


Learn data structures in Go language: from basic to advanced, specific code examples are required
[Introduction]
In the process of learning any programming language, It is very important to master the data structure. Data structure is the foundation of computer science. It provides us with methods to store and organize data so that we can process and operate data more efficiently. As a modern programming language, Go language also provides rich data structures and related operation methods. This article will provide an in-depth introduction to the data structures in the Go language to help readers understand and master the basic knowledge of data structures. It will also provide specific code examples to help readers actually use these data structures.
[1. Array]
Array is the simplest data structure, which can store a group of elements of the same type. In the Go language, we can define and use arrays in the following ways:
var arr [5]int // 定义一个长度为5的整型数组 arr[0] = 1 // 给数组的第一个元素赋值为1 fmt.Println(arr) // 输出整个数组
[2. Slice]
A slice is an encapsulated data structure based on an array, and it has a more flexible length. and capacity. In the Go language, slices can be defined and used in the following ways:
var slice []int // 定义一个切片 slice = append(slice, 1) // 往切片中添加元素1 fmt.Println(slice) // 输出整个切片
[3. Linked list]
The linked list is a dynamic data structure that connects a set of discontinuous memory blocks through pointers. together. In the Go language, linked lists can be implemented through custom structures and pointers:
type Node struct { value int next *Node } var head *Node // 定义链表的头指针 head = &Node{value: 1} // 创建一个节点并赋值为1 head.next = &Node{value: 2} // 创建一个节点并赋值为2,并将其指针赋给上一个节点的next fmt.Println(head.value) // 输出链表的第一个节点的值 fmt.Println(head.next.value) // 输出链表的第二个节点的值
[4. Stack]
The stack is a last-in-first-out (LIFO) data structure, similar to our daily A stack of dishes in life. In the Go language, you can use slices to implement the stack function:
var stack []int // 定义一个切片作为栈 stack = append(stack, 1) // 入栈 fmt.Println(stack[len(stack)-1]) // 输出栈顶元素 stack = stack[:len(stack)-1] // 出栈
[5. Queue]
The queue is a first-in-first-out (FIFO) data structure, similar to queuing in our daily life Waiting scene. In Go language, you can use slices to implement queue functions:
var queue []int // 定义一个切片作为队列 queue = append(queue, 1) // 入队 fmt.Println(queue[0]) // 输出队首元素 queue = queue[1:] // 出队
[6. Hash table]
A hash table is a data structure that uses a hash function to map keys to values. Search and insert operations can be performed quickly. In the Go language, you can use the map type to implement the function of a hash table:
var dict map[string]int // 定义一个map作为哈希表 dict = make(map[string]int) // 初始化哈希表 dict["one"] = 1 // 插入键值对 fmt.Println(dict["one"]) // 输出键对应的值 delete(dict, "one") // 删除键值对
[7. Tree]
The tree is a non-linear data structure with the characteristics of hierarchical structure and recursive definition. . In the Go language, structures and pointers can be used to implement tree functions:
type TreeNode struct { value int left *TreeNode right *TreeNode } var root *TreeNode // 定义树的根节点 root = &TreeNode{value: 1} // 创建一个节点并赋值为1 root.left = &TreeNode{value: 2} // 创建一个节点并赋值为2,并将其指针赋给父节点的left root.right = &TreeNode{value: 3} // 创建一个节点并赋值为3,并将其指针赋给父节点的right fmt.Println(root.value) // 输出根节点的值 fmt.Println(root.left.value) // 输出左子节点的值 fmt.Println(root.right.value) // 输出右子节点的值
[8. Figure]
The graph is a non-linear data structure consisting of nodes and edges. The relationship can be arbitrary. In the Go language, structures and slices can be used to implement graph functions:
type Graph struct { nodes []string edges [][]int } var g Graph // 定义一个图的结构体 g.nodes = []string{"A", "B", "C", "D"} // 定义节点集合 g.edges = [][]int{{0, 1}, {1, 2}, {2, 3}} // 定义边的集合 fmt.Println(g.nodes[0]) // 输出第一个节点 fmt.Println(g.edges[0][1]) // 输出第一条边的终止节点
[Conclusion]
Through the introduction of this article, we have learned about the commonly used data structures in the Go language and their basic operations. method. Data structures are an important foundation in programming, and proficiency in data structures is crucial to writing efficient and reliable code. Through learning and practice, we can better utilize data structures to solve practical problems and write more elegant and efficient code. I hope this article can provide some help to readers in learning data structures in Go language.
The above is the detailed content of A comprehensive guide to mastering Go language data structures: from entry to mastery. For more information, please follow other related articles on the PHP Chinese website!
