


Full analysis of common Golang libraries: Make your project more competitive
Introduction:
Golang is a concise and efficient programming language because of its It is favored by developers for its excellent concurrency performance and lightweight development style. However, as a relatively young language, Golang still lacks in standard libraries. Fortunately, there are many excellent third-party libraries that can make your project more competitive. This article will comprehensively introduce some commonly used Golang libraries, including their functions and specific code examples.
1. Network programming related libraries
- Gin: Gin is a lightweight Web framework that provides fast routing and middleware support and is suitable for building high-performance Web Serve. Here is a basic Gin sample code:
package main import "github.com/gin-gonic/gin" func main() { router := gin.Default() router.GET("/", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, World!", }) }) router.Run() }
- Echo: Echo is another popular web framework that, like Gin, provides fast routing and middleware support. The following is a sample code for using Echo to build a RESTful API:
package main import ( "github.com/labstack/echo" "net/http" ) type User struct { Name string `json:"name"` Email string `json:"email"` } func main() { e := echo.New() e.GET("/", func(c echo.Context) error { return c.String(http.StatusOK, "Hello, World!") }) e.POST("/users", func(c echo.Context) error { u := new(User) if err := c.Bind(u); err != nil { return err } return c.JSON(http.StatusOK, u) }) e.Start(":8080") }
2. Database related library
- Gorm: Gorm is a simple and powerful ORM library that provides It has a concise API and flexible query function. The following is a sample code for using Gorm to connect to a MySQL database:
package main import ( "gorm.io/driver/mysql" "gorm.io/gorm" ) type User struct { ID uint Name string } func main() { dsn := "your-dsn" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic(err) } db.AutoMigrate(&User{}) user := User{Name: "Alice"} db.Create(&user) var users []User db.Find(&users) for _, u := range users { println(u.Name) } db.Delete(&user) }
- Redis: Redis is a high-performance key-value storage database, often used for caches, queues, etc. The following is a sample code using Redis for caching:
package main import ( "github.com/go-redis/redis/v8" "context" ) func main() { ctx := context.Background() rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) err := rdb.Set(ctx, "key", "value", 0).Err() if err != nil { panic(err) } val, err := rdb.Get(ctx, "key").Result() if err != nil { panic(err) } println(val) }
