


Analysis of closure technology: Master this knowledge to make your code more flexible and scalable. Specific code examples are required
Introduction:
In programming In the world, closure is a very powerful and flexible concept. By using closure techniques, you can make your code more resilient and scalable. This article will delve into what closures are, how they work, and how to apply closure techniques in practice. We will use specific code examples to help readers better understand and apply closures.
Part 1: The Concept and Working Principle of Closure
Closure means that a function can access variables defined in its external function and can continue to use these variables after the execution of its external function ends. In other words, a closure creates an independent environment that contains all the variables in the scope in which the function is defined. These variables are private to the function and cannot be accessed by other functions.
Closures work by binding a function to its environment (that is, the scope in which the function is defined). When a function is called, it creates a new execution context and saves the environment information required by the function. When the function is executed, the execution context will be saved forever. This is how closures are implemented.
Part 2: Application Scenarios of Closures
Closures are widely used in many programming languages. It can be used to implement many useful functions, including but not limited to:
- Data hiding and encapsulation: Closures can privatize variables and only access and modify these variables through specific functions. This protects the security of the data while allowing external functions to access the variables.
- Delayed execution: Use closures to implement some functions that require delayed execution, such as executing a function under specific conditions.
- Event processing: Closures can be used to process events. Passing the event processing function as a closure to the event listener allows the event processing function to access the required variables.
Part 3: Specific code examples
Below we will use some specific code examples to illustrate the application of closures.
- Implement private variables:
function createCounter() { let count = 0; return { increment: function() { count++; }, decrement: function() { count--; }, getCount: function() { return count; } }; } let counter = createCounter(); console.log(counter.getCount()); // Output: 0 counter.increment(); console.log(counter.getCount()); // Output: 1
- Implement delayed execution:
function delayExecute(func, delay) { setTimeout(func, delay); } function sayHello() { console.log("Hello World"); } delayExecute(sayHello, 3000); // Output after 3 seconds: Hello World
- Implement event processing:
function createButton() { let count = 0; let button = document.createElement("button"); button.innerHTML = "Click Me"; button.addEventListener("click", function() { count++; console.log("Button was clicked " + count + " times"); }); return button; } let myButton = createButton(); document.body.appendChild(myButton);