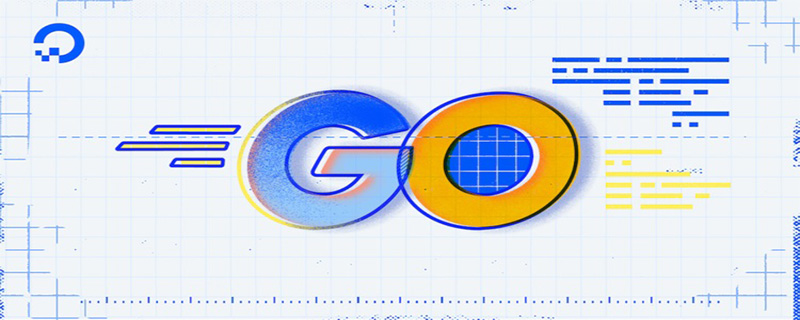
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
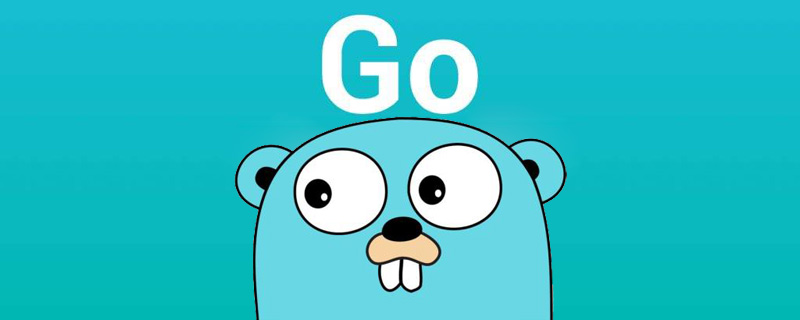
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
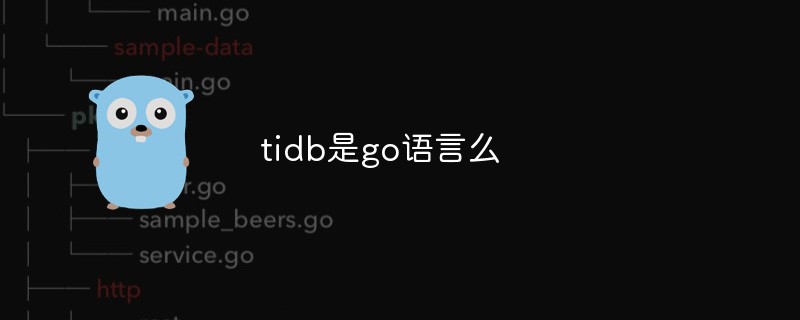
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
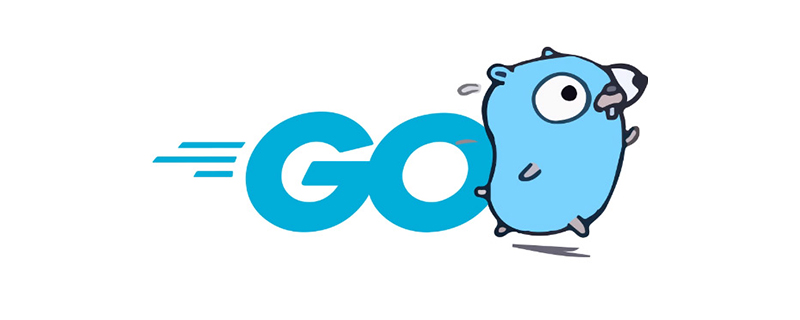
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
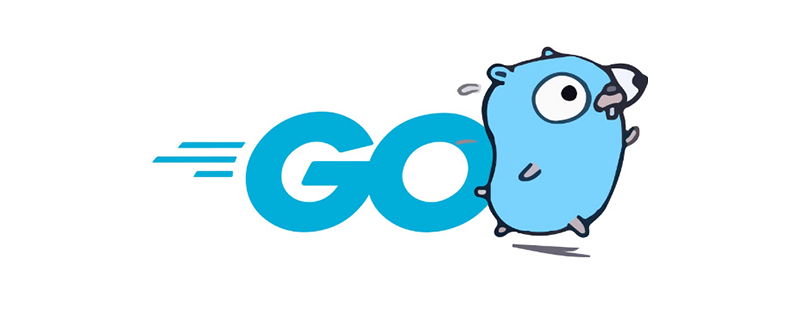
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
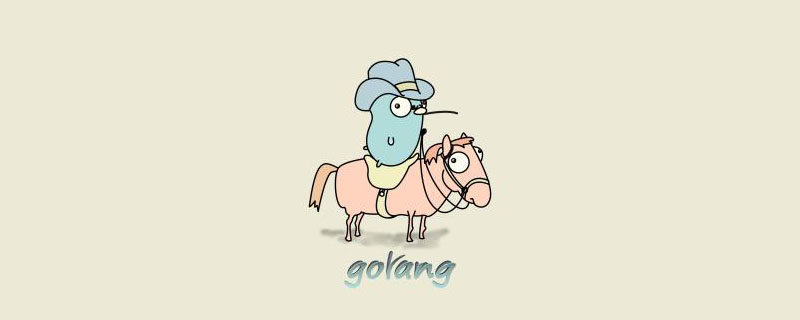
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
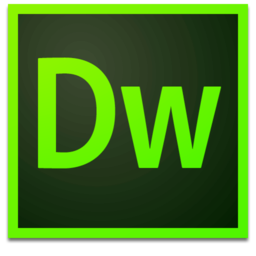
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
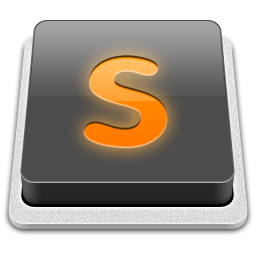
SublimeText3 Mac version
God-level code editing software (SublimeText3)