3. Concurrent programming related libraries
- Goroutine pool: Goroutine pool is a library for managing and reusing Goroutine , can effectively control concurrent resources. The following is a sample code using the Grpool library:
package main import ( "github.com/ivpusic/grpool" "time" ) func main() { pool := grpool.NewPool(10, 100) for i := 0; i < 1000; i++ { pool.JobQueue <- func() { time.Sleep(1 * time.Second) println("Task complete!") } } pool.WaitAll() pool.Release() }
- Channel wrapper: Channel wrapper is a tool that encapsulates common operations and can simplify communication operations in concurrent programming. The following is a sample code using the Chaos library for messaging:
package main import ( "github.com/go-chaos/chaos" ) type Message struct { Content string } func main() { c := chaos.New() c.Start() in := c.In() out := c.Out() go func() { for i := 0; i < 10; i++ { in <- &Message{Content: "Hello, Chaos!"} } }() go func() { for { msg := <-out println(msg.(*Message).Content) } }() c.Stop() }
Conclusion:
The above is just a brief introduction to commonly used Golang libraries. There are many other excellent libraries that can further supplement your project. As a Golang developer, understanding how to use these common libraries can make your project more competitive and improve development efficiency. Hope this article is helpful to you!
The above is the detailed content of In-depth analysis of common Golang libraries: Improve your project competitiveness. For more information, please follow other related articles on the PHP Chinese website!
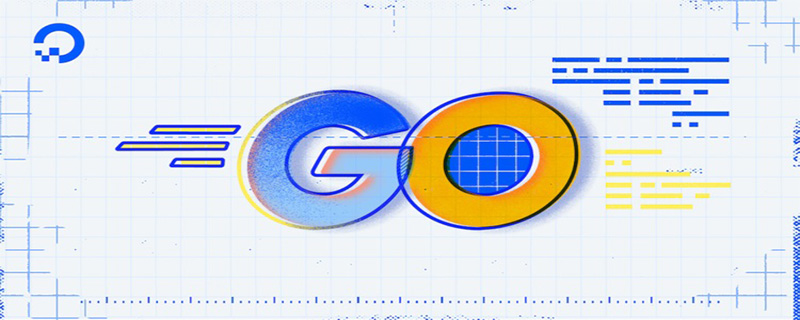
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
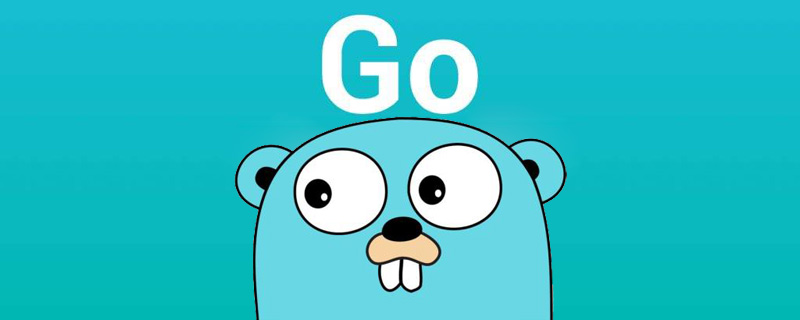
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
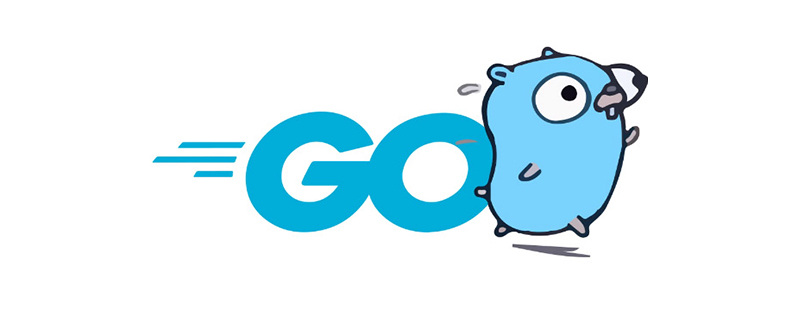
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
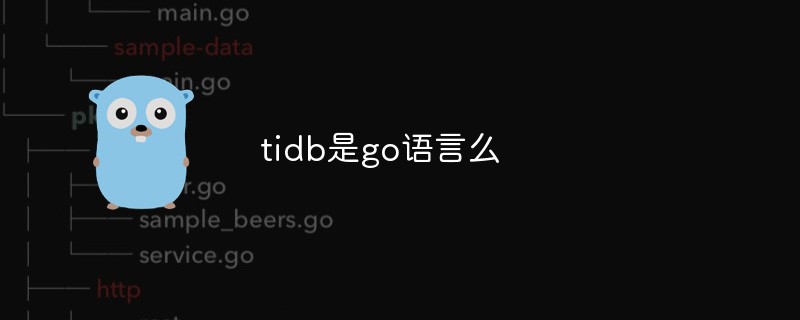
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
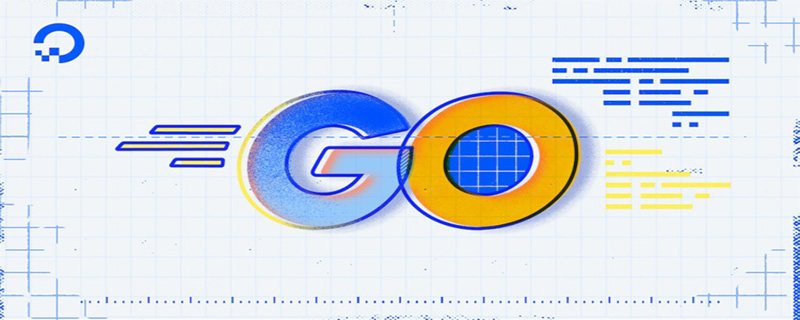
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
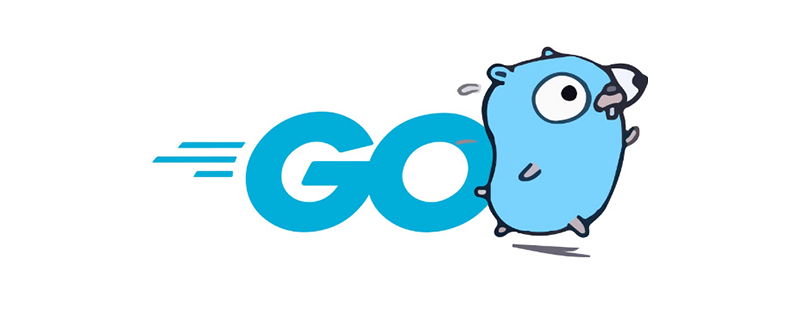
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
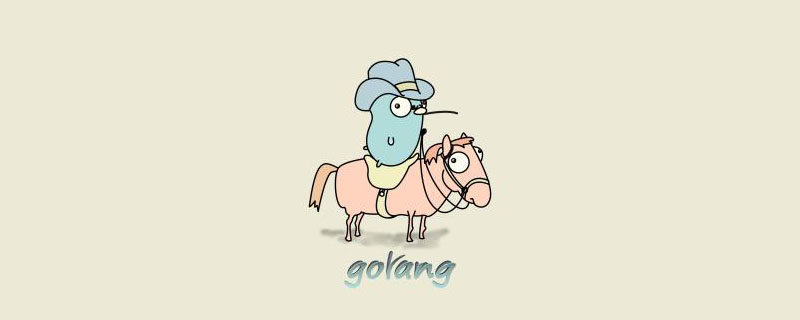
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
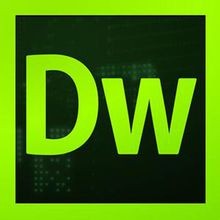
Dreamweaver CS6
Visual web development tools
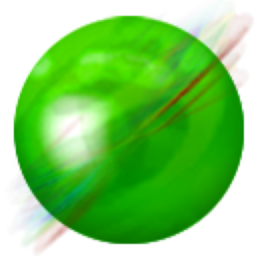
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
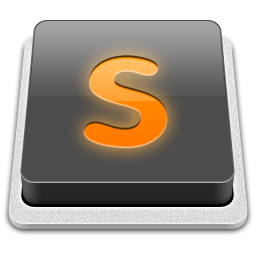
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