Conclusion:
Closures are a powerful technique that can make your code more resilient and scalable. By using closures, we can achieve functions such as data hiding and encapsulation, delayed execution, and event handling. Mastering the concept and working principle of closures and being good at applying them to actual programming work will improve your code quality and development efficiency. I hope the code examples in this article can help readers better understand and apply closure technology.
The above is the detailed content of An in-depth analysis of closure technology: master these principles to make your code more flexible and scalable. For more information, please follow other related articles on the PHP Chinese website!
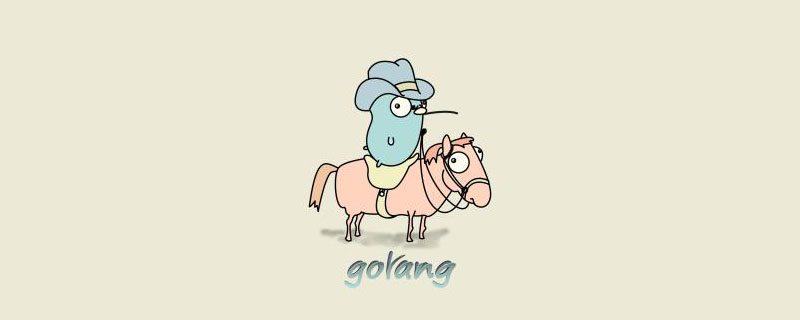
闭包(closure)是一个函数以及其捆绑的周边环境状态(lexical environment,词法环境)的引用的组合。 换而言之,闭包让开发者可以从内部函数访问外部函数的作用域。 闭包会随着函数的创建而被同时创建。
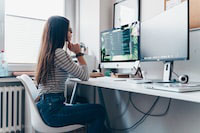
Java是开发分布式系统和微服务的流行编程语言。其丰富的生态系统和强大的并发功能提供了构建健壮、可扩展应用程序的基础。kubernetes是一种容器编排平台,用于管理和自动化容器化应用程序的部署、扩展和管理。它通过提供编排、服务发现和自动故障恢复等特性,简化了微服务环境的管理。Java和Kubernetes的优势:可扩展性:Kubernetes允许您轻松扩展应用程序,无论是在水平扩展还是垂直扩展方面。弹性:Kubernetes提供了自动故障恢复和自愈功能,确保应用程序在出现问题时保持可用。敏捷性
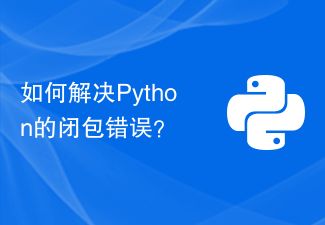
Python是一种非常流行的编程语言,因为它非常易学易用,同时也具备了强大的功能。其中,闭包是Python中的一种函数,它可以在函数的内部定义另一个函数,并返回这个函数作为函数的返回值。尽管闭包非常方便,但有时会出现某些错误,比如闭包错误。本文将介绍如何解决Python的闭包错误。初步了解闭包在Python中,闭包是由一个内部函数和一个定义在内部函数之外的函
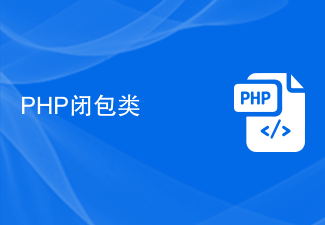
介绍匿名函数(也称为lambda)返回Closure类的对象。这个类有一些额外的方法,可以进一步控制匿名函数。语法Closure{ /*Methods*/ private__construct(void) publicstaticbind(Closure$closure,object$newthis[,mixed$newscope="static"
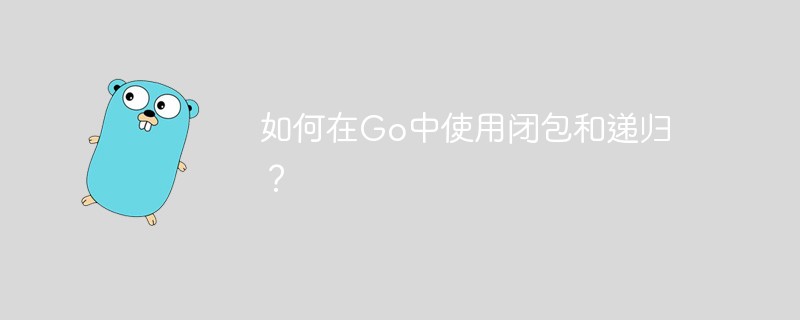
在Go程序设计中,闭包和递归是两个非常重要的概念。它们可以帮助我们更好地解决一些复杂问题,提高代码的可读性和可维护性。在本文中,我们将探讨如何在Go中使用闭包和递归。一、闭包闭包是指一个函数变量的值,它引用了函数体外部的变量。在Go中,我们可以使用匿名函数实现闭包的功能。以下是一个示例代码:funcmain(){user:="Al
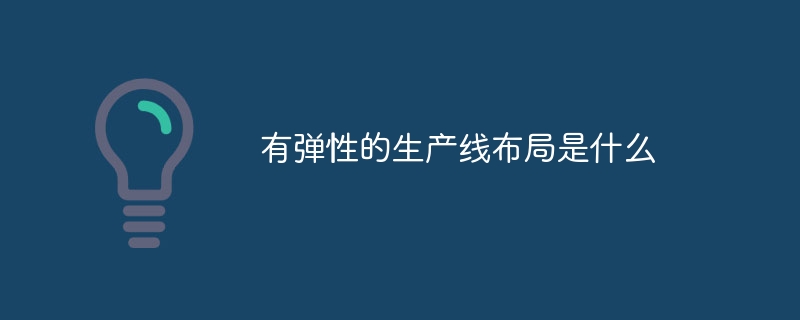
有弹性的生产线布局方式有U型生产线布局、环型生产线布局、生产线平衡布局、生产线流水线布局、生产线单元化布局等等。详细介绍:1、U型生产线布局是一种常见的生产线布局方式,可以提高生产效率和质量,在这种布局中,生产线呈U型排列,工人沿着生产线顺序完成各个生产环节;2、环型生产线布局是一种高效的生产线布局方式,可以提高生产效率和质量;3、生产线平衡布局等等。
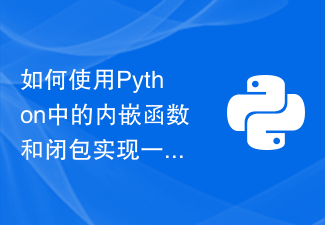
如何使用Python中的内嵌函数和闭包实现一个计数器Python作为一种功能强大的编程语言,提供了很多灵活的工具和技术,使得开发过程变得简单而高效。其中,内嵌函数和闭包是Python中非常重要的概念之一。在本文中,我们将探讨如何利用这两个概念实现一个简单的计数器。在Python中,内嵌函数指的是在一个函数内部定义的函数。内嵌函数可以访问外部函数的变量,并且具
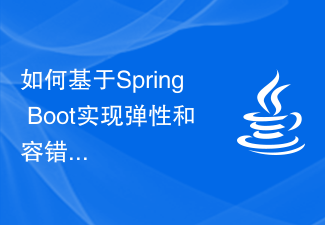
随着系统规模的不断扩大和业务要求的不断提高,软件系统的弹性和容错性成为了架构设计中至关重要的一环,一个具有高可用性、高性能、高效率的系统往往需要具有弹性和容错的设计模式。而SpringBoot作为一个快速开发和部署的Java框架,其丰富的生态系统以及框架本身基于微服务思想的设计让其成为了实现弹性和容错的理想选择。本文将介绍如何基于SpringBoot实现


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
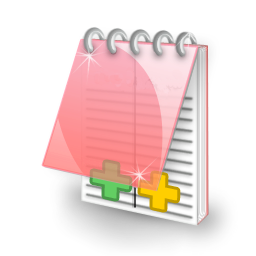
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
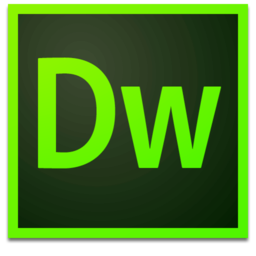
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
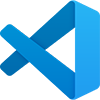
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
