Security issues and solutions for Java Queue in multi-threaded environment
Safety issues and solutions for Java Queue queues in multi-threaded environments
Introduction:
In multi-threaded programming, shared resources in the program may Facing race conditions, this can lead to data inconsistencies or errors. In Java, Queue is a commonly used data structure. When multiple threads operate the queue at the same time, there are security issues. This article will discuss the security issues of Java Queue in a multi-threaded environment and introduce several solutions, focusing on explanations in the form of code examples.
1. Security issues in multi-threaded environments
- Data competition issues:
When multiple threads perform push and pop operations on the queue at the same time, if there is no correct synchronization mechanism, race conditions may occur. For example, one thread is performing a pop operation while another thread is performing a push operation at the same time, which may result in the loss or duplication of data in the queue. - Concurrent modification problem:
When multiple threads modify the queue at the same time, the status of the queue may be inconsistent. For example, one thread is performing a delete operation while another thread performs an insertion operation at the same time, which may cause the inserted element to be deleted.
2. Solution to security issues
- Use the synchronized keyword synchronized:
Using the synchronized keyword can ensure that only one thread can access the code at the same time block to prevent multiple threads from operating on the queue at the same time. The following is a sample code that uses the synchronized keyword to solve queue security issues:
import java.util.Queue; public class SynchronizedQueueExample { private Queue<Integer> queue; // 假设这是一个队列 public synchronized void push(int num) { queue.add(num); } public synchronized int pop() { return queue.remove(); } }
Using the synchronized keyword can ensure that push and pop operations are synchronized, ensuring that only one thread operates at a time.
- Use ReentrantLock:
ReentrantLock is a reentrant lock in Java that can be used to more flexibly control multiple threads' access to the queue. The following is a sample code that uses ReentrantLock locks to solve queue security issues:
import java.util.Queue; import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; public class ReentrantLockQueueExample { private Queue<Integer> queue; // 假设这是一个队列 private Lock lock = new ReentrantLock(); public void push(int num) { lock.lock(); try { queue.add(num); } finally { lock.unlock(); } } public int pop() { lock.lock(); try { return queue.remove(); } finally { lock.unlock(); } } }
Using ReentrantLock locks can more flexibly control the timing of lock acquisition and release, thereby reducing the occurrence of race conditions.
- Using ConcurrentLinkedQueue:
ConcurrentLinkedQueue is a concurrent safe queue in Java, multiple threads can operate on it at the same time without additional synchronization mechanism. The following is a sample code that uses ConcurrentLinkedQueue to solve queue security issues:
import java.util.Queue; import java.util.concurrent.ConcurrentLinkedQueue; public class ConcurrentLinkedQueueExample { private Queue<Integer> queue = new ConcurrentLinkedQueue<>(); public void push(int num) { queue.add(num); } public int pop() { return queue.remove(); } }
Using ConcurrentLinkedQueue can avoid explicit synchronization mechanisms and provide better performance.
Conclusion:
In multi-threaded programming, Java Queue may have security issues in a multi-threaded environment. This article introduces three ways to use the synchronized keyword, ReentrantLock and ConcurrentLinkedQueue to solve queue security issues, and gives corresponding code examples. In actual development, it is very important to choose the appropriate solution to ensure the security of the queue based on specific needs and scenarios.
The above is the detailed content of Security issues and solutions for Java Queue in multi-threaded environment. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
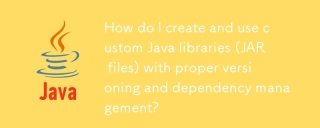
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
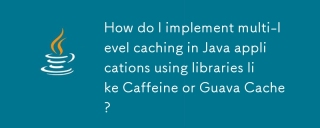
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
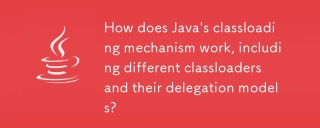
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
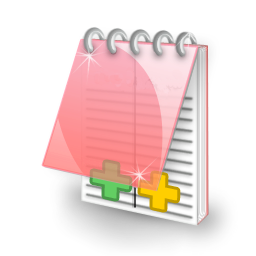
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
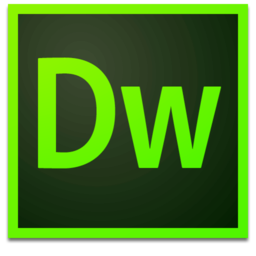
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor