in the system may need different permissions and operation scopes. In order to achieve this kind of permission control, role control method is usually used.
The sample code is as follows:
// 用户与角色映射关系 $users = [ 'Alice' => ['admin'], 'Bob' => ['editor'], 'Charlie' => ['editor', 'viewer'], ]; // 检查当前用户的角色 function get_user_roles($username) { global $users; return $users[$username] ?? []; } // 检查用户是否有权限 function check_user_permission($username, $permission) { $roles = get_user_roles($username); foreach ($roles as $role) { if (isset($permissions[$role]) && $permissions[$role][$permission]) { return true; } } return false; } // 定义角色与权限映射关系 $permissions = [ 'admin' => ['create', 'update', 'delete'], 'editor' => ['create', 'update'], 'viewer' => ['view'], ]; // 检查用户是否有权限 if (!check_user_permission('Alice', 'delete')) { die("Permission denied!"); } // 进行敏感操作
In this example, we define the mapping relationship between roles and permissions, and use the check_user_permission() function to check whether the user has permission to operate. If the user does not have permission, the operation is terminated.
The above is some discussion of security and permission control issues in PHP backend design. We recommend that developers enhance their learning and understanding of these issues during the actual development process, and adhere to the best practices for security and permission control when writing code.
If you have any questions or need further assistance, please feel free to contact us.
The above is the detailed content of PHP backend design: exploration of security and permission control. For more information, please follow other related articles on the PHP Chinese website!
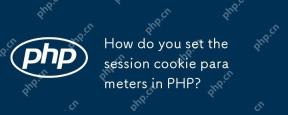
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
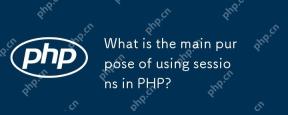
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
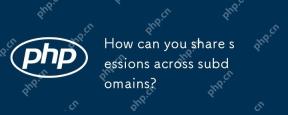
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
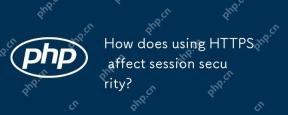
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
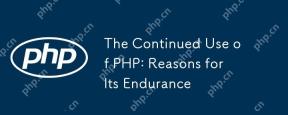
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
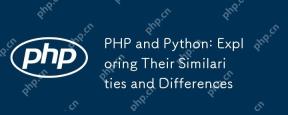
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
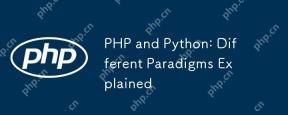
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
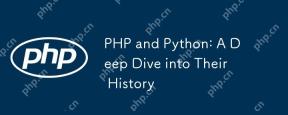
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.